Question
Can you help me with my code? It is only giving me one error saying my identifier: binary_search is unidentified, and I can't figure out
Can you help me with my code? It is only giving me one error saying my "identifier: binary_search is unidentified", and I can't figure out how to fix it.
I have also tried changing my #include's to: #include
#include
using namespace std;
template
public: int seqSearch(T searchItem); int binarySearch(T searchItem); void bubbleSort(); void insertionSort(); }; int main() { // Define test vector myVector
// Add values to the vector nameList.push_back("Alice"); nameList.push_back("Bob"); nameList.push_back("Eve"); nameList.push_back("Charlie"); nameList.push_back("Dave");
// Test sort methods nameList.bubbleSort(); nameList.insertionSort();
// Test search methods int index = nameList.seqSearch("Charlie"); cout << "Charlie was found at index: " << index << endl;
index = nameList.binarySearch("Charlie"); cout << "Charlie was found at index: " << index << endl;
// Print sorted vector using range based for loop cout << "Sorted vector: "; for (const auto& name : nameList) { cout << name << " "; } cout << endl;
// Define new test vector myVector
// Define an iterator to the vector auto it = intList.begin();
// Add values to the vector intList.insert(it, 1); intList.insert(it, 3); intList.insert(it, 2); intList.insert(it, 5); intList.insert(it, 4);
// Test the STL binary_search algorithm bool found = binary_search(intList.begin(), intList.end(), 3); if (found) { cout << "3 was found in the vector." << endl; } else { cout << "3 was not found in the vector." << endl; }
// Print the resulting vector using an iterator cout << "Sorted vector: "; for (it = intList.begin(); it != intList.end(); it++) { cout << *it << " "; } cout << endl;
return 0; }
template
template
template
template
int low = 0; int high = this->size() - 1; while (low <= high) { int mid = (low + high) / 2; if (this->at(mid) == searchItem) { return mid; // return index of found item } else if (this->at(mid) > searchItem) { high = mid - 1; } else { low = mid + 1; } } return -1; // return -1 if item not found }
int main() { // Define test vector myVector
// Add values to the vector nameList.push_back("Alice"); nameList.push_back("Bob"); nameList.push_back("Eve"); nameList.push_back("Charlie"); nameList.push_back("Dave"); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
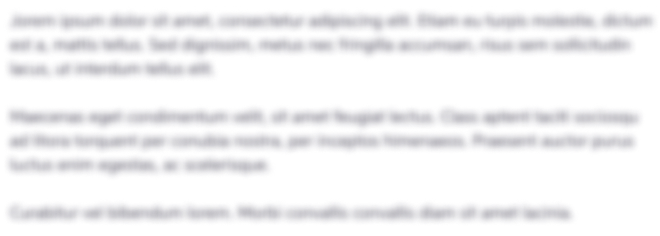
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started