Question
-Can you help with the percolation stats class.. we are getting errors when running the class.. i will attacth all the class files.. import edu.princeton.cs.algs4.StdRandom;
-Can you help with the percolation stats class.. we are getting errors when running the class.. i will attacth all the class files..
import edu.princeton.cs.algs4.StdRandom; import edu.princeton.cs.algs4.StdStats;
public class PercolationStats { private Percolation percolation; private double[] threshold; private double T; private int openSites; public PercolationStats(int N, int T) // perform T independent experiments on an NbyNgrid { if (N <= 0 || T <= 0) {
throw new IllegalArgumentException("Value is out of range");
} threshold = new double[T]; int randRow, randCol; this.T = T; for (int i = 0; i < T; i++) { percolation = new Percolation(N); randRow = StdRandom.uniform(1, N); randCol = StdRandom.uniform(1, N); percolation.open(randRow, randCol); openSites = 1; while (!percolation.percolates()) { randRow = StdRandom.uniform(1, N); randCol = StdRandom.uniform(1, N); if (!percolation.isOpen(randRow, randCol)) { percolation.open(randRow, randCol); openSites++; } } threshold[i] = ((double) openSites)/(N*N); } } public double mean() // sample mean of percolation threshold { return StdStats.mean(threshold); } public double stddev() // sample standard deviation of percolation threshold { return StdStats.stddev(threshold); } public double confidenceLow() // low endpoint of 95% confidence interval { return mean() - (1.96*stddev())/Math.sqrt(T); } public double confidenceHigh() // high endpoint of 95% confidence interval { return mean() + (1.96*stddev())/Math.sqrt(T); } public static void main(String[] args) { int T = 10; int N = 5; PercolationStats ps = new PercolationStats(N, T); //Percolation perc23 = new Percolation(N); //System.out.printf("PercolationStats(%d, %d)", N,T); } }
.....................................................................................
import edu.princeton.cs.algs4.WeightedQuickUnionUF;
public class Percolation { private boolean[][] grid; // grid used to tell if a site isOpen private WeightedQuickUnionUF weightedQUickUnionUF; private int[] flatgrid; // used for numeric values to correspond with each value of the grid private int top; // virtual top of the waterfall private int nSize; // input value used for the grid creation private int bottom; // virtual bottom of the waterfall private int openCount = 0; // this will be used to track open sites public Percolation(int N) // create NbyNgrid, with all sites blocked { if (N <= 0){ throw new IllegalArgumentException("Value is out of range"); } this.grid = new boolean[N][N]; // boolean grid this.flatgrid = new int[N * N]; // flatgrid to hold numbers for union joins this.nSize = N; // stores the N value to be used to determine the size of the grid's max value's in later methods top = (N * N) + 1; // top of percolation waterfall bottom = top - top; //bottom of percolation waterfall this.weightedQUickUnionUF = new WeightedQuickUnionUF((N * N) + 2); // creates weightedQUickUnion object //joins the top of the grid to the grid for waterfall effect for (int i = 0; i < N + 1; i ++) { weightedQUickUnionUF.union(i, top); //weightedQUickUnionUF.union((((N*N) +1) - i), bottom); } //adds numeric values to the flatgrid for (int i = 0; i < flatgrid.length; i++) { flatgrid[i]= i + 1; } } public void open(int i, int j) // open site (row i, column j) if it is not open already { if (!isOpen(i,j)){ openCount++; grid[i][j] = true; connectSquare(i, j); isFull(i, j); } //System.out.println(getFlatgridNum(i, j)); } public boolean isOpen(int i, int j) // is site (row i, column j) open? { return grid[i][j]; } public boolean isFull(int i, int j) // is site (row i, column j) full? { int q = getFlatgridNum(i, j); if (this.isOpen(i, j)) { return weightedQUickUnionUF.connected(q,this.top); } else { return false; } } public boolean percolates() // does the system percolate? { for (int i = 0; i < nSize -1; i++) { if (weightedQUickUnionUF.connected((nSize * nSize) - i, top)) { return true; } } return false; } public String numberOfOpenSites() { //number of open sites return String.format("%s", String.valueOf(openCount)); } //returns the int value for the grid spot based on the input row and column public int getFlatgridNum(int row, int col) { return flatgrid[(row * 10) + (col)]; } //this will check to see if a square is connected to a spot to the left, right, top, and bottom if the site is open. it will also check to make sure it does //not try and check or store a value in a position outside the index. public void connectSquare(int row, int col) { if (col > 0 && isOpen(row, col -1) && !(weightedQUickUnionUF.connected(getFlatgridNum(row, col),getFlatgridNum(row, col -1)))) //check -1 to the left { weightedQUickUnionUF.union(getFlatgridNum(row, col), getFlatgridNum(row, (col -1))); //System.out.printf("Col: %d Col -1: %d%n", col, col-1); } if (col < nSize - 1 && isOpen(row, col +1) && !(weightedQUickUnionUF.connected(getFlatgridNum(row, col),getFlatgridNum(row, col + 1)))) //check +1 to the right { weightedQUickUnionUF.union(getFlatgridNum(row, col), getFlatgridNum(row, col +1)); //System.out.printf("Col: %d Col -1: %d%n", col, col+1); } if (row < nSize -1 && isOpen(row +1, col) && !(weightedQUickUnionUF.connected(getFlatgridNum(row, col),getFlatgridNum(row + 1, col)))) //check going up will add + 1 { weightedQUickUnionUF.union(getFlatgridNum(row, col), getFlatgridNum(row + 1, col)); //System.out.printf("Row: %d Row +1: %d%n", row, row +1); } if (row > 0 && isOpen(row -1, col) && !(weightedQUickUnionUF.connected(getFlatgridNum(row, col),getFlatgridNum(row -1, col)))) //check going down will add - 1 { weightedQUickUnionUF.union(getFlatgridNum(row, col), getFlatgridNum(row - 1, col)); //System.out.printf("Row: %d Row -1: %d%n", row, row -1); } }
}
...................................................................................
/****************************************************************************** * Compilation: javac PercolationVisualizer.java * Execution: java PercolationVisualizer input.txt * Dependencies: Percolation.java * * This program takes the name of a file as a command-line argument. * From that file, it * * - Reads the grid size N of the percolation system. * - Creates an N-by-N grid of sites (intially all blocked) * - Reads in a sequence of sites (row i, column j) to open. * * After each site is opened, it draws full sites in light blue, * open sites (that aren't full) in white, and blocked sites in black, * with with site (0, 0) in the upper left-hand corner. * ******************************************************************************/
import java.awt.Font;
import edu.princeton.cs.algs4.In; import edu.princeton.cs.algs4.StdDraw;
public class PercolationVisualizer {
// delay in miliseconds (controls animation speed) private static final int DELAY = 100;
// draw N-by-N percolation system public static void draw(Percolation perc, int N) { StdDraw.clear(); StdDraw.setPenColor(StdDraw.BLACK); StdDraw.setXscale(-.05*N, 1.05*N); StdDraw.setYscale(-.05*N, 1.05*N); // leave a border to write text StdDraw.filledSquare(N/2.0, N/2.0, N/2.0);
// draw N-by-N grid for (int row = 0; row < N; row++) { for (int col = 0; col < N; col++) { if (perc.isFull(row, col)) { StdDraw.setPenColor(StdDraw.BOOK_LIGHT_BLUE); } else if (perc.isOpen(row, col)) { StdDraw.setPenColor(StdDraw.WHITE); } else { StdDraw.setPenColor(StdDraw.BLACK); } StdDraw.filledSquare(col + 0.5, N - row - 0.5, 0.45); } }
// write status text StdDraw.setFont(new Font("SansSerif", Font.PLAIN, 12)); StdDraw.setPenColor(StdDraw.BLACK); StdDraw.text(.25*N, -N*.025, perc.numberOfOpenSites() + " open sites"); if (perc.percolates()) StdDraw.text(.75*N, -N*.025, "percolates"); else StdDraw.text(.75*N, -N*.025, "does not percolate");
}
private static void simulateFromFile(String filename) { In in = new In(filename); int N = in.readInt(); Percolation perc = new Percolation(N);
// turn on animation mode StdDraw.show(0);
// repeatedly read in sites to open and draw resulting system draw(perc, N); StdDraw.show(DELAY); while (!in.isEmpty()) { int i = in.readInt(); int j = in.readInt(); perc.open(i, j); draw(perc, N); StdDraw.show(DELAY); } }
public static void main(String[] args) { String filename = args[0]; simulateFromFile(filename); } }
.......................................................................................
/****************************************************************************** * Compilation: javac InteractivePercolationVisualizer.java * Execution: java InteractivePercolationVisualizer N * Dependencies: PercolationVisualizer.java Percolation.java * * This program takes the grid size N as a command-line argument. * Then, the user repeatedly clicks sites to open with the mouse. * After each site is opened, it draws full sites in light blue, * open sites (that aren't full) in white, and blocked sites in black. * ******************************************************************************/
import edu.princeton.cs.algs4.StdDraw; import edu.princeton.cs.algs4.StdOut;
public class InteractivePercolationVisualizer { private static final int DELAY = 20;
public static void main(String[] args) { // N-by-N percolation system (read from command-line, default = 10) int N = 10; if (args.length == 1) N = Integer.parseInt(args[0]);
// turn on animation mode StdDraw.show(0);
// repeatedly open site specified my mouse click and draw resulting system //StdOut.println(N);
Percolation perc = new Percolation(N); PercolationVisualizer.draw(perc, N); StdDraw.show(DELAY); while (true) {
// detected mouse click if (StdDraw.mousePressed()) {
// screen coordinates double x = StdDraw.mouseX(); double y = StdDraw.mouseY();
// convert to row i, column j int i = (int) (N - Math.floor(y) - 1); int j = (int) (Math.floor(x));
// open site (i, j) provided it's in bounds if (i >= 0 && i < N && j >= 0 && j < N) { if (!perc.isOpen(i, j)) { //StdOut.println(i + " " + j); } perc.open(i, j); }
// draw N-by-N percolation system PercolationVisualizer.draw(perc, N); } StdDraw.show(DELAY); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
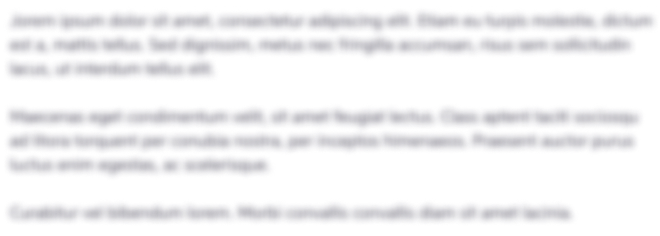
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started