Question
Can you make sure it is C program? and please don't copy it from some one else from chegg... Implement either a singly or doubly
Can you make sure it is C program? and please don't copy it from some one else from chegg...
Implement either a singly or doubly linked list that stores team info records. Each record has the following fields:
-string containing the name of the team. Underscores are used to join individual words (ex: Portland_Timbers) so the full name can be scanned as a single string (%s)
-string containing the nickname (abbreviated name) for the team. Can be scanned as a single string (%s)
-(int) encoding the league the team plays in (OTHER, MLS, NWSL, USL)
-(int) encoding the conference the team plays in (Other, Eastern, Western, NWSL)
-(int-int-int) win-lose-draw record for the 2018 regular season
Lines in the file that start with // are comments and should be ignored. Blank lines should also be ignored.
I have provided functional code that defines and operates on a soccer team info record (soccer_team.c, soccer_team.h). I have also included documentation on the API for this code in both HTML (unzip the file and select index.html to get to the top level) and .pdf format. This documentation was generated bydoxygen ( http://www.doxygen.org/ ) from the source code.
At a minimum, your linked list code should have functions to create a team info node, insert a new node into the list, and retrieve the team information from a node. You do not need to provide the capability to delete a node from the list. Test your linked list API.
/***soccer_team.c***/
/**
* @file soccer_team.c
* @author Roy Kravitz (roy.kravitz@pdx.edu)
* @copyright Portland State University, 2018
* This is the source code for the soccer team information module used in ECE 361 Homework #4. It provides basic
* functionality for creating and getting values from a team information struct. There is also a function
* for displaying all the fields in a team information struct in a readable format. I tried to design this
* module in the vein of a Java-type class, but, of course, OOP is not supported in C.
* MODIFICATION HISTORY:
* Ver Who Date Changes
* 1.00 rhk 11/15/18 First release
******************************************************************************/
#include
#include "soccer_team.h"
/**
* mk_new_team() - allocates a new team struct and populates it. Sort of the same as building a constructor
* space for the team struct is allocated dynamically
* @param name (pointer to a char[]) containing the name of the team
* @param nickname (pointer to a char[]) containing the nickname of the team
* @param league (int) containing the league the team plays in (MLS, NWSL, USL, Other)
* @param conf (int) containing the conference the team plays in (Eastern, Wsstern, NWSL, Other}
* @param nwins (int) containing the number of wins during the regular season
* @param nlosses (int) containing the number of losses during the regular season
* @param ndraws (int) containing the number of draws during the regular season
* @returns a pointer to the new team structure if the struct was created successfully, NULL otherwise
*/
TEAM_PTR_t mk_new_team(char* name, char* nickname, int league, int conf, int nwins, int nlosses, int ndraws)
{
TEAM_PTR_t nt;
int strsize;
// check the name string length. If 0 do not create the struct since name is the primary key to search on
if ((strsize = strlen(name)) <= 0 ) {
fprintf(stderr, "MK_NEW_TEAM: Name field was empty ");
return NULL;
}
else {
// get space for a new team. Return NULL if we couldn't get space
nt = malloc(sizeof(TEAM_t));
if (nt == NULL) {
fprintf(stderr, "MK_NEW_TEAM: Could not create the team record");
return NULL;
}
// so far so good - let's populate the record
strncpy(nt->name, name, strsize);
strncpy(nt->nickname, nickname, strlen(nickname));
nt->league = league;
nt->conf = conf;
nt->wins = nwins;
nt->losses = nlosses;
nt->draws = ndraws;
// return a pointer to the new team record
return nt;
}
}
/**
* get_name() - returns the team name from a team record
* assumes that sufficient space has been allocated for the return string
* @param team_ptr (pointer to the team record) being examined
* @returns a pointer to a char array holding the name. No error checking on the size is done
*/
char* get_name(TEAM_PTR_t team_ptr)
{
static char name_ptr[MAX_TEAM_NAME]; // string containing team name. static so it hangs around after function returns
if (team_ptr == NULL) {
return NULL;
}
strcpy(name_ptr, team_ptr->name);
return name_ptr;
}
/*
* get_nickname() - returns the team nickname from a team record
* assumes that sufficient space has been allocated for the return string
* @param team_ptr (pointer to the team record) being examined
* @returns a pointer to a char array holding the nickname. No error checking on the size is done
*/
char* get_nickname(TEAM_PTR_t team_ptr)
{
static char name_ptr[MAX_TEAM_NICKNAME]; // string containing team nickname. static so it hangs around after function returns
if (team_ptr == NULL) {
return NULL;
}
strcpy(name_ptr, team_ptr->nickname);
return name_ptr;
}
/**
* get_league_str() - returns a string containing the league the team plays in from a team record
* assumes that sufficient space has been allocated for the return string
* @param team_ptr (pointer to the team record) being examined
* @returns an pointer to a char array holding the league the team plays in
*/
char* get_league_str(TEAM_PTR_t team_ptr)
{
static char league_ptr[MAX_LEAGUE_TXT]; // string containing name of league. static so it hangs around after function returns
int l;
if (team_ptr == NULL) {
return NULL;
}
// we have a team record
l = team_ptr->league;
switch (l)
{
case LEAGUE_OTHER: strcpy(league_ptr, "OTHER"); break;
case LEAGUE_MLS: strcpy(league_ptr, "MLS"); break;
case LEAGUE_NWSL: strcpy(league_ptr, "NWSL"); break;
case LEAGUE_USL: strcpy(league_ptr, "USL"); break;
default: strcpy(league_ptr, "?????"); break;
}
return league_ptr;
}
/**
* get_conf_str() - returns a string containing the conference the team plays in from a team record
* assumes that sufficient space has been allocated for the return string
* @param team_ptr (pointer to the team record) being examined
* @returns an pointer to a char array holding the conference the team plays in
*/
char* get_conf_str(TEAM_PTR_t team_ptr)
{
static char conf_ptr[MAX_CONF_TXT]; // string containing name of conference. static so it hangs around after function returns
int c;
if (team_ptr == NULL) {
return NULL;
}
// we have a team record
c = team_ptr->conf;
switch (c)
{
case CONF_OTHER: strcpy(conf_ptr, "OTHER"); break;
case CONF_EASTERN: strcpy(conf_ptr, "EASTERN"); break;
case CONF_WESTERN: strcpy(conf_ptr, "WESTERN"); break;
case CONF_NWSL: strcpy(conf_ptr, "NWSL"); break;
default: strcpy(conf_ptr, "?????"); break;
}
return conf_ptr;
}
/*
* get_league() - returns an int containing the league the team plays in from a team record
* @param team_ptr (pointer to the team record) being examined
* @returns an int holding the league the team plays in
*/
int get_league(TEAM_PTR_t team_ptr){
if (team_ptr == NULL) {
return -1;
}
else {
return team_ptr->league;
}
}
/**
* get_conf() - returns an int containing the conference the team plays in from a team record
* @param team_ptr (pointer to the team record) being examined
* @returns an int holding the conference the team plays in
*/
int get_conf(TEAM_PTR_t team_ptr) {
if (team_ptr == NULL) {
return -1;
}
else {
return team_ptr->conf;
}
}
/**
* get_wins() - returns an int containing then number of games the team won from a team record
* @param team_ptr (pointer to the team record) being examined
* @returns an int holding the number of regular season wins for the team
*/
int get_wins(TEAM_PTR_t team_ptr)
{
if (team_ptr == NULL) {
return -1;
}
else {
return team_ptr->wins;
}
}
/*
* get_losses() - returns an int containing then number of games the team lost from a team record
* @param team_ptr (pointer to the team record) being examined
* @returns an int holding the number of regular season losses for the team
*/
int get_losses(TEAM_PTR_t team_ptr)
{
if (team_ptr == NULL) {
return -1;
}
else {
return team_ptr->losses;
}
}
/*
* get_draws() - returns an int containing then number of games the team tied from a team record
* @param team_ptr (pointer to the team record) being examined
* @returns an int holding the number of regular season draws (ties) for the team
*/
int get_draws(TEAM_PTR_t team_ptr)
{
if (team_ptr == NULL) {
return -1;
}
else {
return team_ptr->draws;
}
}
/*
* display_team_info() - displays the information from a team record on stdout
* @param team_ptr (pointer to the team record) being examined
* @returns nothing
*/
void display_team_info(TEAM_PTR_t team_ptr)
{
if (team_ptr == NULL) {
printf("No Team record to display ");
}
else {
// display the information
printf(
" Team: %s (%s) League: %s (%s) Record: %d-%d-%d ",
get_name(team_ptr),
get_nickname(team_ptr),
get_league_str(team_ptr),
get_conf_str(team_ptr),
get_wins(team_ptr),
get_losses(team_ptr),
get_draws(team_ptr)
);
}
return;
}
/***soccer_team.h***/
/* @file soccer_team.h
* @author Roy Kravitz (roy.kravitz@pdx.edu)
* @copyright Portland State University, 2018
* This header file contains constants, typedefs, identifiers and function prototype(s) for
* the soccer team information module used in ECE 361 Homework #4.
* MODIFICATION HISTORY:
* Ver Who Date Changes
* 1.00 rhk 11/15/18 First release*/
#ifndef SOCCERTEAM_H
#define SOCCERTEAM_H
/**Include Files **/
#include
#include
#include
/** Constants and Macros **/
#define MAX_TEAM_NAME 30 // maximum length of team name string
#define MAX_TEAM_NICKNAME 5 // maximum length of a team nickname string
#define MAX_LEAGUE_TXT 10 // maximum length of text for the league the team plays in
#define MAX_CONF_TXT 10 // maximum length of text for the conference the team plays in
/* enums and typedefs */
typedef enum {LEAGUE_OTHER = 0, LEAGUE_MLS = 1, LEAGUE_NWSL = 2, LEAGUE_USL =3} LEAGUE_t;
typedef enum {CONF_OTHER = 0, CONF_EASTERN = 1, CONF_WESTERN = 2, CONF_NWSL = 3}CONF_t;
typedef struct _teaminfo
{
char name[MAX_TEAM_NAME];
char nickname[MAX_TEAM_NICKNAME];
int league;
int conf;
int wins;
int losses;
int draws;
} TEAM_t, *TEAM_PTR_t;
/*Function prototypes*/
TEAM_PTR_t mk_new_team(char* name, char* nickname, int league,
int conf, int nwins, int nlosses, int ndraws);
char* get_name(TEAM_PTR_t team_ptr);
char* get_nickname(TEAM_PTR_t team_ptr);
char* get_league_str(TEAM_PTR_t team_ptr);
char* get_conf_str(TEAM_PTR_t team_ptr);
int get_league(TEAM_PTR_t team_ptr);
int get_conf(TEAM_PTR_t team_ptr);
int get_wins(TEAM_PTR_t team_ptr);
int get_losses(TEAM_PTR_t team_ptr);
int get_draws(TEAM_PTR_t team_ptr);
void display_team_info(TEAM_PTR_t team_ptr);
#endif // SOCCERTEAM_H
Step by Step Solution
There are 3 Steps involved in it
Step: 1
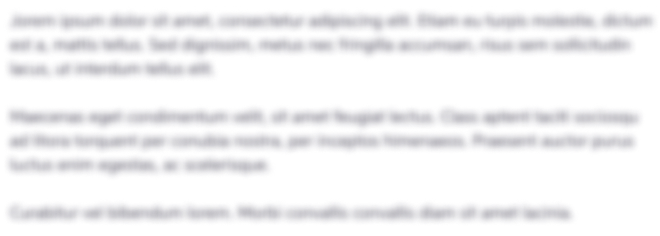
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started