Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Can you please create pseudocode for the code below: #include #include #include CSVparser.hpp using namespace std; / / = = = = = =
Can you please create pseudocode for the code below:
#include
#include
#include "CSVparser.hpp
using namespace std;
Global definitions visible to all methods and classes
forward declarations
double strToDoublestring str char ch;
define a structure to hold bid information
struct Bid
string bidId; unique identifier
string title;
string fund;
double amount;
Bid
amount ;
;
Internal structure for tree node
struct Node
Bid bid;
Node left;
Node right;
default constructor
Node
left nullptr;
right nullptr;
initialize with a bid
NodeBid aBid : Node
bid aBid;
;
Binary Search Tree class definition
Define a class containing data members and methods to
implement a binary search tree
class BinarySearchTree
private:
Node root;
void addNodeNode node, Bid bid;
void inOrderNode node;
Node removeNodeNode node, string bidId;
public:
BinarySearchTree;
virtual ~BinarySearchTree;
void InOrder;
void InsertBid bid;
void Removestring bidId;
Bid Searchstring bidId;
;
Default constructor
BinarySearchTree::BinarySearchTree
root nullptr; Initialize root to nullptr
Destructor
BinarySearchTree::~BinarySearchTree
Recursively delete nodes starting from the root
while root nullptr
Removerootbid.bidId;
Traverse the tree in order left root, right
void BinarySearchTree::InOrder
inOrderroot;
Insert a bid into the tree
void BinarySearchTree::InsertBid bid
if root nullptr
root new Nodebid; Create root node if the tree is empty
else
addNoderoot bid; Call the recursive addNode method to insert the bid
Remove a bid from the tree
void BinarySearchTree::Removestring bidId
root removeNoderoot bidId;
Search for a bid in the tree
Bid BinarySearchTree::Searchstring bidId
Node current root;
while current nullptr
if currentbid.bidId bidId
return currentbid; Found the bid, return it
else if bidId currentbid.bidId
current currentleft; Traverse left if bidId is smaller
else
current currentright; Traverse right if bidId is larger
Bid emptyBid; Return an empty bid if not found
return emptyBid;
Add a bid to a specific node recursive
@param node Current node in tree
@param bid Bid to be added
void BinarySearchTree::addNodeNode node, Bid bid
if bidbidId nodebid.bidId
if nodeleft nullptr
nodeleft new Nodebid; Create a new node if left child is null
else
addNodenodeleft, bid; Recursive call to traverse left subtree
else
if noderight nullptr
noderight new Nodebid; Create a new node if right child is null
else
addNodenoderight, bid; Recursive call to traverse right subtree
Remove a bid from a node recursive
@param node Current node in tree
@param bidId Bid ID to be removed
@return Updated node structure after removal
Node BinarySearchTree::removeNodeNode node, string bidId
if node nullptr
return nullptr; Return nullptr if the tree is empty
if bidId nodebid.bidId
nodeleft removeNodenodeleft, bidId; Recursively search in the left subtree
else if bidId nodebid.bidId
noderight removeNodenoderight, bidId; Recursively search in the right subtree
else
if nodeleft nullptr
Node tempNode noderight;
delete node;
return tempNode; Return the right child if left child is null
else if noderight nullptr
Node tempNode nodeleft;
delete node;
return tempNode; Return the left child if right child is null
If the node has two children, find the minimum value node in the right subtree successor
Node tempNode noderight;
while tempNodeleft nullptr
Step by Step Solution
There are 3 Steps involved in it
Step: 1
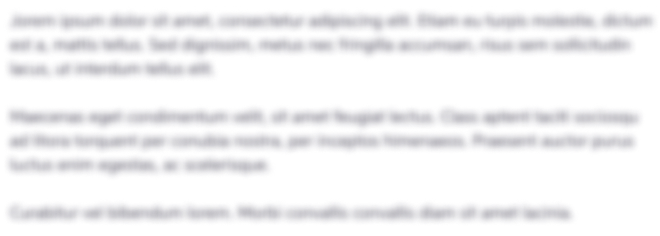
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started