Question
Can you please help me with this project's Main class? Any help that you can give is appreciated. Pseudocode given: Main.java package proj3; import java.io.FileNotFoundException;
Can you please help me with this project's Main class? Any help that you can give is appreciated.
Pseudocode given:
Main.java
package proj3;
import java.io.FileNotFoundException; import javax.swing.JFrame;
/** * The Main class containing the main() and run() methods. */ public class Main {
/** * The Roster of students that is read from the input file "gradebook.dat". */ ???
/** * A reference to the View object. */ ???
/** * This is where execution starts. Instantiate a Main object and then call run(). */ ???
/** * exit() is called when the Exit button in the View is clicked. When we exit we have to write * the roster to the output file "gradebook.dat". Then we exit the program with a code of 0. * * We open the file and write the roster to it in a try-catch block, where we catch a * FileNotFoundException that will be thrown if for some reason, we cannot open "gradebook.dat" * for writing. * * PSEUDOCODE: * method exit() : void * try * instantiate a GradebookWriter object named gbWriter, opening "gradebook.dat" for * writing * call writeGradebook(getRoster()) on gbWriter * call System.exit(0) to terminate the application with an exit code of 0 * catch FileNotFoundException e * call messageBox() on getView() to display a message box containing the text "Could * not open gradebook.dat for writing. Exiting without saving." * call System.exit(-1) to terminate the application with an error code of -1 * end try-catch * end exit */ ???
/** * This method returns the number of exams in the class. */ ???
/** * This method returns the number of homework assignments in the class. */ ???
/** * Accessor method for mRoster. */ private Roster getRoster() { return mRoster; }
/** * Accessor method for mView. */ private View getView() { return mView; }
/** * run() is the main routine and is called from main(). * * PSEUDOCODE: * method run * call JFrame.setDefaultLookAndFeelDecorated(true or false depending on your preference) * -- Create the View passing 'this' as the argument so the View will be linked to the Main * -- class so they may communicate with each other. Then pass the newly created View object * -- to setView() to save the reference to the View in our instance variable mView. * call setView(new View(this)) to create the View and stored the returned object in mView * try * -- Note that when we try to open "gradebook.dat" for reading that GradebookReader() * -- may throw a FileNotFoundException which we catch here. * create a GradbookReader object named gbReader opening "gradebook.dat" for reading * -- Read the student roster from the input file. * call readGradebook() on gbReader, which returns the Roster * call setRoster() on the Roster returned from readGradebook() to save the roster in * our instance variable mRoster * catch * call messageBox() on getView() to display the error message "Could not open * gradebook.dat for reading. Exiting." * call System.exit(-1) to terminate the application with an exit code of -1 * end try-catch * end run */ ???
/** * search() is called when the Search button is clicked in the View. The input parameter is * the last name of the Student to search the roster for. Call getStudent(pLastName) on the * Roster object (call getRoster() to get the reference to the Roster) to get a reference to * the Student with that last name. If the student is not located, getStudent() returns null. * * @param pLastName The last name of the student who we will search the Roster for. * * PSEUDOCODE: * method search(pLastName : String) : Student * call getRoster().getStudent(pLastName) and return what getStudent() returns * end search */ ???
/** * Mutator method for mRoster. */ private void setRoster(Roster pRoster) { mRoster = pRoster; }
/** * Mutator method for mView. */ private void setView(View pView) { mView = pView; } }
3 Background This project shall implement a program which stores grade information for students in a class and allows that grade in- formation to be edited. The data shall be stored in a database le named gradebook.dat. There shall be one student record per line, where the format of a student record is: last-name first-name ex1 ez2 ex3 hw1 hw2 hw3 hwk hws where: last-name The student's last name. A contiguous string of one or more characters, with no spaces. first-rame The student's Erst name. A contiguous string of one or more characters, with no spaces. exi, ex2, ez3 The student's scores on three exams, may be zeros. Each exam is worth 100 pts. hwi-hw5 The student's scores on ve homework assignments, may be zeros. Each assignment is worth 25 pts. Here is an example gradebook dat Ele which you may use for testing if you wish: Sample gradebook. dat Simpson Lisa 100 100 100 25 25 25 25 25 Flintstone Fred 80 60 40 15 17 22 18 23 Jetson George 70 83 81 20 21 22 23 25 Explosion Nathan 7 6 5 4 3 2 1 0 Muntz Nelson 60 70 50 20 15 10 5 8 Terwilliger Robert 80 90 95 23 21 19 17 23 Flanders Ned 85 95 75 12 14 17 23 16 Bouvier Selma 16 16 16 16 16 16 16 16 Spuckler Cletus 1 2 3 4 5 6 7 8 Wiggum Clancy 18 16 14 12 10 8 4 2 Skinner Seymour 78 83 99 19 23 21 24 18 3 Software Requirements The GUI shall be implemented in a class named View. When the program start the View frame shall appear as shown in Fig. 1. 2. The View frame shall be 525 pixels wide and 225 pixels high The program shall display the program name in the title bar. You may change the name of the program to anything 1. 3. you wish. 4. The X close button in the title bar shall be disabled, i.e., when it is clicked the program shall not exit and the View shall remain unchanged. Gred :: Gradebook Editor Student Name: Search Homework Exam: Clear Save Exit 6. Figure 1: The View Frame 5. The student records shall be stored in a gradebook database in a le named gradebook.dat. When the program starts, if the gradebook. dat le cannot be opened for reading, the program shall display an error message dialog (using the JOption Pane.show MessageDialog() method) informing the user that the gradebook database could not be opened for reading and that the program will terminate. When the user clicks the OK button the pro- gram shall terminate. See SR 27. 7. When the program exita, if the gradebook dat le cannot be opened for writing, the program shall display an error message dialog (using the JOption Pane.show MessageDialog() method) informing the user that the gradebook database could not be opened for writing and that clicking the dialog's OK button will cause the program to terminate without updating the gradebook database. When the user click the OK button the program shall terminate. See SR 27. 8. When the program starts, no student record shall be displayed and the text felds shall be empty. See SR 1. 9. The last names of the students in the gradebook database shall be unique (because the last name is the key when searching the database). 10. When the program starts, it shall read the contents of the gradebook database from gradebook. dat and shall sort the list of students into ascending order by last name (per the Software Design requirements, it shall sort using the quick- sort sorting algorithm). The sort is performed so that the program may search the database in memory for a species student record using the binary search algorithm. 11. If the student record for a student who's last name is "Simpson" is being displayed, this is how the View shall appear, displaying the student's full name, homework scores, and exam scores. See SR's 12-14. Gred :: Gradebook Editor Student Name: simpson, usa Search Homework: 25 25 25 25 25 Exam: 10D 100 100 Clear Save Exit Figure 2: The View Displaying a Student's Grades 12. When a student record is being edited, the full name of the student shall be displayed in the Student Name text seld. 13. When a student record is being edited, the student's homework 1-5 scores shall be displayed from left to right in the Homework text selds 14. When a student record is being edited, the student's exam 1-3 scores shall be displayed from left to right in the Exam text Gelds. 15. When the Search button is clicked, if there are values being displayed in the homework and exam Eelda, then those Selds shall be cleared, i.e., set to empty, before the search begins. 16. When the user clicks the Search button and the Student Name text seld is empty, an error message dialog shall be dis- played, requesting that the user enter a student's last name. The Student Name and numeric text selds shall all re- main empty 17. When the user enters a name in the Student Name text Seld and then clicks the Search button, the gradebook data- base in memory shall be searched for a student who's last name matches the name in the text feld. Per the Software Design requirements, the search function shall be implemented either using either iterative binary search or recursive binary search. 18. If the search discussed in SR 17 fails because there are no students whose last name matches the name in the Student Name text feld, then an error message dialog shall be displayed informing the user that a student with that last name could not be found in the gradebook. After the user clicks OK to close the dialog, the Student Name text seld shall be cleared and the numeric text selda shall remain empty 19. If the search discussed in SR 17 succeeds, then the Homework and Eram text selds shall be updated with the stu- dent's homework and exam scores. The Student Name text seld shall continue to display the student's full name per SR 12. 20. When the user is editing the homework and/or exam scores for a student and then clicks the Save button, the student record in memory shall be updated with the new scores. 21. See SR 20. These changes shall remain stored in memory and shall not be written to the gradebook database until the program exite. 22. When no student record is being edited (the homework and exam text selds are empty) and the user click the Save button, nothing shall happen. 23. When the user is editing the information for a student and click the Clear button, without erat clicking the Save but- ton, then the student record in memory shall not be updated even if the homework and/or exam text selda had been modified 24. When no student information is being displayed or edited, and the user clicks the Clear button, then nothing shall happen. 25. When the user is editing and has modised the information for a student and then click the Erit button, the student record and all of the other student records) shall be written to the gradebook database before the program termi- nates. See SR 7 for how write failures are handled. 26. Whether the user is editing student information or not, when the Erit button is clicked, all of the student records shall be written to the gradebook database and then the program shall terminate. 27. Any error message dialogs shall be displayed centered within the View frame and display a message and one button la- beled OK. 4 Software Design Requirements 1. All classes shall be declared in a package named projs. The UML class diagram in UMLet format can be found in the project archive's uml folder. The img folder contains the class diagram in PNG format. Your program shall implement all of the classes (including methods, declaring in- stance and class variables, and so on) of this design. 2. 3. Class Main. A template source code sle for Main is included in the project archive. The Main class shall contain the main() method which shall instantiate an object of the Main class and then call run() on that object. You shall com- plete the code by reading the comments and implementing the paeudocode, while using the UML class diagram for Main as a guide. Main.run() shall catch the FileNotFoundException which may get thrown by Gradebook Reader read Gradebook() and shall terminate the program by displaying an error message diagram which informs the user that the gradebook data- base could not be opened for reading; it shall then terminate the program. See SR 6. Main erit() shall catch the FileNotFoundErception which may be thrown by Gradebook Writer.writeGradebook() when the gradebook database cannot be opened for writing; the handler shall display an error message dialog informing the user that the gradebook database sle could not be opened for writing and that the gradebook will not be updated; it shall then terminate the program. See SR 7. The parameter to Main.search() is the last name of a student and search() shall call Roster.getStudent() to search the Roster for a student with that last name. If the student is found, it will return a Student object which search() shall return. There are eve homework assignments, 30 Main.getNum Homeworks() is a class method which shall return the class con- stant NUM_HOMEWORKS which is declared in Main and is equivalent to 5. Similarly, Mair.getNum Erams() shall return the class constant NUM_EXAMS which is declared in Main and is equivalent to 3. The remainder of the meth- ods in Main are accessor / mutator methods for various data members. 4. Class Gradebook Reader. The constructor of this class creates a Scanner object which is used to read the gradebook database (the Scanner object is stored in instance variable min so that it may be used in the various read methods). Since the ele open may fail, this constructor shall throw the FileNotFoundException to Main.run() which shall catch the exception. This class read the gradebook information from the gradebook database fle gradebook. dat when readGradebook() is called. It then calls read Roster() to read each student record from the input sle and then returns the created Roster object. The read Roster() method &rat instantiates a Roster object, then uses a while loop to read student records from the in- put sle. For each student record, it reads the student's last and Erst names from the input le, creates a Student ob- ject, passing the last name and erst name to the Student constructor, and then calls readHomework() and read Eram() to read the student's homework and exam scores. Then it calls Roster.addStudent() to add the Student object to the Roster. Finally, it returns the Roster object that was created, back to read Gradebook(), which then returns the Roster back to Main.run() which called readGradebook() in the Erst place. Note that after reading the Roster but before returning the Roster to Main.run(), readGradebook() calls Roster.sort- Roster() to sort the Roster. 5. Class Gradebook Writer. This class writes the gradebook information to gradebook. dat before the program exits. It is a subclass of java.io.Print Writer 30 the Gradebook Writer constructor must call the Print Writer constructor to open the Ele for writing. Since the open may fail, Print Writer will return the FileNotFoundException to Gradebook Writer() which will throw it back to Main.erit() which will catch and handle it. The gradebook database is written when write Gradebook() is called. This is a very simple method which just iterates over the Roster, writing each Student object to the sle. 6. Class Roster. The class roster is implemented as an ArrayList of Student objecta. addStudent() is called from Grade- book Reader.readRoster() to add a Student to the Roster's ArrayList. getStudent() is called from Main.search(), which is called by View.action Performed() when the user clicks the Search button in the View.getStudent() calls Searcher search(), passing the Student's last name as the key, search() searches the ArrayList for the student with a matching last name using the binary search algorithm. You shall implement either the iterative or recursive version of the algorithm. If a Student with matching last name is found, then getStudent() re- turns the Student object; otherwise, it returns -1 which represents the "not found" condition. sortRoster() is called from GradebookReader, see that class for a discussion. sortRoster() calls the class method Sorter. sort) passing the ArrayList of Students as the parameter. Upon return, the ArrayList will have been sorted. Note that Roster overrides the inherited toString() method and it returns a String representation of the Roster. The string representation is just the string representation of each Student in the ArrayList, which is formed by calling Stu- dent.toString() on each Student object. Roster.toString() is primarily implemented for use as a useful method to call to print out the Roster during debugging. Roster.getStudentList() and Roster.setStudentList() are accessor/mutator meth- ods for the mStudent List instance variable. 7. Clase Searcher. This class shall implement one public class method int search(ArrayList
Step by Step Solution
There are 3 Steps involved in it
Step: 1
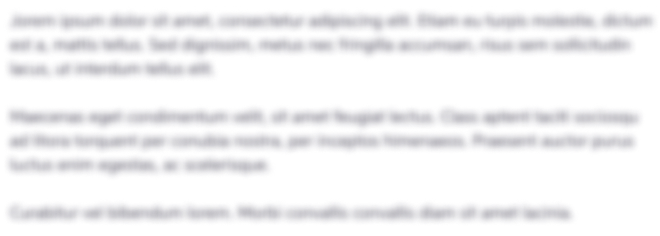
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started