Question
Can you tell me what is wrong with the class? import java.util.*; class ArrayFunctions implements ArrayFunctionsInterface{ --- java is saying this is wrong // create
Can you tell me what is wrong with the class?
import java.util.*; class ArrayFunctions implements ArrayFunctionsInterface{ --- java is saying this is wrong // create array public double [] sortMe(double [] array){ double [] newArray = new double[array.length];
for(int i = 0; i < array.length - 1; i++){ int index = i;
for(int j = i + 1; j < array.length; j ++){ if (array[j] < array[index]) index = j; double smallNumber = array[index]; array[index] = array[i]; array[i] = smallNumber; } return array; } } public double getMax(double [] array){ sortMe(array); return array[array.length - 1]; }
public double getMin(double [] array){ sortMe(array); return array[0]; }
public int whereAmI(double [] array, double searchValue){ int index = 0; for (int i = 0; i < array.length; i++){ if(array[i] == searchValue){ index = i; } } return index; }
public double sumMeUp(double [] array){ double sum = 0; for(int i = 0; i < array.length; i++){ sum += array[i]; } return sum; }
public double[] reverseMe(double [] array){ for(int i = 0; i < array.length/2; i ++){ double temp = array[i]; array[i] = array[array.length - i - 1]; array[array.length - i - 1] = temp; } return array; }
public void printMe(double [] array){ for(int i = 0; i < array.length; i++){ System.out.println(array[i] + " "); } } public double[] doubleMyCapactiy(double[] array){ double [] newArray = new double[array.length * 2]; for(int i = 0; i < array.length; i++){ newArray[i] = array[i]; } return newArray; } }
---------
below is the interface:
public interface ArrayFunctionsInterface{ double [ ] sortMe(double [ ] array); double getMax(double [ ] array); double getMin(double [ ] array); int whereAmI(double [ ] array, double searchValue); double sumMeUp(double [ ] array); double [ ] reverseMe(double [ ] array); void printMe(double [ ] array); double[ ] doubleMyCapacity(double [ ] array); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
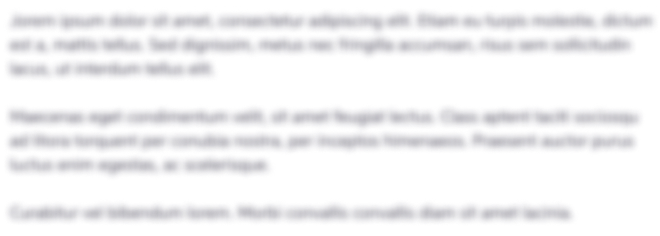
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started