Question
Cant figure it out. Need to Add code for the methods addBoxcarToFront and removeBoxcarFromFront. Do not change the tests or methods. Should complete all tests.
Cant figure it out. Need to Add code for the methods addBoxcarToFront and removeBoxcarFromFront. Do not change the tests or methods. Should complete all tests. Show output if possible. Thank you
Using JUnit version 4.12 and hamcrest-core-1.3.
public class SingleLinkList { private Boxcar head; private int size; public SingleLinkList() { head = null; size = 0; } public String toString() { String s = "engine"; if (head != null) { Boxcar boxcar = head; do { s = s + "->" + boxcar.data().toString(); boxcar = boxcar.next(); } while (boxcar != null); } return s; } public int size() { return size; } public void addBoxcarToEnd(Boxcar b) { if (head == null) head = b; else { // Find the last boxcar and add it to the end Boxcar boxcar = head; while (boxcar.next() != null) boxcar = boxcar.next();
boxcar.setNext(b); } size++; } public void addBoxcarToFront(Boxcar b) { } public void removeBoxcarFromFront() { } public void removeBoxcarFromEnd() { if (head != null) { // If there is just one boxcar remove it if (head.next() == null) head = null; else { // Find the last boxcar and remove it Boxcar boxcar = head; Boxcar prevBoxcar = head; while (boxcar.next() != null) { prevBoxcar = boxcar; boxcar = boxcar.next(); } prevBoxcar.setNext(null); } size--; } } }
TEST
import static org.junit.Assert.*;
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
public class SingleLinkListTest
{
@Test public void testAddOneToFront() { SingleLinkList sll = new SingleLinkList(); Boxcar bc1 = new Boxcar("first"); sll.addBoxcarToFront(bc1); assertEquals("engine->first", sll.toString()); assertEquals(1, sll.size()); }
@Test public void testAddTwoToFront() { SingleLinkList sll = new SingleLinkList(); Boxcar bc1 = new Boxcar("first"); Boxcar bc2 = new Boxcar("second"); sll.addBoxcarToFront(bc1); sll.addBoxcarToFront(bc2); assertEquals("engine->second->first", sll.toString()); assertEquals(2, sll.size()); }
@Test public void testAddFifteenToFront() { SingleLinkList sll = new SingleLinkList(); for (int i = 1; i < 16; i++) { Boxcar bc = new Boxcar(i + ""); sll.addBoxcarToFront(bc); } assertEquals("engine->15->14->13->12->11->10->9->8->7->6->5->4->3->2->1", sll.toString()); assertEquals(15, sll.size()); }
@Test public void testAddOneToEnd() { SingleLinkList sll = new SingleLinkList(); Boxcar bc1 = new Boxcar("first"); sll.addBoxcarToEnd(bc1); assertEquals("engine->first", sll.toString()); assertEquals(1, sll.size()); } @Test public void testAddTwoToEnd() { SingleLinkList sll = new SingleLinkList(); Boxcar bc1 = new Boxcar("first"); Boxcar bc2 = new Boxcar("second"); sll.addBoxcarToEnd(bc1); sll.addBoxcarToEnd(bc2); assertEquals("engine->first->second", sll.toString()); assertEquals(2, sll.size()); } @Test public void testAddFifteenToEnd() { SingleLinkList sll = new SingleLinkList(); for (int i = 1; i < 16; i++) { Boxcar bc = new Boxcar(i + ""); sll.addBoxcarToEnd(bc); } assertEquals("engine->1->2->3->4->5->6->7->8->9->10->11->12->13->14->15", sll.toString()); assertEquals(15, sll.size());
@Test public void testRemoveFromFront() { SingleLinkList sll = new SingleLinkList(); Boxcar bc1 = new Boxcar("first"); Boxcar bc2 = new Boxcar("second"); sll.addBoxcarToFront(bc1); sll.addBoxcarToEnd(bc2); assertEquals("engine->first->second", sll.toString()); assertEquals(2, sll.size()); sll.removeBoxcarFromFront(); assertEquals("engine->second", sll.toString()); assertEquals(1, sll.size()); sll.removeBoxcarFromFront(); assertEquals("engine", sll.toString()); assertEquals(0, sll.size()); } @Test public void testRemoveFromEnd() { SingleLinkList sll = new SingleLinkList(); Boxcar bc1 = new Boxcar("first"); Boxcar bc2 = new Boxcar("second"); sll.addBoxcarToFront(bc1); sll.addBoxcarToEnd(bc2); assertEquals("engine->first->second", sll.toString()); assertEquals(2, sll.size()); sll.removeBoxcarFromEnd(); assertEquals("engine->first", sll.toString()); assertEquals(1, sll.size()); sll.removeBoxcarFromEnd(); assertEquals("engine", sll.toString()); assertEquals(0, sll.size()); } @Test public void testAssortedAddsRemoves() { SingleLinkList sll = new SingleLinkList(); Boxcar bc1 = new Boxcar("first"); Boxcar bc2 = new Boxcar("second"); Boxcar bc3 = new Boxcar("third"); sll.addBoxcarToFront(bc1); sll.addBoxcarToEnd(bc2); sll.addBoxcarToEnd(bc3); assertEquals("engine->first->second->third", sll.toString()); assertEquals(3, sll.size()); sll.removeBoxcarFromEnd(); sll.addBoxcarToFront(bc3); assertEquals("engine->third->first->second", sll.toString()); assertEquals(3, sll.size()); sll.removeBoxcarFromEnd(); sll.addBoxcarToFront(bc2); assertEquals("engine->second->third->first", sll.toString()); assertEquals(3, sll.size()); sll.removeBoxcarFromEnd(); sll.addBoxcarToFront(bc1); assertEquals("engine->first->second->third", sll.toString()); assertEquals(3, sll.size()); sll.removeBoxcarFromEnd(); assertEquals("engine->first->second", sll.toString()); assertEquals(2, sll.size()); sll.removeBoxcarFromFront(); assertEquals("engine->second", sll.toString()); assertEquals(1, sll.size()); sll.removeBoxcarFromEnd(); assertEquals("engine", sll.toString()); assertEquals(0, sll.size()); } @Test public void testRemoveFromEnds() { SingleLinkList sll = new SingleLinkList(); for (int i = 1; i < 16; i++) { Boxcar bc = new Boxcar(i + ""); sll.addBoxcarToFront(bc); } assertEquals("engine->15->14->13->12->11->10->9->8->7->6->5->4->3->2->1", sll.toString()); assertEquals(15, sll.size()); sll.removeBoxcarFromFront(); sll.removeBoxcarFromEnd(); assertEquals("engine->14->13->12->11->10->9->8->7->6->5->4->3->2", sll.toString()); assertEquals(13, sll.size()); sll.removeBoxcarFromFront(); sll.removeBoxcarFromEnd(); sll.removeBoxcarFromFront(); assertEquals("engine->12->11->10->9->8->7->6->5->4->3", sll.toString()); assertEquals(10, sll.size()); sll.removeBoxcarFromEnd(); sll.removeBoxcarFromFront(); sll.removeBoxcarFromEnd(); sll.removeBoxcarFromFront(); sll.removeBoxcarFromEnd(); assertEquals("engine->10->9->8->7->6", sll.toString()); assertEquals(5, sll.size()); for (int i = 0; i < 5; i++) sll.removeBoxcarFromEnd(); assertEquals("engine", sll.toString()); assertEquals(0, sll.size()); } }
BOXCAR code if needed
public class Boxcar {
// instance variables
private String data;
private Boxcar next;
//Constructor for objects of class Boxcar
public Boxcar(String s)
{
// initialise instance variables
data = s;
next = null;
}
public void setNext(Boxcar n)
{
next = n;
}
public Boxcar next()
{
return next;
}
public void setData(String s)
{
data = s;
}
public String data()
{
return data
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
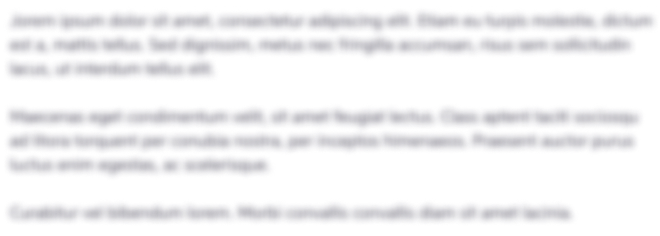
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started