Question
**Cant use Arrays, have to use strings or int variables for storing the users guesses and secret numbers** Design and write Java a program that
**Cant use Arrays, have to use strings or int variables for storing the users guesses and secret numbers**
Design and write Java a program that allows a user to play the classic game of Mastermind. In this game, the computer chooses a random 4 digit number that the player must guess. When the player makes a guess, the computer tells the user how many digits in their 4 digit guess match the digit in the same position in the randomly chosen number. For example, if the computer chooses the random 4 digit number 1442, and the user guesses 4412, the computer will respond with the message "You matched 2". This is because two digits in the user's guess match the digits in the same positions in the computer's randomly chosen number. Specifically, the 2nd and 4th digits match. Here's an example interaction (user input in bold):
----- MASTERMIND -----
Guess the 4 digit number!
Guess 1: 0000 You matched 0
Guess 2: 1111 You matched 1
Guess 3: 1234 You matched 1
Guess 4: 1444 You matched 3
Guess 4: 1442 You matched 4 Congratulations! You guessed the right number in 5 guesses.
Would you like to play again (y/n)?
Part 1 - Implement the Mastermind Class In a file named Mastermind.java, implement the class described by the UML Class Diagram below.
Mastermind
----------------------
- secretNumber: int
- guessCount: int
--------------------------
+ Mastermind() <>
+ pickNewSecretNumber()
+ makeGuess(guess: int): int
+ getGuessCount(): int
- generateSecretNumber(): int
- getRandomDigit(): int
The getRandomDigit method should return a randomly chosen integer between 0 and 9 (inclusive).
The generateSecretNumber method should call the getRandomDigit method 4 times to get the 4 random digits of the new secret number. This method should return the 4 digit secret number as an int.
The pickNewSecretNumber method should call the generateSecretNumber method to get a new 4 digit secret number. This method should store the new secret number in the class level variable named secretNumber, and it should set the value of the class level variable named guessCount to 0.
The default constructor method should call the pickNewSecretNumber method to initialize the game with a randomly chosen 4 digit secret number.
The makeGuess method should compare the digits in the parameter variable (the user's guess) to the digits in the secretNumber. This method should count the number of matching digits and return this number. This method should also increment the class level variable named guessCount by 1.
The getGuessCount method should return the value stored in the class level variable named guessCount.
Part 2 - Implement the main Program Logic In a file named Main.java, implement a main method that meets the requirements detailed below. You should include any other code in this file that you feel is necessary. Here is a pseudocode description of the algorithm you should implement in your main method. Create a Mastermind game object While the user want to play another game Pick a new secret number While the user's guess is not completely correct Prompt the user to make a guess User enters a guess Display number of correct digits in users guess Congratulate the user and tell them the number of guesses they took Ask the user if they want to play again
Step by Step Solution
There are 3 Steps involved in it
Step: 1
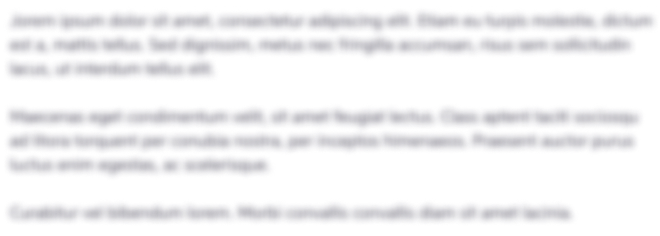
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started