Question
/* * Card.java * * A blueprint class to represent an individual playing card. * * CS 112, Boston University * * completed by: ,
/*
* Card.java
*
* A blueprint class to represent an individual playing card.
*
* CS 112, Boston University
*
* completed by:
*/
public class Card {
// constants for the ranks of non-numeric cards
public static final int ACE = 1;
public static final int JACK = 11;
public static final int QUEEN = 12;
public static final int KING = 13;
// other constants for the ranks
public static final int FIRST_RANK = 1;
public static final int LAST_RANK = 13;
// Arrays of strings for the rank names and abbreviations.
// The name of the rank r is given by RANK_NAMES[r].
// The abbreviation of the rank r is given by RANK_ABBREVS[r].
private static final String[] RANK_NAMES = {
null, "Ace", "2", "3", "4", "5", "6",
"7", "8", "9", "10", "Jack", "Queen", "King"
};
private static final String[] RANK_ABBREVS = {
null, "A", "2", "3", "4", "5", "6",
"7", "8", "9", "10", "J", "Q", "K"
};
// constants for the suits
public static final int FIRST_SUIT = 0;
public static final int LAST_SUIT = 3;
public static final int CLUBS = 0;
public static final int DIAMONDS = 1;
public static final int HEARTS = 2;
public static final int SPADES = 3;
// Arrays of strings for the suit names and abbreviations.
// The name of the suit s is given by SUIT_NAMES[s].
// The abbreviation of the suit s is given by SUIT_ABBREVS[s].
private static final String[] SUIT_NAMES = {
"Clubs", "Diamonds", "Hearts", "Spades"
};
private static final String[] SUIT_ABBREVS = {
"C", "D", "H", "S"
};
/***** part 2: getSuitNum *****/
private static int getSuitNum(String suit) {
// The return statement below is included so the starter code
// will compile.
// Replace it with your implementation of the method.
public static
return 0;
}
/***** Implement parts 3-7 below. *****/
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
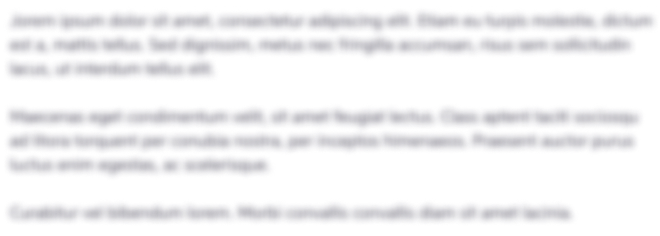
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started