Question
# Card.py class Card(object): '''A simple playing card. A Card is characterized by two components: rank: an integer value in the range 1-13, inclusive (Ace-King)
# Card.py class Card(object): '''A simple playing card. A Card is characterized by two components: rank: an integer value in the range 1-13, inclusive (Ace-King) suit: a character in 'cdhs' for clubs, diamonds, hearts, and spades.'''
SUITS = 'cdhs' SUIT_NAMES = ['Clubs', 'Diamonds', 'Hearts', 'Spades']
RANKS = list(range(1,14)) RANK_NAMES = ['Ace', 'Two', 'Three', 'Four', 'Five', 'Six', 'Seven', 'Eight', 'Nine', 'Ten', 'Jack', 'Queen', 'King']
def __init__(self, rank, suit): '''Constructor pre: rank in range(1,14) and suit in 'cdhs' post: self has the given rank and suit''' print(self.RANKS) self.rank_num = rank self.suit_char = suit def suit(self): '''Card suit post: Returns the suit of self as a single character'''
return self.suit_char
def rank(self): '''Card rank post: Returns the rank of self as an int'''
return self.rank_num def suitName(self): '''Card suit name post: Returns one of ('clubs', 'diamonds', 'hearts', 'spades') corrresponding to self's suit.'''
index = self.SUITS.index(self.suit_char) return self.SUIT_NAMES[index]
def rankName(self): '''Card rank name post: Returns one of ('ace', 'two', 'three', ..., 'king') corresponding to self's rank.'''
index = self.RANKS.index(self.rank_num) return self.RANK_NAMES[index]
def __str__(self): '''String representation post: Returns string representing self, e.g. 'Ace of Spades' '''
return self.rankName() + ' of ' + self.suitName()
--------------------------------------------------------------------------------------------
1. unittests are in a separate file from the Card class.
Card class is already defined and you are to use the one that is given.
2. The ideas (is comparisons for equality are correct, you just need to update your code to use the Card.py file that we have).
3. Keep in mind that we have access to class variables via class name
For example, Card.SUIT_NAMES
-----------------------------------------------------------------------------------------------------------
# unitTestingCard.py # unit testing rank
class Card: dict_rank={"1": "one", "2": "two", "3": "three", "4": "four", "5": "five", "6": "six", "7": "seven", "8": "eight", "9": "nine", "10": "ten", "11": "Joker", "12": "Queen", "13": "King", "14": "ace"}
dict_suites={"c": "clubs", "d": "diomonds", "h": "hearts", "s": "spades"} def __init__(self, rank, suit): self.__suit=suit self.__rank=rank
def rank(self): return self.__rank
def rankName(self): if str(self.__rank) in self.dict_rank.keys(): return self.dict_rank[str(self.__rank)]
def suit(self): return self.__suit
def suitName(self): if self.__suit in self.dict_suites.keys(): return self.dict_suites[self.__suit]
import sys import unittest class RankTest(unittest.TestCase): """ Tests Rank methods: rank() and rankName() """ def testRanks(self): # unit test for ranks 1-13 for i in range(1,14): myCard = Card(i,'c') # create i of clubs self.assertEqual(myCard.rank(),i) # verifies that the card's rank is i
def testRankName(self): # unit test for rank names, 'ace', 'two', 'three',... for i in range(1, 14): myCard=Card(i, 'c') self.assertEqual(myCard.rankName(), myCard.dict_rank[str(i)])
class SuitTest(unittest.TestCase): """ Tests Suit methods: suit() and suitName() """ def testSuits(self): # unit test for suits, 'c', 'd', 'h', 's' for suit in ["c", "d", "h", "s"]: myCard=Card(1, suit) self.assertEqual(myCard.suit(), suit) def testSuitName(self): # unit test for suit names, 'clubs', 'diamonds',... for suit in ["c", "d", "h", "s"]: myCard=Card(1, suit) self.assertEqual(myCard.suitName(), myCard.dict_suites[suit])
def main(argv): unittest.main()
if __name__ == '__main__': main(sys.argv)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
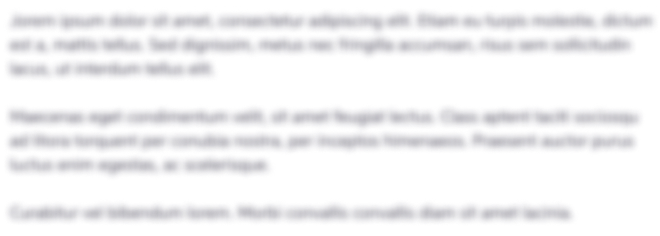
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started