Question
Cars BlockPy Library From the CORGIS Dataset Project Tags: cars, vehicles, fuel Overview This is a dataset about cars and how much fuel they use.
Cars BlockPy Library From the CORGIS Dataset Project Tags: cars, vehicles, fuel Overview This is a dataset about cars and how much fuel they use. Key Descriptions Key List of... Comment Example Value
Dimensions.Height Integer Unknown values are stored as 0. Unfortunately, many cars do not report this data. 140
Dimensions.Length Integer Unknown values are stored as 0. Unfortunately, many cars do not report this data. 143
Dimensions.Width Integer Unknown values are stored as 0. Unfortunately, many cars do not report this data. 202
Engine Information.Driveline String A string representing whether this is "Rear-wheel drive", "Front-wheel drive", or "All-wheel drive". "All-wheel drive"
Engine Information.Engine Type String How many cylinders are in this engine. Most cars are either a 6-cylinder or an 8cylinder. "Audi 3.2L 6 cylinder 250hp 236ftlbs"
Engine Information.Hybrid Boolean Whether this is a hybrid engine or not - that is, if it uses both an internal combustion engine and an electric motor. True
Engine Information.Number of Forward Gears Integer The number of forward gears. If no information is available, it is coded as 0. 6
Engine Information.Transmission String The full name of this type of transmission, based on its Classification and number of forward gears. "6 Speed Automatic Select Shift"
Fuel Information.City mpg Integer The miles-per-gallon this car gets on average in cities. 18
Fuel Information.Fuel Type String Whether this car takes "Gasoline", "Diesel fuel", "Electricity", "Compressed natural gas", or "E85" (a term that refers to high-level ethanol-gasoline blends containing 51%-83% ethanol). If it is unknown, it is left blank. "Gasoline"
Fuel Information.Highway mpg Integer The miles-per-gallon this car gets on average on highways. 25
Identification.Classification String Whether this is a "Manual transmission" or an "Automatic transmission". If it is unknown, it is left blank. "Automatic transmission "
Identification.ID String A unique ID for this particular car, using the year, make, model, and transmission type. "2009 Audi A3 3.2"
Identification.Make String The maker for this car. "Audi"
Identification.Model Year String The specific name/year for this car. "2009 Audi A3"
Identification.Year Integer The year that this car was released. 2009
Engine Information.Engine Statistics.Horsepower Integer A measure of the engine's power. A unit of power equal to 550 foot-pounds per second (745.7 watts). 250
Engine Information.Engine Statistics.Torque Integer The torque of the engine, measured in lb/ft. When an engine is said to make "200 lb-ft of torque", it means that 200 pounds of force on a 1-foot lever is needed to stop its motion. 236
TASK 1
The idea of this project is to create a text-based application with the following options:
- List all cars. This option will read from the file and when the user selects this option, the program will list all the cars in the file.
- Find via Linear search. This option will allow the user to type a model of the car (ID field in the dataset) and it should print out the information about the car searched, the amount of time it took to find the car using linear search. For this option, create a class called SearchMethods and implement the method :
public static
- Find via Binary search. This option will allow the user to type a model of the car (ID field in the dataset) and it should print out the information about the car searched, and the amount of time it took to find the car using binary search. For this option create, a class called SearchMethods and implement the method:
public static
int binarySearch(T[] data, int min, int max, T target)
in both 2 and 3 the binary and linear search methods return the position in the array where the elements are found.
Remember: To apply binary Search you must sort the array. Measure the time to execute binary search only when calling binary search, not on the sorting part of it.
- Option to quit the program.
TASK 2
Time Complexity: Build a table and record the time complexity taken by linear and binary search to look for an element with the following array sizes: 10, 50, 100, 200, 500, 700, 1000. Compare the performance of both algorithms highlighting the time complexity for both algorithms when run on small input size and when run on large input size.
To accomplish this, load a list with the file data and then create a program that selects at random X amount of elements from the original list (where X is a value that the user selects) and place them in an array, then select at random an element in the original list to search in the sampled array using both algorithms. Measure the time it takes for both algorithms on an array of a given input size.
For this part just create a class called Task2Exercise in your project, reuse any code you did for the first part.
TASK 3
Using one of the sorting methods, sort the Car ArrayList based on their City mpg feature. Justify your choice of the algorithm used.
HINTS:
- You need to create a class called Car that implements the Comparable interface and load the data from the file to a list. Then somehow convert the arraylist to an array to use the implementations of the algorithms
- To measure the time it took to run, you can use System.nanoTime() or System.currentTimeMillis before and after the method is executed (see here: https://docs.oracle.com/javase/7/docs/api/java/lang/System.html) and subtract.
Output::
- A IntelliJ project implementing the tasks
- A report on the second exercise showing a graph of running time vs. input size of both algorithms and your conclusions.
Special Note: Please complete in IntelliJ and make the code as simple as possible Please provide note for understanding. Thank you.
25 A B C D E F G H I J K L M N O P Q R City mpg Classificat Driveline Engine Ty Fuel Type Height Highway r Horsepow Hybrid ID Length Make Model Ye: Number o Torque Transmiss Width Year 18 Automatic All-wheel Audi 3.2L Gasoline 140 250 FALSE 2009 Audi 143 Audi 2009 Audi 6 236 6 Speed A 202 2009 22 Automatic Front-whe Audi 2.0L Gasoline 140 200 FALSE 2009 Audi 143 Audi 2009 Audi 207 6 Speed A 202 2009 21 Manual tr: Front-whe Audi 2.0L Gasoline 140 30 200 FALSE 2009 Audi 143 Audi 2009 Audi 207 6 Speed M 2009 21 Automatic All-wheel Audi 2.0L Gasoline 140 28 200 FALSE 2009 Audi 143 Audi 2009 Audi 207 6 Speed A 2009 21 Automatic All-wheel Audi 2.0L Gasoline 140 28 200 FALSE 2009 Audi 143 Audi 2009 Audi 207 6 Speed A 2009 16 Manual tr All-wheel Audi 3.2L Gasoline 265 FALSE 2009 Audi 17 Audi 2009 Audi 243 6 Speed 2009 17 Automatic All-wheel Audi 3.2L Gasoline 91 26 265 FALSE 2009 Audi 17 Audi 2009 Audi 243 6 Speed A 2009 13 Automatic All-wheel Audi 4.2L Gasoline 201 18 350 FALSE 2009 Audi 221 Audi 2009 Audi 325 6 Speed A 2009 14 Automatic All-wheel Audi 3.6L Gasoline 280 FALSE 2009 Audi 221 Audi 2009 Audi 266 6 Speed A 2009 22 Manual tri All-wheel Audi 2.0L Gasoline 211 FALSE 2009 Audi 96 Audi 2009 Audi 258 6 Speed M 2009 21 Automatic All-wheel Audi 2.0L Gasoline 211 FALSE 2009 Audi 96 Audi 2009 Audi 258 6 Speed A 2009 17 Automatic All-wheel Audi 3.2L Gasoline 147 265 FALSE 2009 Audi 96 Audi 2009 Audi 243 6 Speed A 2009 20 Automatic Front-whe Acura 3.5L Gasoline 172 280 FALSE 2012 Acuri 63 Acura 2012 Acur 254 6 Speed A 87 2012 18 Automatic All-wheel Acura 3.7L Gasoline 172 305 FALSE 2012 Acuri 63 Acura 2012 Acuri 273 6 Speed A 2012 12 Automatic All-wheel BMW 4.4L Gasoline 555 FALSE 2010 BMW 243 BMW 2010 BMW 500 6 Speed A 2010 12 Automatic All-wheel BMW 4.4L Gasoline 226 17 555 FALSE 2011 BMW 243 BMW 2011 BMW 500 6 Speed A 2011 12 Automatic All-wheel BMW 4.4L Gasoline 148 17 555 FALSE 2010 BMW 12 BMW 2010 BMW 500 6 Speed A 2010 12 Automatic All-wheel BMW 4.4L Gasoline 148 555 FALSE 2011 BMW 12 BMW 2011 BMW 500 6 Speed A 2011 18 Manual tr: Rear-whe Chevrolet Gasoline 112 185 FALSE 2012 Chev 22 Chevrolet 2012 Chev 190 5 Speed 2012 18 Manual tri Rear-whe Chevrolet Gasoline 185 FALSE 2012 Chev 140 Chevrolet 2012 Chev 190 5 Speed M 2012 18 Automatic Rear-whe Chevrolet Gasoline 185 FALSE 2012 Chev 140 Chevrolet 2012 Chev 190 4 Speed A 181 2012 201 147 226 25 A B C D E F G H I J K L M N O P Q R City mpg Classificat Driveline Engine Ty Fuel Type Height Highway r Horsepow Hybrid ID Length Make Model Ye: Number o Torque Transmiss Width Year 18 Automatic All-wheel Audi 3.2L Gasoline 140 250 FALSE 2009 Audi 143 Audi 2009 Audi 6 236 6 Speed A 202 2009 22 Automatic Front-whe Audi 2.0L Gasoline 140 200 FALSE 2009 Audi 143 Audi 2009 Audi 207 6 Speed A 202 2009 21 Manual tr: Front-whe Audi 2.0L Gasoline 140 30 200 FALSE 2009 Audi 143 Audi 2009 Audi 207 6 Speed M 2009 21 Automatic All-wheel Audi 2.0L Gasoline 140 28 200 FALSE 2009 Audi 143 Audi 2009 Audi 207 6 Speed A 2009 21 Automatic All-wheel Audi 2.0L Gasoline 140 28 200 FALSE 2009 Audi 143 Audi 2009 Audi 207 6 Speed A 2009 16 Manual tr All-wheel Audi 3.2L Gasoline 265 FALSE 2009 Audi 17 Audi 2009 Audi 243 6 Speed 2009 17 Automatic All-wheel Audi 3.2L Gasoline 91 26 265 FALSE 2009 Audi 17 Audi 2009 Audi 243 6 Speed A 2009 13 Automatic All-wheel Audi 4.2L Gasoline 201 18 350 FALSE 2009 Audi 221 Audi 2009 Audi 325 6 Speed A 2009 14 Automatic All-wheel Audi 3.6L Gasoline 280 FALSE 2009 Audi 221 Audi 2009 Audi 266 6 Speed A 2009 22 Manual tri All-wheel Audi 2.0L Gasoline 211 FALSE 2009 Audi 96 Audi 2009 Audi 258 6 Speed M 2009 21 Automatic All-wheel Audi 2.0L Gasoline 211 FALSE 2009 Audi 96 Audi 2009 Audi 258 6 Speed A 2009 17 Automatic All-wheel Audi 3.2L Gasoline 147 265 FALSE 2009 Audi 96 Audi 2009 Audi 243 6 Speed A 2009 20 Automatic Front-whe Acura 3.5L Gasoline 172 280 FALSE 2012 Acuri 63 Acura 2012 Acur 254 6 Speed A 87 2012 18 Automatic All-wheel Acura 3.7L Gasoline 172 305 FALSE 2012 Acuri 63 Acura 2012 Acuri 273 6 Speed A 2012 12 Automatic All-wheel BMW 4.4L Gasoline 555 FALSE 2010 BMW 243 BMW 2010 BMW 500 6 Speed A 2010 12 Automatic All-wheel BMW 4.4L Gasoline 226 17 555 FALSE 2011 BMW 243 BMW 2011 BMW 500 6 Speed A 2011 12 Automatic All-wheel BMW 4.4L Gasoline 148 17 555 FALSE 2010 BMW 12 BMW 2010 BMW 500 6 Speed A 2010 12 Automatic All-wheel BMW 4.4L Gasoline 148 555 FALSE 2011 BMW 12 BMW 2011 BMW 500 6 Speed A 2011 18 Manual tr: Rear-whe Chevrolet Gasoline 112 185 FALSE 2012 Chev 22 Chevrolet 2012 Chev 190 5 Speed 2012 18 Manual tri Rear-whe Chevrolet Gasoline 185 FALSE 2012 Chev 140 Chevrolet 2012 Chev 190 5 Speed M 2012 18 Automatic Rear-whe Chevrolet Gasoline 185 FALSE 2012 Chev 140 Chevrolet 2012 Chev 190 4 Speed A 181 2012 201 147 226Step by Step Solution
There are 3 Steps involved in it
Step: 1
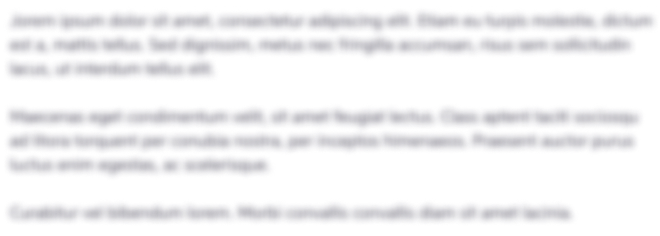
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started