Question
C++ Chapter 10 defined the class circleType to implement the basic properties of a circle. (Add the function print to this class to output the
C++
Chapter 10 defined the class circleType
to implement the basic properties of a circle. (Add the function print
to this class to output the radius, area, and circumference of a circle.) Now every cylinder has a base and height, where the base is a circle. Design a class cylinderType
that can capture the properties of a cylinder and perform the usual operations on the cylinder. Derive this class from the class circleType
designed in Chapter 10. Some of the operations that can be performed on a cylinder are as follows: calculate and print the volume, calculate and print the surface area, set the height, set the radius of the base, and set the center of the base. Also, write a program to test various operations on a cylinder. Assume the value of \piπ to be 3.14159.
circleType.h document
#ifndef circleType_H
#define circleType_H
class circleType
{
public:
void print();
void setRadius(double r);
//Function to set the radius.
//Postcondition: if (r >= 0) radius = r;
// otherwise radius = 0;
double getRadius();
//Function to return the radius.
//Postcondition: The value of radius is returned.
double area();
//Function to return the area of a circle.
//Postcondition: Area is calculated and returned.
double circumference();
//Function to return the circumference of a circle.
//Postcondition: Circumference is calculated and returned.
circleType(double r = 0);
//Constructor with a default parameter.
//Radius is set according to the parameter.
//The default value of the radius is 0.0;
//Postcondition: radius = r;
private:
double radius;
};
#endif
circleType.imp document
//ImplementatioFile for the class circleType
#include
#include "circleType.h"
using namespace std;
void circleType::print()
{
cout << "Radius = " << radius
<< ", area = " << area()
<< ", circumference = " << circumference();
}
void circleType::setRadius(double r)
{
if (r >= 0)
radius = r;
else
radius = 0;
}
double circleType::getRadius()
{
return radius;
}
double circleType::area()
{
return 3.1416 * radius * radius;
}
double circleType::circumference()
{
return 2 * 3.1416 * radius;
}
circleType::circleType(double r)
{
setRadius(r);
}
Step by Step Solution
3.40 Rating (159 Votes )
There are 3 Steps involved in it
Step: 1
Program circleTypeh ifndef circleTypeH define circleTypeH class circleType public void print void setRadiusdouble r Function to set the radius Postcon...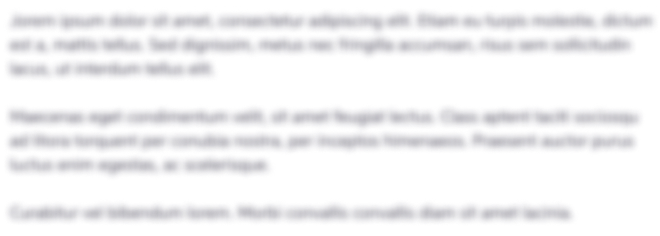
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started