Question
Change java files to C# Convert Fraction.java to C# Convert FractionApp.java to C# /** * Fraction class used to create fraction objects, * with a
Change java files to C#
Convert Fraction.java to C#
Convert FractionApp.java to C#
/**
* Fraction class used to create fraction objects,
* with a numerator and denominator (like 3/4)
*
* Includes no, one, and two argument constructors,
* equals and toString methods, and methods to
* reduce to lowest terms, add, subt, mult, div fraction
* get/set methods are optional
*/
public class Fraction {
private int num;
private int denom;
/**
* Fraction creates a new fraction
*
* @param n The numerator
* @param d The denominator
*/
public Fraction(int n, int d) {
num = n;
denom = d;
reduce(); // reduce within the constructor
} // end constructor Fraction(int n, int d)
/**
* Fraction creates a default fraction 1/1
*/
public Fraction() {
num = 1;
denom = 1;
} // end constructor Fraction()
/**
* Fraction creates a new fraction with a whole number
*
* @param n The numerator
* @param d The denominator
*/
public Fraction(int n) {
num = n;
denom = 1;
} // end constructor Fraction(int n)
/**
* returns the fraction as a String
* in the from num/dem (like 3/4)
*/
public String toString() {
return (num + "/" + denom);
} // end print
/**
* modifies the numerator and denominator so the fraction
* is reduced to lowest terms
*/
private void reduce() {
boolean negative = false; // assume not negative
if (num
negative = true;
num = num * -1;
} // end if
int bound = num; // test if values 2 through num are factors
for (int i = 2; i
// if remainder is zero for both num & den, then factor
if (num%i == 0 && denom%i == 0) {
num = num/i;
denom = denom/i;
// have to check if values is again a factor
//(EX: 80/120 =40/60=20/30=10/15)
i--;
} // end if
} // end for
// if negative, change back to negative after reducing
if (negative) num = num * -1;
} // end reduce
/**
* plus adds this fraction to the parameter and returns the result
*
* @param f The fraction to add to
* @return This fraction plus f
*/
public Fraction plus(Fraction f) {
int newNum = num*f.denom + f.num*denom;
int newDenom = denom*f.denom;
return new Fraction(newNum, newDenom); // create and reduce new fraction
} // end plus
/**
* minus takes this fraction minus the parameter and returns the result
*
* @param f The fraction to subtract
* @return This fraction minus f
*/
public Fraction minus(Fraction f) {
int newNum = num*f.denom - f.num*denom;
int newDenom = denom*f.denom;
return new Fraction(newNum, newDenom); // create and reduce new fraction
} // end minus
/**
* times multiplies this fraction by the parameter and returns the result
*
* @param f The fraction to multiply by
* @return This fraction times f
*/
public Fraction times(Fraction f) {
int newNum = num*f.num;
int newDenom = denom*f.denom;
return new Fraction(newNum, newDenom); // create and reduce new fraction
} // end times
/**
* divide takes this fraction divided by the parameter and returns the result
*
* @param f The fraction to divide by
* @return This fraction divided by f
*/
public Fraction divide(Fraction f) {
int newNum = num*f.denom;
int newDenom = denom*f.num;
return new Fraction(newNum, newDenom); // create and reduce new fraction
} // end divide
/**
* equals determines if two reduced fractions have the same num/denom
*
* @param f The fraction to test for equality
* @return true if equal, else false
*/
public boolean equals (Fraction f) {
return ( f.num == this.num && f.denom == this.denom);
} // end equals
} // end class Fraction
____________________________________________
/**
* Gets two fractions and an operation (+,-,*,/) from
* the user, and displays the result, reducing all fractions
* to lowest terms.
*/
import java.util.*;
public class FractionApp {
public static void main(String[] args) {
Scanner s = new Scanner(System.in);
// create an array of fractions
Fraction [] fractionArray = new Fraction[3];
// read in Initial Expression
System.out.print("Enter the expression (like 2/3 + 3/4 or 3 - 1/2): ");
String line = s.nextLine();
// Works with positives and negative values
// Split equation into first number/fraction, operator, second number/fraction
StringTokenizer st = new StringTokenizer(line, " ");
String first = st.nextToken();
char op = (st.nextToken()).charAt(0);
String second = st.nextToken();
// Determine if first value is a whole number or fraction
String [ ] pieces = first.split("/");
if (pieces.length == 1) // whole number, call one-arg constructor
fractionArray[0] = new Fraction(Integer.parseInt(pieces[0]));
else // fraction, call two-arg constructor
// Split into numeration and denominator and create fraction object
fractionArray[0] = new Fraction(Integer.parseInt(pieces[0]),
Integer.parseInt(pieces[1]));
// Determine if second value is a whole number or fraction
pieces = second.split("/");
if (pieces.length == 1) // whole number, call one-arg constructor
fractionArray[1] = new Fraction(Integer.parseInt(pieces[0]));
else // fraction, call two-arg constructor
// Split into numeration and denominator and create fraction object
fractionArray[1] = new Fraction(Integer.parseInt(pieces[0]),
Integer.parseInt(pieces[1]));
boolean flag = true; // error if operator is not +, -, *, /
switch (op) {
case '+':
// 'plus' returns a reduced fraction object
fractionArray[2] = fractionArray[0].plus(fractionArray[1]);
break;
case '-':
// 'minus' returns a reduced fraction object
fractionArray[2] = fractionArray[0].minus(fractionArray[1]);
break;
case '*':
// 'times' returns a reduced fraction object
fractionArray[2] = fractionArray[0].times(fractionArray[1]);
break;
case '/':
// 'divide' returns a reduced fraction object
fractionArray[2] = fractionArray[0].divide(fractionArray[1]);
break;
default:
System.out.println("Invalid operator. Rerun the program.");
flag = false;
} // end switch-case
if (flag) {
System.out.print(" " + fractionArray[0]); // calls toString method
System.out.print(" " + op + " ");
System.out.print(fractionArray[1]); // calls toString method
System.out.print(" = ");
System.out.print(fractionArray[2]); // calls toString method
System.out.println();
if (fractionArray[0].equals(fractionArray[1]))
System.out.println("Fraction1 equals Fraction2");
else
System.out.println("Fraction1 does NOT equal Fraction2");
} // end if
} // end main
} // end class
Assignment Description: Partial credit given if only completing/submitting Part1 Part 1 (20 pts): Create a non-GUI (Console) C# application to do the following: To demonstrate the similarities between Java and C#, simply convert the Java files created for Project 6 (Fraction and Fraction IPI) into C# (cs) files. Functionality Input Output will be the same as Proj 6 (shown below). For reference, assignment description of Project 6 is available in Canvas through the Project 9 assignment description. C:. Windows' system32Cmd.exe Enter the expression (like 2/3 + 3/4): 80/12012/16 2/33/417/12 To assist those who had problems with Proj 6, a Java solution to Proj 6 is posted in Canvas (under Programming Projects) that you can modify. Only error checking required is to check for a valid operator (+,*). Modification of the posted solution is allowed. Part 1 can be done using Visual Studio (call the Project Solution Proj9_Partl) or using a text editor with the C# command line compiler (submit both files in a zipped folder Pro,Part) Part l "MVC" Optional Extra Credit (+5 pts). This applies to Part I only ( can be done in less than 30 min). You do not need to create a separate solution. Just modify the Part 1 solution to use 3 classes rather than2 Convert the two java files created for Project 6 (Fraction and FractionApp) into THREE cs files using the Model-View-Controller architecture: Fraction (Model). I0 (View) and FractionApp (Controler). All I/O must be done within your I0 class. Your controller class will contain ONLY a Main method (and methods that are not a required part of the Fraction or IO class) and will NOT contain any Input or Output statements. Refer to MVC lecture notes, examples, and Lab 9 and Project 7 for reference Assignment Description: Partial credit given if only completing/submitting Part1 Part 1 (20 pts): Create a non-GUI (Console) C# application to do the following: To demonstrate the similarities between Java and C#, simply convert the Java files created for Project 6 (Fraction and Fraction IPI) into C# (cs) files. Functionality Input Output will be the same as Proj 6 (shown below). For reference, assignment description of Project 6 is available in Canvas through the Project 9 assignment description. C:. Windows' system32Cmd.exe Enter the expression (like 2/3 + 3/4): 80/12012/16 2/33/417/12 To assist those who had problems with Proj 6, a Java solution to Proj 6 is posted in Canvas (under Programming Projects) that you can modify. Only error checking required is to check for a valid operator (+,*). Modification of the posted solution is allowed. Part 1 can be done using Visual Studio (call the Project Solution Proj9_Partl) or using a text editor with the C# command line compiler (submit both files in a zipped folder Pro,Part) Part l "MVC" Optional Extra Credit (+5 pts). This applies to Part I only ( can be done in less than 30 min). You do not need to create a separate solution. Just modify the Part 1 solution to use 3 classes rather than2 Convert the two java files created for Project 6 (Fraction and FractionApp) into THREE cs files using the Model-View-Controller architecture: Fraction (Model). I0 (View) and FractionApp (Controler). All I/O must be done within your I0 class. Your controller class will contain ONLY a Main method (and methods that are not a required part of the Fraction or IO class) and will NOT contain any Input or Output statements. Refer to MVC lecture notes, examples, and Lab 9 and Project 7 for reference
Step by Step Solution
There are 3 Steps involved in it
Step: 1
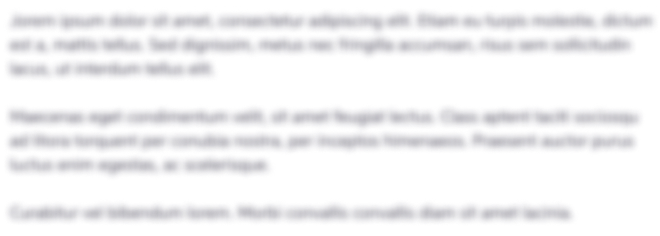
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started