Question
Chapter 13 Lab Advanced GUI Applications Lab Objectives Be able to add a menu to the menu bar Be able to use nested menus Be
Chapter 13 Lab Advanced GUI Applications Lab Objectives Be able to add a menu to the menu bar Be able to use nested menus Be able to add scroll bars, giving the user the option of when they will be seen. Be able to change the look and feel, giving the user the option of which look and feel to use. Introduction In this lab we will be creating a simple note taking interface. It is currently a working program, but we will be adding features to it. The current program displays a window which has one item on the menu bar, Notes, which allows the user 6 choices. These choices allow the user to store and retrieve up to 2 different notes. It also allows the user to clear the text area or exit the program. We would like to add another choice on the menu bar called, giving the user choices about scroll bars and the look and feel of the GUI. The Views has two submenus. One named Look and Feel and one named Scroll Bars. The submenu named Look and Feel lets the user change the look and feels: Metal, Motif, and Windows. The submenu named Scroll Bars offers the user three choices: Never, Always, and As Needed. When the user makes a choice, the scroll bars are displayed according to the choice. Task #1 Creating a Menu with Submenus 1. Copy the file NoteTaker.java to your NetBeans or other Java IDE tools. Compile and run the program. 2. The method createLookAndFeel()is provided (See figure 1). Similarly, write the createScrollBars()method to create a JMenu that has three menu items, Never, Always, and As Needed. See figure 2. 3. Now that we have our submenus, these menus will become menu items for the Views menu. The createViews()method will make the vertical menu shown cascading from the menu choice Views as shown in figure 3. We will add code into the given createViews() method. The call createScrollBars()part is given. You need to add calling createLookAndFeel() inside the createViews()method. 4. Finish creating your menu system by adding the Views menu to the menu bar in the constructor. Task #2 Adding Scroll Bars and Editing the Action Listener 1. Add scroll bars to the text area by completing the following steps in the constructor Create a JScrollPane object called scrolledText, passing in theText. Change the line that adds to the textPanel, by passing in scrolledText (which now has theText). 2. Edit the action listener for menu item Metal, Motif, Window. The Metal is given as a sample. 3. Edit the action listener for menu item Never, Always, and As Needed. The Never is given as a sample. Figure 1
Figure 2
NoteTaker.java:
import javax.swing.*; import java.awt.*; import java.awt.event.*;
public class NoteTaker extends JFrame { //constants for set up of note taking area public static final int WIDTH = 600; public static final int HEIGHT = 300; public static final int LINES = 13; public static final int CHAR_PER_LINE = 45;
//objects in GUI private JTextArea theText; //area to take notes private JMenuBar mBar; //horizontal menu bar private JPanel textPanel; //panel to hold scrolling text area private JMenu notesMenu; //vertical menu with choices for notes //****THESE ITEMS ARE NOT YET USED. YOU WILL BE CREATING THEM IN THIS LAB private JMenu viewMenu; //vertical menu with choices for views private JMenu lafMenu; //vertical menu with look and feel private JMenu sbMenu; //vertical menu with scroll bar option private JScrollPane scrolledText;//scroll bars
//default notes private String note1 = "No Note 1."; private String note2 = "No Note 2.";
/**constructor*/ public NoteTaker() { //create a closeable JFrame with a specific size super("Note Taker"); setSize(WIDTH, HEIGHT); setDefaultCloseOperation (JFrame.EXIT_ON_CLOSE); //get contentPane and set layout of the window Container contentPane = getContentPane(); contentPane.setLayout(new BorderLayout());
//creates the vertical menus createNotes(); createViews(); //creates horizontal menu bar and //adds vertical menus to it mBar = new JMenuBar(); mBar.add(notesMenu); // Add the NotesMenu to the manu bar // Task 1 step 4 // Add the viewMenu to the menu bar here, similar to add notesMenu. setJMenuBar(mBar);
//creates a panel to take notes on textPanel = new JPanel(); textPanel.setBackground(Color.blue); theText = new JTextArea(LINES, CHAR_PER_LINE); theText.setBackground(Color.white); // Task 2 step 1 //****CREATE A JScrollPane object scrolledText like //scrolledText = new JScrollPane(theText); //****CHANGE THE LINE BELOW BY PASSING IN scrolledText, instead of theText textPanel.add(theText); contentPane.add(textPanel, BorderLayout.CENTER); }
/**creates vertical menu associated with Notes menu item on menu bar*/ public void createNotes() { notesMenu = new JMenu("Notes"); JMenuItem item; item = new JMenuItem("Save Note 1"); item.addActionListener(new MenuListener()); notesMenu.add(item);
item = new JMenuItem("Save Note 2"); item.addActionListener(new MenuListener()); notesMenu.add(item);
item = new JMenuItem("Open Note 1"); item.addActionListener(new MenuListener()); notesMenu.add(item);
item = new JMenuItem("Open Note 2"); item.addActionListener(new MenuListener()); notesMenu.add(item);
item = new JMenuItem("Clear"); item.addActionListener(new MenuListener()); notesMenu.add(item);
item = new JMenuItem("Exit"); item.addActionListener(new MenuListener()); notesMenu.add(item); } /**creates vertical menu associated with Views menu item on the menu bar*/ public void createViews() { viewMenu = new JMenu("Views");
createLookAndFeel(); lafMenu.addActionListener(new MenuListener()); viewMenu.add(lafMenu); // Task 1 step 3. // call createScrollBars() method the similar way // of calling createLookAndFeel(); // using sbMenu instead of lafMenu }
/**creates the look and feel submenu */ public void createLookAndFeel() { lafMenu = new JMenu("Look and Feel"); // create Look and feel menu JMenuItem item; item = new JMenuItem("Metal"); // breate a menu item item.addActionListener(new MenuListener()); // add action listener to the item lafMenu.add(item); // add the item to the menu
item = new JMenuItem("Motif"); item.addActionListener(new MenuListener()); lafMenu.add(item);
item = new JMenuItem("Windows"); item.addActionListener(new MenuListener()); lafMenu.add(item); }
/**creates the scroll bars submenu*/ public void createScrollBars() { // Task 1 step 2. // code createScrollBars() method the similar way // of createLookAndFeel(); // using sbMenu instead of lafMenu }
private class MenuListener implements ActionListener { public void actionPerformed(ActionEvent e) { String actionCommand = e.getActionCommand(); if (actionCommand.equals("Save Note 1")) note1 = theText.getText(); else if (actionCommand.equals("Save Note 2")) note2 = theText.getText(); else if (actionCommand.equals("Clear")) theText.setText(""); else if (actionCommand.equals("Open Note 1")) theText.setText(note1); else if (actionCommand.equals("Open Note 2")) theText.setText(note2); else if (actionCommand.equals("Exit")) System.exit(0); //****ADD 6 BRANCHES TO THE ELSE-IF STRUCTURE //****TO ALLOW ACTION TO BE PERFORMED FOR EACH //****MENU ITEM YOU HAVE CREATED else if (actionCommand.equals("Metal")) { try // { UIManager.setLookAndFeel( "javax.swing.plaf.metal.MetalLookAndFeel"); // Any components that have already been created need to be updated SwingUtilities.updateComponentTreeUI( getContentPane()); } catch (Exception except) { System.out.println( "Could not load the Metal look and feel"); } } // Task 2 step 2 - add action for Motif style // by using com.sun.java.swing.plaf.motif.MotifLookAndFeel // add action for Window style // by using com.sun.java.swing.plaf.windows.WindowsLookAndFeel else if (actionCommand.equals("Never")) { scrolledText.setHorizontalScrollBarPolicy( JScrollPane.HORIZONTAL_SCROLLBAR_NEVER); scrolledText.setVerticalScrollBarPolicy( JScrollPane.VERTICAL_SCROLLBAR_NEVER); SwingUtilities.updateComponentTreeUI( getContentPane()); } // Task 2 step 3 - add action for Always, and As Needed // by using constant JScrollPane.HORIZONTAL_SCROLLBAR_ALWAYS and // JScrollPane.VERTICAL_SCROLLBAR_ALWAYS // add action for "As Needed" style // by using JScrollPane.HORIZONTAL_SCROLLBAR_AS_NEEDED // and JScrollPane.VERTICAL_SCROLLBAR_AS_NEEDED else theText.setText("Error in memo interface"); } }
public static void main(String[] args) { NoteTaker gui = new NoteTaker(); gui.setVisible(true); } }
Note Taker Notes Views Look and Feel Metal Scroll Bars Motif Windows LEXStep by Step Solution
There are 3 Steps involved in it
Step: 1
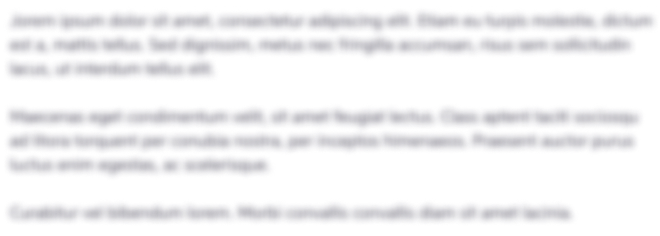
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started