Question
Chapter 7-2 Lab Assignment: Add data validation to the simple calculator. In this exercise, youll add data validation to the Simple Calculator form of the
Chapter 7-2 Lab Assignment: Add data validation to the simple calculator.
In this exercise, youll add data validation to the Simple Calculator form of the Chapter 7-1 Lab Assignment.
1. Code methods named IsPresent, IsDecimal, and IsWithinRange that work like the methods described in chapter 7 of the book (Murach C#2015).
2. Code a method named IsOperator that checks that the text box thats passed to it contains a value of +, -, *, or /. A sample of the error message for this method can be found attached to this project.
3. Code a method named IsValidData that checks that the Operand 1 and Operand 2 text boxes contain a decimal value between 0 and 1,000,000 (non-inclusive) and that the Operator text box contains a valid operator. The attached form shows entries that can be used to test this.
4. Delete all of the catch blocks from the try-catch statement in the btnCalculate_Click event handler except for the one that catches any exception. Then, add code to this event handler that performs the calculation and displays the result only if the values of the text boxes are valid.
5. Add a label to the bottom of the form that contains the text " Programmed By: Your Name". For example if your name is John Doe. The text for this label should read "Programmed by: John Doe".
6. Test the entire application to be sure that all data is validated correctly.
7. When youre done, press the Esc key to end the application.
7-1 Lab Code Included Below -
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms;
namespace SimpleCalculator { public partial class Form1 : Form { public Form1() { InitializeComponent(); }
private void btnCalculate_Click(object sender, System.EventArgs e) { try { decimal operand1 = Convert.ToDecimal(txtOperand1.Text); string operator1 = txtOperator.Text; decimal operand2 = Convert.ToDecimal(txtOperand2.Text); decimal result = Calculate(operand1, operator1, operand2);
result = Math.Round(result, 4); this.txtResult.Text = result.ToString(); txtOperand1.Focus(); } //Handles Format Exception catch (FormatException ex) { MessageBox.Show("Please enter only numbers for operands"); }
//Handles Overflow Exception catch (OverflowException ex) { MessageBox.Show("Overflow Exception Occurred!!!"); }
//Handles DivideByZero Exception catch (DivideByZeroException ex) { MessageBox.Show("In Division, denominator can't be zero"); } //Handles All Exceptions catch (Exception ex) { //Exception Details MessageBox.Show(ex.ToString(), "Error Occured");
decimal operand1 = Convert.ToDecimal(txtOperand1.Text); string operator1 = txtOperator.Text; decimal operand2 = Convert.ToDecimal(txtOperand2.Text); decimal result = Calculate(operand1, operator1, operand2);
result = Math.Round(result, 4); this.txtResult.Text = result.ToString(); txtOperand1.Focus(); } } private decimal Calculate(decimal operand1, string operator1, decimal operand2) { decimal result = 0; if (operator1 == "+") result = operand1 + operand2; else if (operator1 == "-") result = operand1 - operand2; else if (operator1 == "*") result = operand1 * operand2; else if (operator1 == "/") result = operand1 / operand2; return result; }
private void btnExit_Click(object sender, System.EventArgs e) { this.Close(); }
private void ClearResult(object sender, System.EventArgs e) { this.txtResult.Text = ""; } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
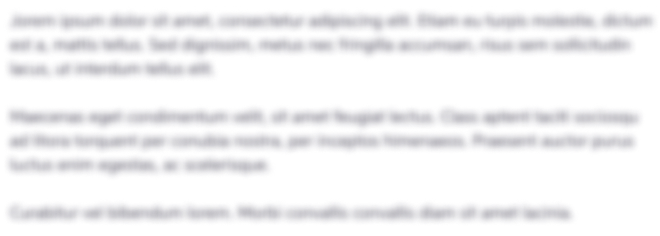
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started