Question
Chapter 9 Exercise 12, Introduction to Java Programming, Tenth Edition Y. Daniel LiangY. 9.12 (Geometry: intersecting point) Suppose two line segments intersect. The two end-points
Chapter 9 Exercise 12, Introduction to Java Programming, Tenth Edition Y. Daniel LiangY.
9.12 (Geometry: intersecting point) Suppose two line segments intersect. The two end-points for the first line segment are (x1, y1) and (x2, y2) and for the second line segment are (x3, y3) and (x4, y4). Write a program that prompts the user to enter these four endpoints and displays the intersecting point. As discussed in Program- ming Exercise 3.25, the intersecting point can be found by solving a linear equation. Use the LinearEquation class in Programming Exercise 9.11 this is the code from 9.11
import java.util.Scanner;
public class LinearEquationTest {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter value of a, b, c, d, e and f: ");
int a = sc.nextInt(); int b = sc.nextInt(); int c = sc.nextInt(); int d = sc.nextInt(); int e = sc.nextInt(); int f = sc.nextInt(); LinearEquation eqution = new LinearEquation(a, b, c, d, e, f); System.out.println("isSolavable: "+eqution.isSolavable()); System.out.println("X: "+eqution.getX()); System.out.println("Y: "+eqution.getY()); } }
/* Sample run: Enter value of a, b, c, d, e and f:
1 2 3 4 5 6 isSolavable: true X: -4.0 Y: 4.5 */ -------------
and
public class LinearEquation {
// instance variables
private int a, b, c, d, e, f;
// constructor
public LinearEquation(int a, int b, int c, int d, int e, int f) {
this.a = a; this.b = b; this.c = c; this.d = d; this.e = e; this.f = f;
}
// getters
public int getA() {
return a;
}
public int getB() {
return b;
}
public int getC() {
return c;
}
public int getD() {
return d;
}
public int getE() {
return e;
}
public int getF() {
return f;
}
// other functions
public boolean isSolavable(){
if((a*d - b*c) != 0)
return true;
else
return false;
}
public double getX(){
if(isSolavable())
return (double)(e*d - b*f)/(double)(a*d - b*c);
else
return -999;
}
public double getY(){
if(isSolavable())
return (double)(a*f - e*c)/(double)(a*d - b*c);
else
return -999;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
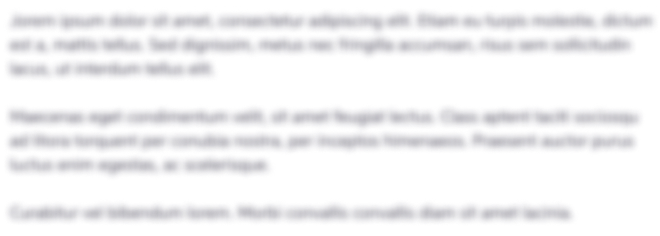
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started