Question
Check if first CharSet has the same elements as second CharSet(equals): return true if two sets have the same exact elements; otherwise return false Check
Check if first CharSet has the same elements as second CharSet(equals): return true if two sets have the same exact elements; otherwise return false
Check if first CharSet is a subset of second CharSet(subset): return true if all elements of first set are in second set; otherwise return false
Check if first CharSet if a superset of second CharSet(superset) : return true if all elements of second set are in first set; otherwise return false
Clear all elements from the CharSet(clear): remove all elements
public class Charset {
private int asciiSet[] = new int [128];
public Charset() {
//by default all values in array are 0
}
public Charset(char c) {
insert(c);
}
public Charset(String str) {
for(int i=0;i < str.length();i++) {
insert(str.charAt(i));
}
}
public Charset union(Charset other)
{
Charset result = new Charset();
for(int i = 0; i < 128; i++)
{
if(asciiSet[i] == 1 || other.asciiSet[i] == 1)
result.asciiSet[i] = 1;
}
return result;
}
public Charset intersection(Charset other)
{
Charset result = new Charset();
for(int i = 0; i < 128; i++)
{
if(asciiSet[i] == 1 && other.asciiSet[i] == 1)
result.asciiSet[i] = 1;
}
return result;
}
public boolean insert(char c)
{
if(c >= 32 && c < 128)
{
asciiSet[c] = 1;
return true;
}
else
return false;
}
public boolean remove(char c)
{
if(c >= 32 && c < 128)
{
asciiSet[c] = 0;
return true;
}
else
return false;
}
public boolean isElement(char c)
{
if(c >= 32 && c < 128 && asciiSet[c] == 1)
{
return true;
}
else
return false;
}
public int size()
{
int total = 0;
for(int i = 0; i < 128; i++)
{
if(asciiSet[i] == 1)
total++;
}
return total;
}
public String toString()
{
String str = "{";
for(int c = 32; c < 128; c++)
{
if(asciiSet[c] == 1)
str += (char)c;
}
str += "}";
return str;
}
public Charset clone()
{
Charset copy = new Charset();
for(int i = 0; i < 128; i++)
copy.asciiSet[i] = asciiSet[i];
return copy;
}
/*
public void printSet() {
System.out.println("*******************");
System.out.println("Index:\tValue");
for(int i=0;i System.out.println(i+"\t"+asciiSet[i]); System.out.println("*******************"); }*/ public static void main(String[] args) { Charset set1 = new Charset(); Charset set2= new Charset('A'); Charset set3 = new Charset("Laptop"); Charset set4 = new Charset("Monitor"); System.out.println("set1 : size = " + set1.size() + " " + set1); System.out.println("set2 : size = " + set2.size() + " " + set2); System.out.println("set3 : size = " + set3.size() + " " + set3); System.out.println("set4 : size = " + set4.size() + " " + set4); Charset union = set3.union(set4); Charset inter = set4.intersection(set3); System.out.println("Union of set3 and set 4 is " + union); System.out.println("Intersection of set3 and set 4 is " + inter); System.out.println("Removing 'M' from set4"); set4.remove('M'); System.out.println("set4: " + set4); System.out.println("Inserting 'z' into set4"); set4.insert('z'); System.out.println("set4: " + set4); System.out.println("Cloning set3 "); Charset copyset3 = set3.clone(); System.out.println("Copy of set3 : " + copyset3); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
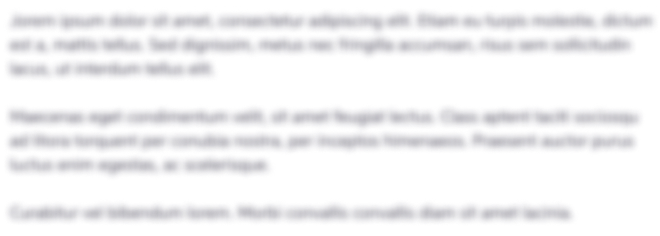
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started