Question
Checkpoint 1 Add another pointer variable to the struct node data-structure as follows: struct node *prev; Check at: https://www.thecodingdelight.com/doubly-linked-list/ It illustrates the different operations that
Checkpoint 1
Add another pointer variable to the struct node data-structure as follows: struct node *prev;
Check at: https://www.thecodingdelight.com/doubly-linked-list/ It illustrates the different operations that we want to implement into our doubly linked list and also provides working code in Java, JavaScript and C++ where the C++ will be most useful to implement the different functions in this lab.
Checkpoint 2 Implement the following functions:
(i) insert(int num): Insert num to the front/back of the linked list.
(ii) Display the doubly linked list.
Checkpoint 3 Implement the following functions:
insertAfter(int num, int index): Insert num after item at a particular index.
Checkpoint 4
remove(int num): Search from the start of the list for the passed in num and remove it from the list, if found.
remove(int index): Remove num at a specific index.
Checkpoint 5
Do we have to update the count() function to work with the doubly linked list?
Code:
#include
#include
struct node
{
int data;
struct node *next;
}*head;
void append(int num)
{
struct node *temp,*right;
temp= (struct node *)malloc(sizeof(struct node));
temp->data=num;
right=(struct node *)head;
if(right==NULL)
{
add(num)
}
else
{
while(right->next != NULL)
right=right->next;
right->next =temp;
right=temp;
right->next=NULL;
}
}
void add( int num )
{
struct node *temp;
temp=(struct node *)malloc(sizeof(struct node));
temp->data=num;
if (head== NULL)
{
head=temp;
head->next=NULL;
}
else
{
temp->next=head;
head=temp;
}
}
void addafter(int num, int loc)
{
int i;
struct node *temp,*left,*right;
right=head;
for(i=1;i { left=right; right=right->next; } temp=(struct node *)malloc(sizeof(struct node)); temp->data=num; left->next=temp; left=temp; left->next=right; return; } void insert(int num) { int c=0; struct node *temp; temp=head; if(temp==NULL) { add(num); } else { while(temp!=NULL) { if(temp->data return; if(temp->data c++; temp=temp->next; } if(c==0) add(num); else if(c addafter(num,++c); else append(num); } } int delete(int num) { struct node *temp, *prev; temp=head; while(temp!=NULL) { if(temp->data==num) { if(temp==head) { head=temp->next; free(temp); return 1; } else { prev->next=temp->next; free(temp); return 1; } } else { prev=temp; temp= temp->next; } } return 0; } void display(struct node *r) { r=head; if(r==NULL) { return; } while(r!=NULL) { printf("%d ",r->data); r=r->next; } printf(" "); } int count() { struct node *n; int c=0; n=head; while(n!=NULL) { n=n->next; c++; } return c; } int main() { int i,num; struct node *n; head=NULL; while(1) { printf(" List Operations "); printf("=============== "); printf("1.Insert "); printf("2.Display "); printf("3.Size "); printf("4.Delete "); printf("5.Exit "); printf("Enter your choice : "); if(scanf("%d",&i)<=0){ printf("Enter only an Integer "); exit(0); } else { switch(i) { case 1: printf("Enter the number to insert : "); scanf("%d",&num); // insert(num); append(num); break; case 2: if(head==NULL) { printf("List is Empty "); } else { printf("Element(s) in the list are : "); } display(n); break; case 3: printf("Size of the list is %d ",count()); break; case 4: if(head==NULL) printf("List is Empty "); else{ printf("Enter the number to delete : "); scanf("%d",&num); if(delete(num)) printf("%d deleted successfully ",num); else printf("%d not found in the list ",num); } break; case 5: return 0; default: printf("Invalid option "); } } } return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
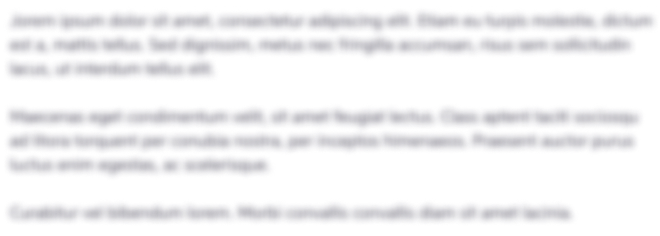
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started