Question
C#-I need someone to help me with this problem: For today's lab you will be creating a program that will act as basic employee tracking
C#-I need someone to help me with this problem:
For today's lab you will be creating a program that will act as basic employee tracking software. The program will consist of multiple connected classes. Employee will serve as a base class that contains fields for name and address. Hourly will inherit from employee and contains decimal fields for payPerHour and hoursPerWeek. FullTime will inherit from Hourly. PartTime will inherit from Hourly. Contractor will inherit from hourly and contains a decimal field for noBenefitsBonus. Salaried will inherit from Employee and contains a decimal field for salary. Manager will inherit from Salaried with a decimal field for bonus.
Main will contain a list of employees and present a menu to the user that offers the following options: Add employee - lets the user to choose to create an employee of type (FullTime, PartTime, Contractor, Salaried, or Manager) prompts the user for the appropriate values, and adds them to the list. Remove Employee - Allows the user to select an existing employee and remove her/him from the list, Display Payroll - displays all of the employees (1 per line, using properties to access name / address from employee and the CalculatePay method for the employees yearly pay), Exit - allows the user to exit the program. The program will continue running until the user chooses to exit.
Input must be validated. The user must not be able to crash your program with invalid input. For all methods the user should be able to make a selection either by number 1,2,3 or by typing out the option add employee. String values should be case insensitive so that AdD EMPloyEE works just like add employee.
Use the following guidelines to complete this application:
Classes
Create a class called Employee
Contains the following fields
name of type string
address of type string
Implements IComparable interface
Create a class called Hourly
Inherits from Employee
Contains the following fields
payPerHour of type decimal
hoursPerWeek of type decimal
Create a class called FullTime
Inherits from Hourly
Create a class called PartTime
Inherits from Hourly
Create a class called Contractor
Inherits from Hourly
Contains the following fields
noBenefitsBonus of type decimal - contractors tend to be paid 10 to 15 % more than normal employees because they do not receive benefits.
Create a class called Salaried
Inherits from Employee
Contains the following fields
salary of type decimal - salaried employees get a lump sum each year no matter the number of hours worked.
Create a class called Manager
Inherits from Salaried
Contains the following fields
bonus of type decimal - managers are salaried but usually receive a yearly bonus in addition to their normal pay.
Constructors
Employee needs a constructor that takes parameters for name and address
Hourly needs a constructor that takes parameters for name, address, payPerHour, and hoursPerWeek.
Name and address need to be passed to the Hourlys parent constructor.
FullTime needs a constructor that takes parameters for name, address, and payPerHour.
Name, address, payPerHour, and a constant 40 for hours need to be passed to FullTimes parent constructor.
PartTime needs a constructor that takes parameters for name, address, payPerHour, and HoursPerWeek.
All of the parameter values need to be passed to PartTimes parent constructor.
Contractor needs a constructor that takes parameter for name, address, payPerHour, hoursPerWeek, and noBenefitsBonus.
All of the parameters except noBenefitsBonus need to be passed to Contractors parent constructor.
Salaried needs a constructor that takes name, address, and salary
Name and address need to be passed to Salarieds parent constructor.
Manager needs a constructor that takes name, address, salary, and bonus.
Name, address, and salary need to be passed to Managers parent constructor.
Menu Options
The menu must have the following options:
Add Employee - this option presents the user with the option of creating an employee of the following types: FullTime, PartTime, Contractor, Salaried, and Manager and adds it to the list. The last thing this option should do is call .Sort() on the list of employees.
Remove Employee - this option presents the user with a list of all employees in the list and allows the user to select 1 to remove.
Display Payroll - this option displays all of the employees in the list showing name, address, and yearly pay (Calculated using CalculatePay method).
Exit - stop the program.
Other Items
At the top of main you will need to create a list of Employees
Employee must implement the IComparable interface (This interface already exists like the Console class. You do not have to create it. Just declare that Employee implements it and then implement the required CompareTo method).
CompareTo method implemented to sort employees by name
CalculatePay
Employee must contain a virtual method CalculatePay
Other classes must override CalculatePay so that it returns the correct value for what that employee would make in a year.
Contractor - income is base amount + base amount * noBenefitBonus
Manager - income is base amount + bonus
Input Validation
Input must be validated
Step by Step Solution
There are 3 Steps involved in it
Step: 1
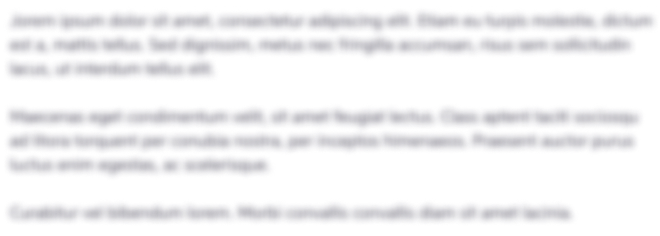
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started