Question
CIS 1068 Assignment 5 practice with static methods and strings Due: February 24 60 points description Please download this zipped Eclipse project. Unzip the project,
CIS 1068 Assignment 5 practice with static methods and strings
Due: February 24
60 points
description
Please download this zipped Eclipse project. Unzip the project, and import it into Eclipse by choosing File -> Import -> Existing Projects into Workspace. Open the project and its included source file StringPractice.java. You'll find that it contains several methods that have not yet been finished. For now, the methods contain only a placeholder return value so that the code will compile. Fill in your own implementation. Do not modify any other files in the project.
You do not have to write a main() to test your code. Though it may not be obvious, all of the test code has been written for you. If you're curious, you can find it by looking at the file StringPracticeTest.java included in the same directory as the code you need to complete. These tests use the popular JUnit testing framework. For now, you are not required to understand how they work. If you're curious, by all means look, but please do not modify the contents of this file.
In order to test your methods using the provided tests, click on the StringPracticeTest.java file, and the click the run button. Initially, you'll see a red bar, indicating that not all of the tests have passed. Each of your functions whose tests did not pass will have an X through its name. Eventually, you'll have perfectly debugged code, and the bar will turn green.
It's highly recommended that you use short helper methods to help program other methods. For example, you might use your isFace() method in the more complicated methods involving "face cards". Similarly, you might consider using the reversed() method from this assignment in your your sameInReverse() method.
******************************************
package cis1068.stringPracticeAssignment;
import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertFalse; import static org.junit.Assert.assertTrue; import org.junit.Test;
public class StringPracticeTest extends StringPractice {
@Test public void testIsFace() { assertTrue(StringPractice.isFace('a')); assertTrue(StringPractice.isFace('A')); assertTrue(StringPractice.isFace('k')); assertTrue(StringPractice.isFace('K')); assertTrue(StringPractice.isFace('q')); assertTrue(StringPractice.isFace('A')); assertTrue(StringPractice.isFace('j')); assertTrue(StringPractice.isFace('J')); assertFalse(StringPractice.isFace('f')); assertFalse(StringPractice.isFace('G')); assertFalse(StringPractice.isFace('0')); }
@Test public void testIndexOfFirstFaceString() { assertEquals(-1, StringPractice.indexOfFirstFace("")); assertEquals(0, StringPractice.indexOfFirstFace("abalone")); assertEquals(6, StringPractice.indexOfFirstFace("trepidation")); assertEquals(3, StringPractice.indexOfFirstFace("workbook")); }
@Test public void testIndexOfFirstFaceStringInt() { assertEquals(1, StringPractice.indexOfFirstFace("squidward")); assertEquals(1, StringPractice.indexOfFirstFace("SQUIDWARD")); assertEquals(2, StringPractice.indexOfFirstFace("vikinglike")); assertEquals(2, StringPractice.indexOfFirstFace("VIKINGLIKE")); assertEquals(0, StringPractice.indexOfFirstFace("kafkaesque")); assertEquals(0, StringPractice.indexOfFirstFace("KAFKAESQUE")); assertEquals(-1, StringPractice.indexOfFirstFace("homer")); }
@Test public void testIndexOfLastFace() { assertEquals(6, StringPractice.indexOfLastFace("squidward")); assertEquals(6, StringPractice.indexOfLastFace("SQUIDWARD")); assertEquals(8, StringPractice.indexOfLastFace("vikinglike")); assertEquals(8, StringPractice.indexOfLastFace("VIKINGLIKE")); assertEquals(7, StringPractice.indexOfLastFace("kafkaesque")); assertEquals(7, StringPractice.indexOfLastFace("KAFKAESQUE")); assertEquals(-1, StringPractice.indexOfLastFace("homer")); }
@Test public void testReversed() { assertEquals("cba", StringPractice.reversed("abc")); assertEquals("a", StringPractice.reversed("a")); assertEquals("dcba", StringPractice.reversed("abcd")); }
@Test public void testNumOccurrences() { assertEquals(2, StringPractice.numOccurrences("banana", "na")); assertEquals(2, StringPractice.numOccurrences("mississippi", "ss")); assertEquals(0, StringPractice.numOccurrences("undertow", "sushi")); }
@Test public void testSameInReverse() { assertTrue(StringPractice.sameInReverse("peep")); assertTrue(StringPractice.sameInReverse("madam")); assertTrue(StringPractice.sameInReverse("rotator")); assertFalse(StringPractice.sameInReverse("blue")); assertFalse(StringPractice.sameInReverse("aspirin")); assertFalse(StringPractice.sameInReverse("ab")); assertTrue(StringPractice.sameInReverse("a")); }
@Test public void testWithoutFaces() { assertEquals("suidwrd", StringPractice.withoutFaces("squidward")); assertEquals("SUIDWRD", StringPractice.withoutFaces("SQUIDWARD")); assertEquals("viinglie", StringPractice.withoutFaces("vikinglike")); assertEquals("VIINGLIE", StringPractice.withoutFaces("VIKINGLIKE")); assertEquals("fesue", StringPractice.withoutFaces("kafkaesque")); assertEquals("FESUE", StringPractice.withoutFaces("KAFKAESQUE")); assertEquals("homer", StringPractice.withoutFaces("homer")); }
@Test public void testContainsOnlyFaces() { assertTrue(StringPractice.containsOnlyFaces("kQjj")); assertTrue(StringPractice.containsOnlyFaces("JAK")); assertFalse(StringPractice.containsOnlyFaces("spring break")); assertFalse(StringPractice.containsOnlyFaces("temple")); }
@Test public void testContainsNoFaces() { assertTrue(StringPractice.containsNoFaces("beguile")); assertTrue(StringPractice.containsNoFaces("chess")); assertTrue(StringPractice.containsNoFaces("cider")); assertTrue(StringPractice.containsNoFaces("unsupported")); assertTrue(StringPractice.containsNoFaces("visitor")); assertFalse(StringPractice.containsNoFaces("viking")); assertFalse(StringPractice.containsNoFaces("ajax")); }
@Test public void testLastFirst() { assertEquals("Sqigmann, Andrew", StringPractice.lastFirst("Andrew Sqigmann")); assertEquals("Squarepants, Spongebob", StringPractice.lastFirst("Spongebob Squarepants")); assertEquals("Pines, Stanley", StringPractice.lastFirst("Stanley Pines")); } }
****************************************************
package cis1068.stringPracticeAssignment;
public class StringPractice { /* * returns true if c is one of the characters typically used to represent a "face card" in a * standard deck of playing cards. * * These include: jack 'J' or 'j' queen 'Q' or 'q' king 'K' or 'k' ace 'A' or 'a */ public static boolean isFace(char c) { /* * placeholder just so that the function compiles. fill in your implementation here. * * there is a similar placeholder for each of the remaining functions */ return true; }
/* * returns the index of the first face-card letter in s or -1 if s contains no face-card letters */ public static int indexOfFirstFace(String s) { return -1; }
/* * returns the index of the first occurrence of a face-card letter in s starting from index * startPosition or -1 if there are none at index startPosition or later. Notice that this method * has the same name as the previous one, but that it takes a different number of arguments. This * is perfectly legal in Java. It's called "method overloading" */ public static int indexOfFirstFace(String s, int startPosition) { return -1; }
/* * returns the index of the last occurrence of a face-card letter in s or -1 if s contains none */ public static int indexOfLastFace(String s) { return -1; }
/* * returns s in reverse. For example, if s is "Apple", the method returns the String "elppA" */ public static String reversed(String s) { return ""; }
/* * returns the number of times that n occurs in h. For example, if h is "Mississippi" and n is * "ss" the method returns 2. */ public static int numOccurrences(String h, String n) { return 0; }
/* * returns true if s is the same backwards and forwards and false otherwise */ public static boolean sameInReverse(String s) { return true; }
/* * returns a new String which is the same as s, but with all of the face-card letters removed. */ public static String withoutFaces(String s) { return ""; }
/* * returns true if s consists only of face-card letters or false otherwise */ public static boolean containsOnlyFaces(String s) { return true; }
/* * returns true if s contains no face-card letters or false otherwise */ public static boolean containsNoFaces(String s) { return true; }
/* * Passed a reference to a person's name in the form FIRST_NAME LAST_NAME. The method returns a * reference to a new String in the form LAST_NAME, FIRST_NAME. * * For example, if we were passed "Spongebob Squarepants", we'd return "Squarepants, Spongebob". * You may assume that the method is passed exactly two words separated by a single space. */ public static String lastFirst(String s) { return " " ; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
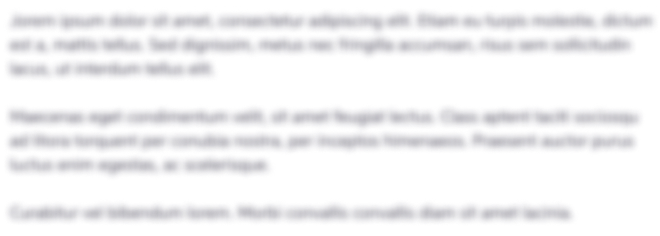
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started