Question
class BigShopper is a subclass of Shopper (20 points) note: all the abstract methods in Shopper and in the interface ShoppingTimeRemaing are implemented here Note:
class BigShopper is a subclass of Shopper (20 points) note: all the abstract methods in Shopper and in the interface ShoppingTimeRemaing are implemented here
Note: You dontr\ say implement in the class header since you this is done in Shopper
Note: Items in red are different From FastShopper
It has a public static int variable bigShopperCounter set to 0
It has a public static int variable TIMEWITHCHECKER = 2
It has a private String instance variable bigShopperID - note: has a simple accessor method
It has the following additional private instance int variables, each of which has a simple accessor method that returns its current value. Those with an * also have a simple mutator method
- startTime*
- timeShopping
- shoppingTimeRemaining*
- timeIntoCheckoutLine*
- timeOutOfCheckoutLine
- endTime calculated in calculateFinalDurations method
- totalTimeCheckingOut - calculated in calculateFinalDurations method
- totalTimeInStore - calculated in calculateFinalDurations method
It also has a Random reference variable randy (dont forget the proper import statement)
There is one constructor with one int input for the startTime.
- It calls the super constructor with the String BigShopper
- It creates a Random object that randy refer to using as the seed the bigShopperCounter
- It calls setBigShopperID() with no parameters
- It calls setStartTime with the startTime as the parameter
- It calls setTimeShopping with no parameters
- It calls setShoppingTimeRemaining with the timeShopping (Must be done last)
The following methods has special code used in the mutator method.
setBigShopperID has no parameters and a void return.
It increments bigShopperCounter by 1 sets bigShopperID equal to the concatenation of the return from getShopperType() and bigShopperCounter
setTimeShopping has no parameters and a void return
It sets the timeShopping variable to a value between 5 and 15 inclusively using the randy Random object
setTimeOutOfCheckoutLine has an int input parameter and a void return
It sets timeOutOfCheckoutLine to the input parameter value
It calls calculateFinalDurations with no parameters
There are two additional methods.
calculateFinalDurations has no parameters and a void return and sets the following values:
endTime equals the sum of timeOutOfCheckoutLine and TIMEWITHCHECKER
totalTimeCheckingOut is the positive difference of the endTime and timeIntoCheckoutLine
totalTimeInStore is the positive difference between the endTime and the startTime;
There is also a toString method that returns a formatted String for printing as shown in the output.
It includes the following values: bigShopperID, startTime, endTime, timeShopping, totalTimeCheckingOut, totalTimeInStore
class Supermarket (80 points)
Determine and use the necessary import statements
The following instance variables are used
- A String superName with a standard accessor and standard mutator
- A Random variable of type randy An int bigCheckerOccupied set to 0
- An int fastCheckerOccupied set to 0
- The following ArrayLists, each of which can be instantiated when declared
~ An ArrayList of type Shopper called currentShoppers
~ An ArrayList of type BigShopper called bigCheckOut
~ An ArrayList of type FastShopper called fastCheckOut
~ An ArrayList of type Shopper called doneShopping
Supermarket has a single constructor that accepts a String value and an int value
It calls setSuperName with that value
It uses the int value as a seed to initialize randy
Method openSupermarket with no parameters and a void return
Create a loop with i going from 0 to less than 14 inclusive
if i is divisible by 3
Create a new BigShopper instantiated with startTime equal to 0
Add the BigShopper to the currentShoppers ArrayList
else
Create a new FastShopper instantiated with startTime equal to 0
Add the FastShopper to the currentShoppers ArrayList
Method operateSupermarket with an int parameter with the minutes to run the simulation and a void return
Set an int minCounter with the current minute equal to 1
While (currentShoppers is not empty or bigCheckOut is not empty or fastCheckout is not empty
//This section lets more shoppers in
If the minCounter <=minutes and minCounter is divisible by 5
Create a loop with i going from 0 to less than 9
if i is divisible by 3
Create a new BigShopper instantiated with startTime equal to minCounter
Add the BigShopper to the currentShoppers ArrayList
else
Create a new FastShopper instantiated with startTime equal to minCounter
Add the FastShopper to the currentShoppers ArrayList
end loop
End the If
/This section processes the Shoppers
For i = 0 to i < number of Shoppers in currentShoppers
Get the iTH Shopper on the currentShoppers ArrayList (create a tempShopper variable)
Use the appropriate methods to retrieve shoppingTimeRemaining for tempShopper and decrement by 1
if the shoppingTimeRemaining for the tempShopper now equals 0 if the tempShopper is a BigShopper
Create a casted BigShopper reference variable from the tempShopper variable (call it tempBig)
Set the timeIntoCheckoutLine for tempBig to the minCounter
Add tempBig to bigCheckOut
else
Create a casted FastShopper reference variable from the
tempShopper variable (call it tempFast)
Set the timeIntoCheckoutLine for tempFast to the minCounter
Add tempFast to fastCheckOut
End if else
Remove the tempShopper from currentShoppers
Decrement i by 1
End the outer if
End Of the For Loop
//process the Big Checkout (You are still in the operateSupermarket method
if bigCheckOut is not empty and bigCheckerOccupied == 0
Remove the shopper from the 0TH position of bigCheckOut and create a
temporary reference variable tempBig for it
Set the timeOutOfCheckoutLine tempBig to the minCounter
Add the tempBig BigShopper to the doneShopping ArrayList
Set bigCheckerOccupied to BigShopper.TIMEWITHCHECKER
else if bigCheckerOccupied doesnt equal 0
Decrement bigCheckerOccupied by 1
if fastCheckOut is not empty and fastCheckerOccupied == 0
Remove the shopper from the 0TH position of fastCheckOut and create a
temporary reference variable tempFast for it
Set the timeOutOfCheckoutLine for tempFast to the minCounter
Add the tempFast FastShopper to the doneShopping ArrayList
Set fastCheckerOccupied to FastShopper.TIMEWITHCHECKER
else if fastCheckerOccupied doesnt equal 0
Decrement fastCheckerOccupied by 1
Increment the minCounter
End Of The while loop
End of the operateSupermarket method
Method generateSupermarketResults accepts a String with the name of the Physical file (remember what it throws)
Create a PrintWriter object based on the input parameter
Create the output headers as per the sample output
Create the necessary counter variables set to 0
Create an Iterator for the doneShopping ArrayList
Use the Iterator to go through the items,
Print each item using toString
Collecting the data necessary to separately be able to figure out the average time a
BigShopper or a FastShopper waits on line and checks out based on the times returned
with the getTotalTimeCheckingOut() method
Print out the two averages as shown in the output file
Close the PrintWriter object
class ModelSupermarket (10 points)
This class consists of only the main method which could have a throws clause
Remember to make any necessary import statements
Create a Scanner for interactive input
Request and read in the name of the supermarket (use nextLine)
Request and read in an int seed value for use by the Supermarket
Create a new Supermarket with the name and seed as input parameters
Request and read in the name of the output file (use next)
Request and read in the number of minutes to operate the Supermarket
Call openSupermarket for the created Supermarket
Call operateSupermarket with the number of minutes to operate the Supermarket for the created Supermarket
Call generateSupermarketResults with the name of the output file for the created Supermarket
Step by Step Solution
There are 3 Steps involved in it
Step: 1
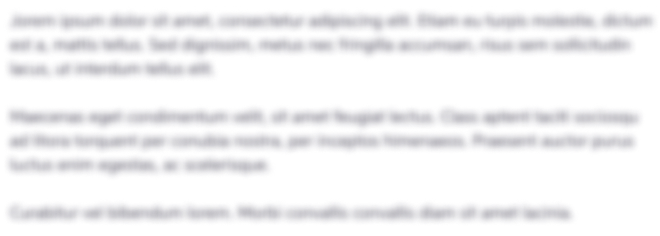
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started