Question
class IntList { private: struct Node { int data; Node* next; }; Node* head; unsigned int size; public: class iterator { int& operator*() const; iterator&
class IntList {
private:
struct Node {
int data;
Node* next;
};
Node* head;
unsigned int size;
public:
class iterator {
int& operator*() const;
iterator& operator++();
};
class const_iterator {
const int& operator*() const;
const_iterator& operator++();
};
// returns an iterator associated with the first element in the list
iterator begin();
// returns an iterator associated with the last element in the list
iterator end();
// returns the last element in the list
int pop_back();
// returns the first element in the list
int pop_front();
// adds x to the end of the list
void push_back(const int& x);
// adds x to the beginning of the list
void push_front(const int& x);
// returns the number of elements in the list
unsigned int size();
};
Below is the question
Write the code for the function getIntersection below, that given two sorted linked lists returns a
linked list that is an intersection of them. Assume that these linked lists are implemented by the
class IntList with the interface from Problem 2.
IntList& getIntersection(IntList& intList1, IntList& intList2) {
// TODO: you code here
}
Estimate the big-O complexity of your implementation.
I HAVE INCLUDED CLASS INTLIST ABOVE.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
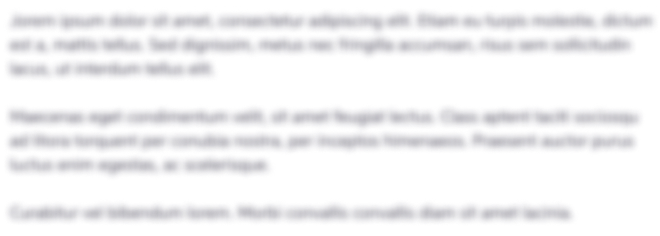
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started