Question
CLASS Main import java.io.*; import java.util.*; import javax.swing.*; // Main class class Main { // The main method of the whole program, allows the name
CLASS Main import java.io.*; import java.util.*; import javax.swing.*; // Main class class Main { // The main method of the whole program, allows the name of the scene definition file to be input on the // command line or selected using the file chooser dialog window. It calls the parser to parse the scene // definition file and add the graphic objects to the scene. public static void main(String[] args) { Scanner stdin = new Scanner(System.in); String sceneFileName; File sceneFile; Scene scene; JFileChooser choice = new JFileChooser(new File(".")); int option = choice.showOpenDialog(null); if (option == JFileChooser.APPROVE_OPTION) sceneFile = choice.getSelectedFile(); else { System.out.print("Enter scene file name or a single space to choose file from window: "); sceneFileName = stdin.nextLine(); sceneFile = new File(sceneFileName);} try { Parser parser = new Parser(sceneFile); scene = parser.parseScene(); scene.draw(); } catch (SyntaxError error) { System.out.println(error.getMessage()); } catch (LexicalError error) { System.out.println(error.getMessage()); } catch (IOException error) { System.out.println("IO Error"); } } }
CLASS Parser import java.awt.*; import java.io.*; import java.util.*; import javax.swing.*; // This class provides the skeleton parser class Parser { private JFrame window; private Token token; private Lexer lexer; // Constructor to create new lexical analyzer from input file public Parser(File file) throws IOException { lexer = new Lexer(file); } // Parses the production // scene -> SCENE IDENTIFIER number_list images END '.' public Scene parseScene() throws LexicalError, SyntaxError, IOException { verifyNextToken(Token.SCENE); verifyNextToken(Token.IDENTIFIER); String window = lexer.getLexeme(); int[] dimensions = getNumberList(2); Scene scene = new Scene(window, dimensions[0], dimensions[1]); parseImages(scene, lexer.getNextToken()); verifyNextToken(Token.PERIOD); return scene; }// Parses the following productions // images -> image images | image // image -> right_triangle | rectangle // right_triangle -> RIGHT_TRIANGLE COLOR number_list AT number_list HEIGHT NUMBER WIDTH NUMBER ';' // rectangle -> RECTANGLE_ COLOR number_list AT number_list HEIGHT NUMBER WIDTH NUMBER ';' private void parseImages(Scene scene, Token imageToken) throws LexicalError, SyntaxError, IOException { int height, width, offset, radius; verifyNextToken(Token.COLOR); int[] colors = getNumberList(3); Color color = new Color(colors[0], colors[1], colors[2]); verifyNextToken(Token.AT); int[] location = getNumberList(2); Point point = new Point(location[0], location[1]); if (imageToken == Token.RIGHT_TRIANGLE) { verifyNextToken(Token.HEIGHT); verifyNextToken(Token.NUMBER); height = lexer.getNumber(); verifyNextToken(Token.WIDTH); verifyNextToken(Token.NUMBER); width = lexer.getNumber(); RightTriangle triangle = new RightTriangle(color, point, height, width); scene.addImage(triangle); } else if (imageToken == Token.RECTANGLE) { verifyNextToken(Token.HEIGHT); verifyNextToken(Token.NUMBER); height = lexer.getNumber(); verifyNextToken(Token.WIDTH); verifyNextToken(Token.NUMBER); width = lexer.getNumber(); Rectangle rectangle = new Rectangle(color, point, height, width); scene.addImage(rectangle); } else { throw new SyntaxError(lexer.getLineNo(), "Unexpected image name " + imageToken); } verifyNextToken(Token.SEMICOLON); token = lexer.getNextToken(); if (token != Token.END) parseImages(scene, token); }// Parses the following productions // number_list -> '(' numbers ')' // numbers -> NUMBER | NUMBER ',' numbers // Returns an array of the numbers in the number list private int[] getNumberList(int count) throws LexicalError, SyntaxError, IOException { int[] list = new int[count]; verifyNextToken(Token.LEFT_PAREN); for (int i = 0; i list = new ArrayList CLASS Polygon_ import java.awt.*; // Base class for all polygon classes class Polygon_ extends Image { private int vertexCount; private Polygon polygon; // Constructor that initializes color and vertexCount of a polygon public Polygon_(Color color, int vertexCount) { super(color); this.vertexCount = vertexCount; } // Creates a polygon object given its vertices public void createPolygon(int[] x_points, int[] y_points) { polygon = new Polygon(x_points, y_points, vertexCount); } // Draws polygon using polygon object @Override public void draw(Graphics graphics) { colorDrawing(graphics); graphics.drawPolygon(polygon); } } CLASS Rectangle import java.awt.*; // Class that defines a hollow rectangle object class Rectangle extends HollowPolygon { // Constructor that initializes the vertices of the rectanglepublic Rectangle(Color color, Point upperLeft, int height, int width) { super(color, 4); int[] x_points = {upperLeft.x, upperLeft.x + width, upperLeft.x + width, upperLeft.x}; int[] y_points = {upperLeft.y, upperLeft.y, upperLeft.y + height, upperLeft.y + height}; createPolygon(x_points, y_points); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
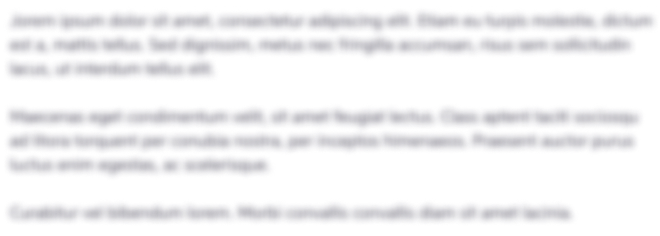
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started