Question
class Monster: # Creating class of monster def __init__(self, name , hp=20) -> None: # Constructor of class self.exp = 0 self.name = name self.hp
class Monster: # Creating class of monster
def __init__(self, name , hp=20) -> None: # Constructor of class self.exp = 0 self.name = name self.hp = hp self.max_hp = hp self.attacks = { 'wait' : 0 } def win_fight(self, ): # Win_fight method self.exp += 5 self.hp = self.max_hp def lose_fight(self, ): # lose_fight method self.exp += 1 self.hp = self.max_hp
def add_attack(self, attack_name, attack_point): # method to add attacks in the monster self.attacks[attack_name] = attack_point
def remove_attack(self, attack_name): # method to remove attack from the monster del self.attacks[attack_name]
def monster_fight(monster1 : Monster, monster2 : Monster): # Method to make two monster fight
# Checking for edge case if list(monster1.attacks.keys()) == ['wait'] and list(monster2.attacks.keys() == ['wait']): # This condition means edge case is detected and no fight will take place return -1, None, None #Declaring variables. round_number = 0 # List of name of attacks of monster sorted by their power m1Attacks = sorted( monster1.attacks.keys(), key=lambda x: monster1.attacks[x]) m2Attacks = sorted( monster2.attacks.keys(), key=lambda x: monster2.attacks[x]) # Index to keep track of which attack will be used m1Index = 0 m2Index = 0 # Max index limit m1Max = len(m1Attacks) m2Max = len(m2Attacks) # List to store attacks of fight. attack_list = []
# loop to make monster fight. while monster1.hp >0 and monster2.hp > 0: # Using round_number to decide which monster will attack
# If rounder number is even monster1 will attack if round_number % 2 == 0: # Monster1 attacks
# finding the index of attack attack_index = m1Index % m1Max # getting attack name from the list. attack = m1Attacks[attack_index] # Decreasing monster2 hp by attack monster2.hp -= monster1.attacks[attack] # Increasing the m1index. m1Index += 1 # If rounder_number is odd monster2 will attack. else: # Saame as above block, just in this monster2 attacks. attack_index = m2Index % m2Max
attack = m2Attacks[attack_index]
monster1.hp -= monster2.attacks[attack]
m2Index += 1
# Adding attack to attack list attack_list.append(attack) # Increasing round number round_number += 1 # Checking which monster loosed if monster1.hp
# calling lose_fight and win_fight on respective monster. monster1.lose_fight() monster2.win_fight()
# returning the data return round_number, monster2, attack_list else: # This means monster2 loosed the fight monster2.lose_fight() monster1.win_fight()
# returning the data return round_number, monster1, attack_list
Step by Step Solution
There are 3 Steps involved in it
Step: 1
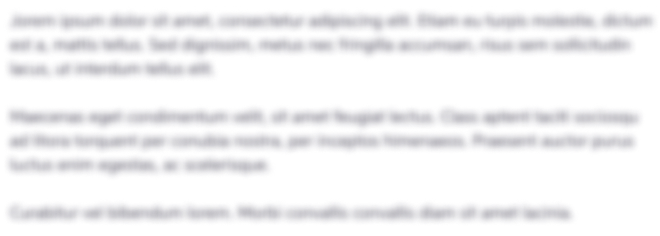
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started