Question
class Node: def __init__(self, data = None, prev = None, next = None): self.data = data self.prev = prev self.next = next class DLL: def
class Node:
def __init__(self, data = None, prev = None, next = None):
self.data = data
self.prev = prev
self.next = next
class DLL:
def __init__(self):
self.header = Node(None, None, None)
self.trailer = Node(None, None, None)
self.header.next = self.trailer
self.trailer.prev = self.header
self.current = self.header
self.header.prev = None
self.trailer.next = None
self.size = 0
def is_empty(self):
return self.size == 0
def insert(self, data):
if self.is_empty():
temp = Node(data, self.trailer, self.header)
self.trailer.prev = temp
self.header.next = temp
self.current = temp
self.size += 1
else:
temp = Node(data, self.current, self.current.next)
if self.current.next is not None:
self.current.next.prev = temp
self.current.next = temp
self.current = temp
self.size += 1
def remove(self):
if self.current is not None and self.current != self.header and self.current != self.trailer:
prev_node = self.current.prev
next_node = self.current.next
prev_node.next = next_node
next_node.prev = prev_node
self.current = next_node
def get_value(self):
if self.current is not None and self.current != self.header and self.current != self.trailer:
return self.current.data
else:
return None
def move_to_next(self):
if self.current is not None and self.current.next is not None:
self.current = self.current.next
def move_to_prev(self):
if self.current is not None and self.current.prev is not None:
self.current = self.current.prev
def move_to_pos(self, pos):
if pos = len(self):
return
node = self.header.next
count = 0
while node and count
node = node.next
count += 1
if node:
self.current = node
def clear(self):
self.header.next = self.trailer
self.trailer.prev = self.header
self.current = self.header
def get_first_node(self):
if self.header.next == self.trailer:
return None
else:
return self.header.next
def get_last_node(self):
if self.trailer.prev == self.header:
return None
else:
return self.trailer.prev
def partition(self, low, high):
if low is None or high is None:
return None
pivot = low
node = low.next
while node != high.next:
if node.data
temp = node.prev
node.prev.next = node.next
node.next.prev = node.prev
node.prev = pivot.prev
node.next = pivot
pivot.prev.next = node
pivot.prev = node
node = temp.next
else:
node = node.next
return pivot
def sort(self):
self.quick_sort(self.get_first_node(), self.get_last_node())
self.current = self.get_first_node()
def quick_sort(self, low, high):
if low is not None and high is not None and low != high and low.prev != high:
pivot = self.partition(low, high)
self.quick_sort(low, pivot.prev)
self.quick_sort(pivot.next, high)
def __len__(self):
return self.size
def __str__(self):
ret_str = ""
node = self.header.next
count = 0
while node and count
ret_str += str(node.data) + " "
node = node.next
count += 1
return ret_str
Expected output should be this but my output is not that.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
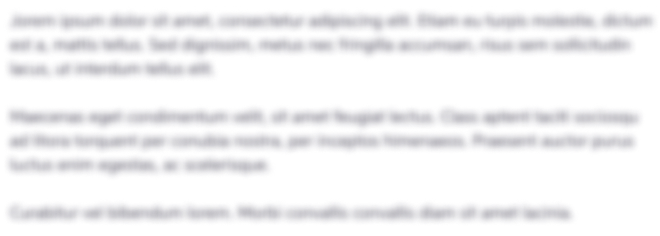
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started