Question
class RoundRobinScheduler: Round Robin Scheduler Simulation. Implement the scheduler Print each time slice or changes Print final reults: table of completion time, turnaround waiting time
class RoundRobinScheduler:
"""Round Robin Scheduler Simulation.
Implement the scheduler
Print each time slice or changes
Print final reults: table of completion time, turnaround waiting time
?Experiment effect of slice time to performane
"""
"""
Nested class for task definition
"""
class _Task:
def __init__(self, id = None):
self._PID = id #the task id, could be string
self._arrivalTime = 0 #the arrival time, in ms
self._taskTime = 0 #the task time required to finish the job, in ms
self._taskTimeLeft = self._taskTime #task time left to be finished. It is initialized using the task time
self._completeTime = 0 #task completed at clock time
def print(self):
print("Task ID: " + self._PID +
"\t Arrival time: " + str(self._arrivalTime) +
"\t Task time: " + str(self._taskTime) +
"\t Complete at: " + str(self._completeTime) +
"\t Completion time: " + str(self._completeTime - self._arrivalTime) +
"\t Waiting time: " + str(self._completeTime - self._arrivalTime - self._taskTime))
def __init__(self):
"""Create an empty queue."""
self._tasksInQueue = ArrayQueue() #This is the Robin Queue
self._clock = 0 #this clock keeps increase by timeslice (quantum time) or task remaining time when it
self._timeSlice = 100 #Here we set the quantum time as 100 ms
self._tasksList = ArrayQueue() #tasks list to be send into the round robin queue
self._setUpTaskList() #method that set up some test data
def _setUpTaskList(self):
"""Initialize the waittime tasks list
"""
newTask = self._Task("P0")
newTask._arrivalTime = 0
newTask._taskTimeLeft = newTask._taskTime = 250
self._tasksList.enqueue(newTask)
newTask = self._Task("P1")
newTask._arrivalTime = 50
newTask._taskTimeLeft = newTask._taskTime = 170
self._tasksList.enqueue(newTask)
newTask = self._Task("P2")
newTask._arrivalTime = 130
newTask._taskTimeLeft = newTask._taskTime = 75
self._tasksList.enqueue(newTask)
def scheduling(self):
"""Scheduling algorithm"""
"""Some suggested notes might help your implementation, BUT YOU CAN ALWAYS DO YOUR OWN WAY!
1 keep running while both tasks list and robin queue is not empty
2 keep enqueue into Robin queue for those arrival time before next time slot
3 process the first task in the Robin Queue
3.1 if the task can be finished with next time slot (_taskTimeLeft
3.2 else clock increases by time slice, _taskTimeLeft decreases by time slice, put back to the queue
"""
"""
YOUR CODE GOES HERE
"""
if __name__ == '__main__':
Q = RoundRobinScheduler()
Q.scheduling()
2. (50 points) Implement the Round Robin Scheduler as described in attached notes "Round Robin Scheduler Example.pdf using queue (you can use either ArrayQueue or LinkedQueue), based on the provided starter Python code robin_scheduler.py". In the starter file, three tasks have already been set in a tasks list according to the example in the attached document. You are only asked to complete the method scheduling. Once you finish your code based on the starter code and run it, the output should be same as bellow. You should test your code using more examples. Task ID: P2 Task ID: P1 Task ID: PO Arrival time: 130 Arrival time: 50 Arrival time: @ Task time: 75 Complete at: 375 Task time: 170 Complete at: 445 Task time: 250 Complete at: 495 Completion time: 245 Completion time: 395 Completion time: 495 Waiting time: 170 Waiting time: 225 Waiting time: 245 Note: Please also read carefully the code and comments in the starter code. class LinkedQueue: RTFIFO queue implementation using a singly linked list for storage." nested Node classe class Mode: **"Lightweight, nonpublic class for storing a singly linked node." _slots__ - '_element', '_next' #streamline nemory usage def __init_(self, element, next): self._clement - element self._next - next queue methods def __init__(self): "*"Create an empty queue. *** self._head None self._tail - None self._size- # number of queue elements def _len_(self): "*"Return the number of elements in the queue." return self._size def is_compty(self): "*"Return True if the queue is empty."** return self._size -- def first (self): **Return (but do not remove) the element at the front of the queue. Raise Empty exception if the queue is empty. if self.is_empty(): print('Queue is empty') return #raise Empty( 'Queue is empty') return self._head. _element # front aligned with head of list def dequeue (self): **Remove and return the first element of the queue (i.c., FIFD). Raise Empty exception if the queue is empty. if self.is_empty(): print('Queue is empty') return #raise Empty( 'Queue is empty') answer = self._head. _element self._head - self._head._next self._size -- 1 if self.is_empty(): self._tail return answer # special case as queue is crpty # removed head had been the tail None def enqueue (self, e): "*"Add an element to the back of queue. *** newest - self._Node(e, None) # node will be now tail node if self.is_empty(): self._head - newest # special case: previously crpty else: self._tail._next - newest self. tail - newest # update reference to tail node self._size = 1 def disp(self): by X3 next - self._head while next 1- None: print(next._clement) next - next._next name --'_main_': lQueue - LinkedQueue() print(Queue. is_empty()) 1Queue.enqueue (1) 1Queue.enqueue (2) 1Queue.enqueue (3) print(Queue. is_empty()) 1Queue.disp() class Arraystack: ***LIFO Stack implementation using a Python list as underlying storage.*** def _init_(self): "Create an empty stack.*** self._data-u1 # nonpublic list instance def _len_(self): "Return the number of elements in the stack."** return len(self. data) def is_empty(self): "Return True if the stack is empty."** return len(self._data) -- def push(self, e): "Add element e to the top of the stack.*** self._data.append(e) #new item stored at end of list def top(self): ***"Return (but do not remove) the clement at the top of the stack. Raise Empty exception if the stack is empty. if self.is empty(): print('stack is empty') return #raise Empty( 'Stack is empty') return self._data1] # the last item in the list def pop(self): "Remove and return the element from the top of the stack (i.c., LIFD). Raise Empty exception if the stack is empty. if self.is_empty(): print('Stack is empty') return #raise Empty( 'Stack is empty') return self._data. #renove last item from list def disp(self): print(self._data) if name _main__': 5 - ArrayStack() # contents: 0 5.push(5) # contents: [5] 5.push(3) # contents: [5, 3] s.disp() print(len(S)) # contents: [5, 3); outputs 2 print(s.pop()) # contents: [5]; outputs 3 print(s.is_empty()) # contents: [5]; outputs False print(s.pop()) # contents: (); outputs 5 print(s.is_empty()) # contents: (); outputs True 5.push(7) # contents: [7] s.push(9) # contents: (7, 9) print(s.top()) # contents: [7, 9); outputs 9 5.push(4) # contents: (7, 9, 4] print(len(s)) # contents: [7, 9, 4); outputs 3 print(s.pop()) # contents: [7, 9); outputs 4 5.push(6) # contents: (7, 9, G] S.push(8) # contents: (7, 9, 6, 8] print(s.pop()) # contents: (7, 9, 6); outputs 8 2. (50 points) Implement the Round Robin Scheduler as described in attached notes "Round Robin Scheduler Example.pdf using queue (you can use either ArrayQueue or LinkedQueue), based on the provided starter Python code robin_scheduler.py". In the starter file, three tasks have already been set in a tasks list according to the example in the attached document. You are only asked to complete the method scheduling. Once you finish your code based on the starter code and run it, the output should be same as bellow. You should test your code using more examples. Task ID: P2 Task ID: P1 Task ID: PO Arrival time: 130 Arrival time: 50 Arrival time: @ Task time: 75 Complete at: 375 Task time: 170 Complete at: 445 Task time: 250 Complete at: 495 Completion time: 245 Completion time: 395 Completion time: 495 Waiting time: 170 Waiting time: 225 Waiting time: 245 Note: Please also read carefully the code and comments in the starter code. class LinkedQueue: RTFIFO queue implementation using a singly linked list for storage." nested Node classe class Mode: **"Lightweight, nonpublic class for storing a singly linked node." _slots__ - '_element', '_next' #streamline nemory usage def __init_(self, element, next): self._clement - element self._next - next queue methods def __init__(self): "*"Create an empty queue. *** self._head None self._tail - None self._size- # number of queue elements def _len_(self): "*"Return the number of elements in the queue." return self._size def is_compty(self): "*"Return True if the queue is empty."** return self._size -- def first (self): **Return (but do not remove) the element at the front of the queue. Raise Empty exception if the queue is empty. if self.is_empty(): print('Queue is empty') return #raise Empty( 'Queue is empty') return self._head. _element # front aligned with head of list def dequeue (self): **Remove and return the first element of the queue (i.c., FIFD). Raise Empty exception if the queue is empty. if self.is_empty(): print('Queue is empty') return #raise Empty( 'Queue is empty') answer = self._head. _element self._head - self._head._next self._size -- 1 if self.is_empty(): self._tail return answer # special case as queue is crpty # removed head had been the tail None def enqueue (self, e): "*"Add an element to the back of queue. *** newest - self._Node(e, None) # node will be now tail node if self.is_empty(): self._head - newest # special case: previously crpty else: self._tail._next - newest self. tail - newest # update reference to tail node self._size = 1 def disp(self): by X3 next - self._head while next 1- None: print(next._clement) next - next._next name --'_main_': lQueue - LinkedQueue() print(Queue. is_empty()) 1Queue.enqueue (1) 1Queue.enqueue (2) 1Queue.enqueue (3) print(Queue. is_empty()) 1Queue.disp() class Arraystack: ***LIFO Stack implementation using a Python list as underlying storage.*** def _init_(self): "Create an empty stack.*** self._data-u1 # nonpublic list instance def _len_(self): "Return the number of elements in the stack."** return len(self. data) def is_empty(self): "Return True if the stack is empty."** return len(self._data) -- def push(self, e): "Add element e to the top of the stack.*** self._data.append(e) #new item stored at end of list def top(self): ***"Return (but do not remove) the clement at the top of the stack. Raise Empty exception if the stack is empty. if self.is empty(): print('stack is empty') return #raise Empty( 'Stack is empty') return self._data1] # the last item in the list def pop(self): "Remove and return the element from the top of the stack (i.c., LIFD). Raise Empty exception if the stack is empty. if self.is_empty(): print('Stack is empty') return #raise Empty( 'Stack is empty') return self._data. #renove last item from list def disp(self): print(self._data) if name _main__': 5 - ArrayStack() # contents: 0 5.push(5) # contents: [5] 5.push(3) # contents: [5, 3] s.disp() print(len(S)) # contents: [5, 3); outputs 2 print(s.pop()) # contents: [5]; outputs 3 print(s.is_empty()) # contents: [5]; outputs False print(s.pop()) # contents: (); outputs 5 print(s.is_empty()) # contents: (); outputs True 5.push(7) # contents: [7] s.push(9) # contents: (7, 9) print(s.top()) # contents: [7, 9); outputs 9 5.push(4) # contents: (7, 9, 4] print(len(s)) # contents: [7, 9, 4); outputs 3 print(s.pop()) # contents: [7, 9); outputs 4 5.push(6) # contents: (7, 9, G] S.push(8) # contents: (7, 9, 6, 8] print(s.pop()) # contents: (7, 9, 6); outputs 8
Step by Step Solution
There are 3 Steps involved in it
Step: 1
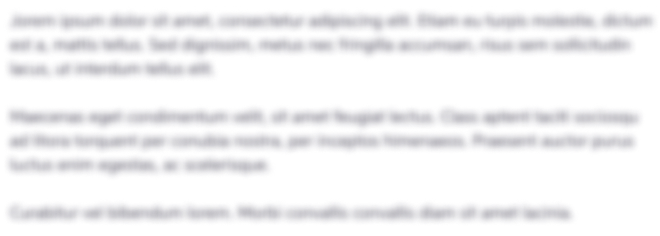
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started