Question
classes, programs using multiple classes, stdDraw (graphics) This program uses a publicly available drawing library created by Robert Sedgewick and Kevin Wayne to simplify the
classes, programs using multiple classes, stdDraw (graphics) This program uses a publicly available drawing library created by Robert Sedgewick and Kevin Wayne to simplify the process of drawing graphic objects to the computer screen. (snowman)
1.Write a class Circle.java similar to store information about a circle that has the attributes: ? two double data members to store the x and y coordinates of the center of the circle ? radius: double variable to store the radius of the circle. ? color - the color of the circle, using one of the predefined constants in the StdDraw package, the data type of this datamember must be Color. The class should have a constructor that takes as parameters the two coordinates ,radius, and color of the circle. public Circle (double inputX, double inputY, double radius, Color color ) The class should also have the following methods: ? public void draw() - for drawing the circle. (Uses the method StdDraw.filledCircle) ? public void setColor(Color newColor) - method that changes the color of the circle. ? public void setRadius( double rad)- method receives a new value for the radius of the circle
2.Write a class Rectangle.java that has attributes: ? x and y - the coordinates of the center of the rectangle, type double ? length: length of 1/2 of the length of the rectangle, type double ? width: - length of 1/2 of the width of the rectangle, type double ? color - the color of the rectangle, using one of the predefined constants in the StdDraw package. The data type of this data member must be Color. The class should have a constructor that takes the two coordinates , length, width and color. public Rectangle (double inputX, double inputY, double len, double wid, Color color) The class should also have the following methods: ? public void draw() - for drawing the rectangle. (Used the method StdDraw.filledRectangle) ? NOTE: To draw a rectangle with StdDraw.filledRectangle you will need to divide the length and width in half. ? public void setColor(Color newColor) - method that changes the color of the rectangle. ? public void setWidth(double newWidth) - changes the width of the rectangle ? public void setLength(double newLength) - changes the length of the rectangle
3.Write a class Snowman.java that will contain the information about the different parts of a snowman. It should contain (at least) the following members: ? bottom: An instance of class Circle ? middle: An instance of class Circle ? head: an instance of class Circle ? hat: An instance of class Rectangle ? leftEye: An instance of class Circle ? rightEye: An instance of class Circle ? mouth: An instance of class Rectangle This class should have 2 constructors: ? Snowman(): A default constructor with no arguments which sets each data member to null; ? Snowman(Circle bottom, Circle middle, Circle head, Rectangle hat, Circle leftEye, Circle rightEye, Rectangle mouth): This constructor receives an instance of either the Circle or rectangle class for each data members. These objects would be created in main and saved into the snowman. The class should also have the methods: ? public void draw(): This calls the appropriate methods from class Circle and Rectangle to draw each part of the snowman. (ie: bottom.draw() ) ? public void setBottom( Circle newBottom) ? public void setMiddle(Circle newMiddle) ? public void setHead (Circle newHead) ? public void setLeftEye(Circle left) ? public void setRightEye(Circle right) ? public void setMouth( Rectangle newMouth) ? public void setHat(Rectangle newHat)
4.Write a tester class BuildSnowman.java with a main method that creates and displays at least one snowman. You can use your creativity to build different parts of the snowman(men) in different colors, sizes and shapes. The main method must set up the drawing canvas for StdDraw by calling : StdDraw.setXscale(0.0, 300.0); StdDraw.setYscale(0.0, 300.0); Remember the BOTTOM left corner is x=0, y=0. The main method will create instances of Rectangles and Circles to represent the different parts of a snowmans body, and then call the constructor for class Snowman to create a snowman object and then finally draw it.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
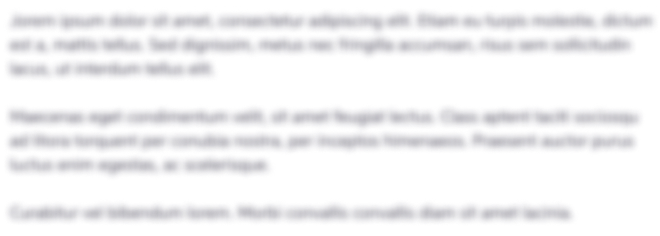
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started