Question
ClockTimeTest.java // DO NOT MODIFY THIS FILE. public class ClockTimeTest { public static void main(String[] args) { testConstructor(); testGetters(); testToString(); testEquals(); testCompareTo(); } private static
ClockTimeTest.java
// DO NOT MODIFY THIS FILE.
public class ClockTimeTest { public static void main(String[] args) { testConstructor(); testGetters(); testToString(); testEquals(); testCompareTo(); }
private static void testConstructor() { System.out.println("Testing constructor");
try { new ClockTime(12, 0, "am"); new ClockTime(11, 59, "am"); new ClockTime(12, 0, "pm"); new ClockTime(11, 59, "pm"); new ClockTime(1, 0, "am"); } catch (Exception e) { System.out.println("An exception is not supposed to occur here, but it did"); }
try { new ClockTime(0, 10, "am"); System.out.println("An exception was supposed to occur here, but didn't"); } catch (Exception ignored) { // exception should be caught here }
try { new ClockTime(10, -1, "am"); System.out.println("An exception was supposed to occur here, but didn't"); } catch (Exception ignored) { // exception should be caught here }
try { new ClockTime(13, 10, "am"); System.out.println("An exception was supposed to occur here, but didn't"); } catch (Exception ignored) { // exception should be caught here }
try { new ClockTime(10, 60, "am"); System.out.println("An exception was supposed to occur here, but didn't"); } catch (Exception ignored) { // exception should be caught here }
try { new ClockTime(10, 33, "a String"); System.out.println("An exception was supposed to occur here, but didn't"); } catch (Exception ignored) { // exception should be caught here }
System.out.println(); }
private static void testGetters() { System.out.println("Testing getters"); ClockTime time = new ClockTime(10, 33, "pm"); System.out.println(time.getHour()); // expected: 10 System.out.println(time.getMinute()); // expected: 33 System.out.println(time.getAmOrPm()); // expected: pm System.out.println(); }
private static void testToString() { System.out.println("Testing toString"); System.out.println(new ClockTime(10, 33, "pm")); // expected: 10:33pm System.out.println(new ClockTime(5, 7, "am")); //expected: 5:07am System.out.println(); }
private static void testEquals() { System.out.println("Testing equals"); System.out.println(new ClockTime(10, 33, "pm").equals(new ClockTime(10, 33, "pm"))); // expected: true System.out.println(new ClockTime(10, 33, "pm").equals(new ClockTime(10, 33, new String("pm")))); // expected: true System.out.println(new ClockTime(10, 33, "pm").equals(new ClockTime(10, 33, "am"))); // expected: false System.out.println(new ClockTime(10, 33, "pm").equals(new ClockTime(10, 45, "pm"))); // expected: false System.out.println(new ClockTime(10, 33, "pm").equals(new ClockTime(11, 33, "pm"))); // expected: false System.out.println(new ClockTime(10, 33, "pm").equals("a String")); // expected: false System.out.println(new ClockTime(10, 33, "pm").equals(null)); // expected: false System.out.println(); }
private static void testCompareTo() { System.out.println("Testing compareTo");
// test cases are from: https://practiceit.cs.washington.edu/problem/view/cs2/sections/comparable/ClockTime System.out.println(new ClockTime(3, 15, "pm").compareTo(new ClockTime(5, 45, "pm")) 0); // expected: true System.out.println(new ClockTime(11, 59, "pm").compareTo(new ClockTime(12, 0, "am")) > 0); // expected: true System.out.println(new ClockTime(11, 59, "am").compareTo(new ClockTime(12, 0, "pm")) 0); // expected: true System.out.println(new ClockTime(12, 59, "am").compareTo(new ClockTime(1, 0, "am")) 0); // expected: true System.out.println(new ClockTime(12, 59, "pm").compareTo(new ClockTime(1, 0, "pm")) 0); // expected: true System.out.println(new ClockTime(5, 55, "am").compareTo(new ClockTime(5, 55, "pm")) Write a class named ClockTime. Each object of this class should represent a time on a standard 12-hour clock, storing the hour, min ute, and either "am" or "pm". The earliest possible time is 12:00am, and the latest possible time is 11:59pm. The constructor should have three parameters: an int for the hour, an int for the minute, and a String that is either "am" or "pm". If an of the parameters is not valid, the constructor should throw an IllegalArgumentException. The valid hours are 1-12, the valid minutes are 059, and thestring must either be "am" or "pm" to be valid. There should be standard getter methods: getHour, getMinute, getAmorPm. ClockTime should override the toString method (of the Object class) so that it returns a String consisting of the following: the hour ( one or two digits), then a colon (:), then the minute (always two digits), and then either "am" or "pm". Examples: "10:33am", "9:45pm" "12:07pm". ClockTime should also override the equals method of the Object class. Equality should be determined in the obvious way: two Cloc kTimes should be considered equal if and only if they have the same hour, have the same minute, and are either both "am" or both "pm". ClockTime should implement the Comparable interface. ClockTimes should be ordered chronologically. Examples: - 2:30am should be considered less than 1:30pm - 12:30am should be considered less than 1:30am Paste your solution in ClockTime.java, below. The other file, ClockTimeTest.java, contains code to test your solution. You may copy the testing code, but do not modify it. (This exercise is mostly taken from a Practice-It exercise.) Additional Notes: Regarding your code's standard output, CodeLab will check for case errors but will ignore whitespace (tabs, spaces, newlines) altogether
Step by Step Solution
There are 3 Steps involved in it
Step: 1
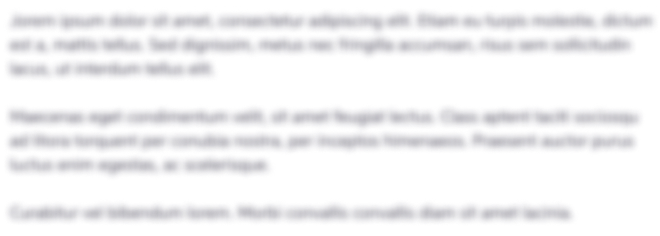
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started