Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Code 1 : import numpy as np import pandas as pd from PIL import Image from sklearn.preprocessing import StandardScaler # Initialize dataframe df = pd
Code:
import numpy as np
import pandas as pd
from PIL import Image
from sklearn.preprocessing import StandardScaler
# Initialize dataframe
df pdDataFramenpzeros
count
for i in range:
for j in range:
image Image.openUsersORLFaces stri strjpng
imagearray nparrayimage
imagearray imagearray.reshape
dfiloccount imagearray
count
# Add gender labels to the dataframe
dfGender npones
# Modify certain entries to represent actual gender labels
dfiloc:
dfiloc:
dfiloc:
dfiloc:
from sklearn.cluster import KMeans
# Standardize image data
X dfiloc::
scaler StandardScaler
X scaler.fittransformX
# Apply KMeans clustering
kmeansmodel KMeansnclusters
kmeansmodel.fitX
# Add cluster labels to the dataframe
dfKMeansClusters" kmeansmodel.labels
from sklearn.cluster import AgglomerativeClustering
from sklearn.preprocessing import StandardScaler
import pandas as pd
# Assuming df is your dataframe and it has been loaded correctly
X dfiloc:: # Adjust the slice as necessary to include the right columns
# Standardize image data for hierarchical clustering
scaler StandardScaler
Xscaled scaler.fittransformX
# Apply Hierarchical clustering using Ward's method
hierarchicalcluster AgglomerativeClusteringnclusters linkage'ward'
hierarchicalcluster.fitXscaled
# Add cluster labels to the dataframe
dfHierarchicalClusters" hierarchicalcluster.labels
# Calculate true and false labelings for KMeans clustering
truelabelkmeans sumdfKMeansClusters" dfGender
falselabelkmeans sumdfKMeansClusters" dfGender
# Calculate true and false labelings for Hierarchical clustering
truelabelhierarchical sumdfHierarchicalClusters" dfGender
falselabelhierarchical sumdfHierarchicalClusters" dfGender
import matplotlib.pyplot as plt
pltfigurefigsize
pltsubplot
pltscattertruelabelkmeans, falselabelkmeans, color'blue'
plttitleKMeans Clustering'
pltxlabelTrue Labels'
pltylabelFalse Labels'
pltsubplot
pltscattertruelabelhierarchical, falselabelhierarchical, color'red'
plttitleHierarchical Clustering'
pltxlabelTrue Labels'
pltylabelFalse Labels'
plttightlayout
pltshow
printKMeans Clustering Results:"
print# of True Labeling:", truelabelkmeans
print# of False Labeling:", falselabelkmeans
print
Hierarchical Clustering Results:"
print# of True Labeling:", truelabelhierarchical
print# of False Labeling:", falselabelhierarchical
Code:
import numpy as np
import matplotlib.pyplot as plt
from sklearn.datasets import fetcholivettifaces, loaddigits
from sklearn.cluster import KMeans, AgglomerativeClustering
from sklearn.metrics import silhouettescore
try:
# Try to fetch the Olivetti faces dataset
data fetcholivettifaces
X data.data
y data.target
datasetname "Olivetti Faces"
except Exception as e:
printFailed to fetch Olivetti Faces dataset:", e
# Fallback to a different dataset, eg loaddigits
data loaddigits
X data.data
y data.target
datasetname "Digits"
# Apply kmeans clustering
kmeans KMeansnclusters randomstate
kmeanslabels kmeans.fitpredictX
# Apply hierarchical clustering
hierarchical AgglomerativeClusteringnclusters
hierarchicallabels hierarchical.fitpredictX
# Evaluate clustering performance using silhouette score
kmeansscore silhouettescoreX kmeanslabels
hierarchicalscore silhouettescoreX hierarchicallabels
# Print the silhouette scores
printfSilhouette Score for KMeans on datasetname: kmeansscore
printfSilhouette Score for Hierarchical Clustering on datasetname: hierarchicalscore
# Visualize the clustering results
pltfigurefigsize
pltsubplot
pltscatterX: X: ckmeanslabels, cmap'viridis', s
plttitlefKMeans Clustering on datasetname
pltxlabelFeature
pltylabelFeature
pltsubplot
pltscatterX: X: chierarchicallabels, cmap'viridis', s
plttitlefHierarchical Clustering on datasetname
pltxlabelFeature
pltylabelFeature
plttightlayout
pltshow
Please use the dataset of Codethe link for Code combine the codes together, and give me the complete code. Thank you!:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
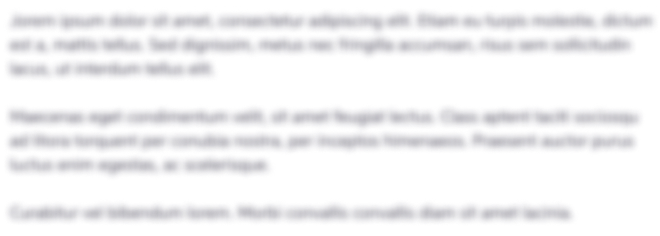
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started