Question
(code) # 14.1 Create, then run, a program, passing it command-line arguments and recovering its output # ## import directives ## # subprocess.run - executes
(code)
# 14.1 Create, then run, a program, passing it command-line arguments and recovering its output
# ## import directives ## # subprocess.run - executes a program in a subshell # os.path.exists - check if a path expression denotes an object of some sort # os.remove - deletes specified file # import subprocess, os
# ## program constants ## CREATE_NEW_FILE = 'x' # python open mode FAILURE_EXIT = 1 # POSIX error code for program failure
# ## supporting functions ## byte_seq_to_string = lambda byteseq: ''.join( chr(byte) for byte in byteseq )
# - program to create # program_file_name = 'test.py' program_content = [ '# import directives ', '# - sys.argv - list of command line arguments ', '# - sys.stderr - error output ', '# ', 'import sys ', ' ', '# program constants ', 'FAILURE_EXIT = 1 # POSIX error code for program failure ', ' ', '# supporting functions ', '# ', 'def make_numeric(string): ' ' try: ', ' return int(string) ', ' except ValueError: ', ' return float(string) ', ' ', 'def plus(x, y): return x + y ', ' ', 'if __name__ == "__main__": ', ' try: ', ' assert len(sys.argv) >= 3, f"?? {sys.argv[0]}: insufficient arguments ({len(sys.argv)-1}); 2 required" ', ' a, b = make_numeric(sys.argv[1]), make_numeric(sys.argv[2]) ', ' print( a, "+", b, "is", plus(a, b)) ', ' except Exception as exception: ', ' exception_message = "" if str(exception) is None else str(exception) ', ' print( f"?? {sys.argv[0]}: exiting", exception_message, file=sys.stderr ) ' ' exit( FAILURE_EXIT ) ' ] trial_1 = [ '3', '4.2' ] trial_2 = [ '3', 'four point two'] trial_3 = [ '3' ] list_of_trials = [ trial_1, trial_2, trial_3 ]
# main proper # if os.path.exists( program_file_name ): print( f'{program_file_name} already exists; please remove or rename it and rerun the example' ) else: with open( program_file_name, CREATE_NEW_FILE ) as program_fd: for line in program_content: program_fd.write( line ) for (trial_number, this_trial) in enumerate(list_of_trials): print( 'executing sample program with argument list of ', this_trial ) program_status = subprocess.run( [ 'python', program_file_name ] + this_trial, capture_output=True ) print( 'return code is', program_status.returncode ) standard_output = byte_seq_to_string( program_status.stdout ) print( '> no standard output returned no error output returned
(output)
executing sample program with argument list of ['3', '4.2'] return code is 0 standard output: 3 + 4.2 is 7.2 > no error output returned no standard output returned no standard output returned(language python)
# 14.1 Create, then run, a program, passing it command-Line arguments and recovering its output # ## import directives ## # subprocess.run - executes a program in a subshell # os.path.exists - check if a path expression denotes an object of some sort # os.remove - deletes specified file '# ', import subprocess, os # ## program constants ## CREATE_NEW_FILE = 'X' # python open mode FAILURE_EXIT = 1 # POSIX error code for program failure # ## supporting functions ## byte_seq_to_string - lambda byteseg: ".join( chr(byte) for byte in byteseg) # - program to create program_file_name = 'test.py" program_content = [ '# import directives ', '# - sys.argv - list of command line arguments ', '# - sys.stderr - error output ', 'import sys ', '# program constants ', 'FAILURE_EXIT = 1 # POSIX error code for program failure ', ' ', # supporting functions ', '# ', 'def make_numeric(string): ' try: ", return int (string) ', except valueError: ', return float(string) ', 'def plus(x, y): return x + y ', 'if name "_main_": ', try: ', assert len(sys.argv) >= 3, f"?? (sys.argv[@]}: insufficient arguments ({len(sys.argv)-1}); 2 required" ', a, b = make_numeric(sys.argv[1]), make_numeric(sys.argv[2]) ', print(a, +", b, "is", plus(a, b) ', " ' ' ', ' ', 1 1 ' ', ' ', == . '# supporting functions ', # ', def make_numeric(string): ' try: ", return int(string) ', except valueError: ', return float(string) ', 'def plus(x, y): return x + y ', 'if _name_ "_main_": ', try: ', assert len(sys.argv) >= 3, f"?? {sys.argv[@]}: insufficient arguments ({len(sys.argv)-1}); 2 required" ', a, b = make_numeric(sys.argv[1]), make_numeric(sys.argv[2]) ', print( a, "+", b, "is", plus (a, b)) ', except Exception as exception: ', exception_message = "" if str(exception) is None else str(exception) ', print( f"?? {sys.argv[@]}: exiting", exception_message, file-sys.stderr) ' exit( FAILURE_EXIT ) ' trial_1 = [ '3', '4.2' ] trial_2 = [ '3', 'four point two'] trial 3 = [ '3''] list_of_trials = [ trial_1, trial_2, trial_3 ] # main proper if os.path.exists( program_file_name): print( f'{program_file_name} already exists; please remove or rename it and rerun the example' ) else: with open( program_file_name, CREATE_NEW_FILE ) as program_fd: for line in program_content: program_fd.write( line) for (trial_number, this_trial) in enumerate(list_of_trials): print('executing sample program with argument list of, this_trial ) program_status = subprocess.run( ['python', program_file_name] + this_trial, capture_output=True) print( return code is', program_status.returncode ) standard_output = byte_seq_to_string( program_status.stdout) print( no standard Output returned ' if standard_output == "else 'standard output: ' + standard_output) error_output = byte_seq_to_string( program_status.stderr ). print > no error output returned
= 3, f"?? (sys.argv[@]}: insufficient arguments ({len(sys.argv)-1}); 2 required" ', a, b = make_numeric(sys.argv[1]), make_numeric(sys.argv[2]) ', print(a, +", b, "is", plus(a, b) ', " ' ' ', ' ', 1 1 ' ', ' ', == . '# supporting functions ', # ', def make_numeric(string): ' try: ", return int(string) ', except valueError: ', return float(string) ', 'def plus(x, y): return x + y ', 'if _name_ "_main_": ', try: ', assert len(sys.argv) >= 3, f"?? {sys.argv[@]}: insufficient arguments ({len(sys.argv)-1}); 2 required" ', a, b = make_numeric(sys.argv[1]), make_numeric(sys.argv[2]) ', print( a, "+", b, "is", plus (a, b)) ', except Exception as exception: ', exception_message = "" if str(exception) is None else str(exception) ', print( f"?? {sys.argv[@]}: exiting", exception_message, file-sys.stderr) ' exit( FAILURE_EXIT ) ' trial_1 = [ '3', '4.2' ] trial_2 = [ '3', 'four point two'] trial 3 = [ '3''] list_of_trials = [ trial_1, trial_2, trial_3 ] # main proper if os.path.exists( program_file_name): print( f'{program_file_name} already exists; please remove or rename it and rerun the example' ) else: with open( program_file_name, CREATE_NEW_FILE ) as program_fd: for line in program_content: program_fd.write( line) for (trial_number, this_trial) in enumerate(list_of_trials): print('executing sample program with argument list of, this_trial ) program_status = subprocess.run( ['python', program_file_name] + this_trial, capture_output=True) print( return code is', program_status.returncode ) standard_output = byte_seq_to_string( program_status.stdout) print( no standard Output returned ' if standard_output == "else 'standard output: ' + standard_output) error_output = byte_seq_to_string( program_status.stderr ). print > no error output returned
Step by Step Solution
There are 3 Steps involved in it
Step: 1
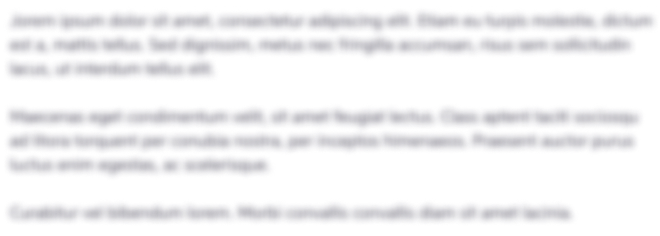
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started