Answered step by step
Verified Expert Solution
Question
1 Approved Answer
code for ArrayStack.java: public class ArrayStack implements Stack { public static final int INITIAL_CAPACITY = 10; private E[] elems; private int top; @SuppressWarnings(unchecked) public ArrayStack()
code for ArrayStack.java:
public class ArrayStackimplements Stack { public static final int INITIAL_CAPACITY = 10; private E[] elems; private int top; @SuppressWarnings("unchecked") public ArrayStack() { elems = (E[]) new Object[INITIAL_CAPACITY]; top = 0; } public boolean isEmpty() { return top == 0; } @SuppressWarnings("unchecked") public E push(E elem) { if (top == elems.length) { Object[] tmp = new Object[2 * elems.length]; for (int i = 0; i ["); for (int i = 0; i
Stack.java:
public interface Stack{ boolean isEmpty(); E push(E elem); E pop(); E peek(); } Q2Test.java:
public class Q2Test { public static void main(String[] args) { UniquifiableArrayStackintegerStack = new UniquifiableArrayStack (); integerStack.push(0); integerStack.push(1); integerStack.push(1); integerStack.push(1); integerStack.push(2); integerStack.push(2); integerStack.push(3); integerStack.push(3); integerStack.push(3); integerStack.push(4); System.out.println("Original Integer stack: " + integerStack); System.out.println("Integer stack without immediately consecutive duplicates: " + integerStack.uniquify()); System.out.println("Original Integer stack (after uniquify): " + integerStack); System.out.println(); UniquifiableArrayStack stringStack = new UniquifiableArrayStack (); stringStack.push("a"); stringStack.push("a"); stringStack.push("b"); stringStack.push("b"); stringStack.push("b"); stringStack.push("c"); stringStack.push("d"); stringStack.push("d"); stringStack.push("d"); stringStack.push("e"); stringStack.push("e"); System.out.println("Original String stack: " + stringStack); System.out.println("String stack without immediately consecutive duplicates: " + stringStack.uniquify()); System.out.println("Original String stack (after uniquify): " + stringStack); System.out.println(); System.out.println("---- Now, testing with some non-consecutive duplicates ----- "); System.out.println(); UniquifiableArrayStack stringStackWithNonConsecutiveDuplicates = new UniquifiableArrayStack (); stringStackWithNonConsecutiveDuplicates.push("a"); stringStackWithNonConsecutiveDuplicates.push("b"); stringStackWithNonConsecutiveDuplicates.push("b"); stringStackWithNonConsecutiveDuplicates.push("c"); stringStackWithNonConsecutiveDuplicates.push("a"); stringStackWithNonConsecutiveDuplicates.push("d"); stringStackWithNonConsecutiveDuplicates.push("d"); stringStackWithNonConsecutiveDuplicates.push("e"); stringStackWithNonConsecutiveDuplicates.push("e"); stringStackWithNonConsecutiveDuplicates.push("d"); stringStackWithNonConsecutiveDuplicates.push("d"); stringStackWithNonConsecutiveDuplicates.push("d"); stringStackWithNonConsecutiveDuplicates.push("d"); stringStackWithNonConsecutiveDuplicates.push("b"); System.out.println("Original String stack: " + stringStackWithNonConsecutiveDuplicates); System.out.println("String stack without immediately consecutive duplicates: " + stringStackWithNonConsecutiveDuplicates.uniquify()); System.out.println("Original String stack (after uniquify): " + stringStackWithNonConsecutiveDuplicates); } }
UniquifiableArrayStack.java: (the file to edit)
public class UniquifiableArrayStackQuestion 2 (10 marks) For this question, you are going to develop a specialization (subclass) of ArrayStack. ArrayStack has been already covered in the course lectures. The subclass, named UniquifiableArrayStack, provides a single additional method, named uniquify(). public class UniquifiableArrayStackextends ArrayStack { public Stack uniquify() { // ADD YOUR CODE HERE //Remove the following line when this method has been implemented return null; } } extends ArrayStack { public Stack uniquify () { // All the code that you write for Question 2 goes here! } The method uniquify) does the following: It returns a stack which is the same as the UniquifiableArrayStack instance that the method is called on, except that the returned stack has no immediately consecutive duplicate elements. To illustrate what "immediately consecutive duplicate elements" are, consider the example stack below: immediately consecutive duplicate elements "e" "e" "d" "d" "d" "C" "b" immediately consecutive duplicate elements immediately consecutive duplicate elements "b" "b" "a" immediately consecutive duplicate elements "a" More precisely, two stack elements are immediately consecutive duplicate elements, when they are adjacent and have identical content. Having identical content for (non-null) stack elements eleml and elem2 means that eleml.equals(elem2) is true. You can assume that all stack elements are non-null. In Question 2, you complete the method uniquify() in the UniquifiableArrayStack class, so that only one instance of immediately consecutive duplicate elements would be retained. For example, if uniquify() is called over the example stack above (an instance of UniquifiableAr- rayStack ), the result should be as shown in the figure on the top of the next page. "e" "d" "C" "b" "a" Note that uniquify() should not have any side effect on the UniquifiableArrayStack instance that the method is called on. Furthermore, uniquify() is not meant to deal with non-consecutive duplicate elements. In other words, the stack returned by uniquify() can have non-consecutive duplicate elements. The absence of side effects on the original stack as well as the carry-over of non-consecutive duplicate elements to the result of uniquify() are illustrated in the example output by Q2Test, presented next. Example Output To test your implementation of uniquify0), you can use the Q2Test.java in the template code provided to you. The output from running Q2Test should be as follows: Original Integer stack: bottom->[0, 1, 1, 1, 2, 2, 3, 3, 3, 4] (0, 1, 2, 3, 4][0, 1, 1, 1, 2, 2, 3, 3, 3, 4][a, a, b, b, b, c, d, d, d, e, e][a, b, c, d, e][a, a, b, b, b, c, d, d, d, e, e][a, b, b, c, a, d, d, e, e, d, d, d, d, b] [a, b, c, a, d, e, d, b][a, b, b, c, a, d, d, e, e, d, d, d, d, b]. Stated otherwise, you cannot declare any reference variable that has a different type than either E or Stack . You are allowed to use local primitive variables if you find them necessary, but please note that it is feasible to implement uniquify() without using any local primitive variables
Step by Step Solution
There are 3 Steps involved in it
Step: 1
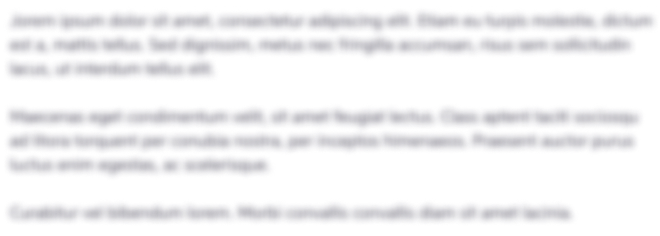
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started