Question
Code for c++. Specify, design, and implement a classes called Statistician . After a statistician is initizlized, it can given a sequence of double numbers.
Code for c++.
Specify, design, and implement a classes called Statistician. After a statistician is initizlized, it can given a sequence of double numbers. Each number in the sequence is given to the statistician by activating a method called nextNumber. For example, we can declare a statistician called s and then give it the sequence of numbers 1.1, -2.4, 0.8 as shown here:
Statistician s = new Statistician();
s.nextNumber(1.1);
s.nextNumber(-2.4);
s.nextNumber(0.8);
After a sequence has been given to a statistician, there are various methods to obtain information about the sequence. Include methods that will provide the length of the sequence, the last number of the sequence, the sum of all the numbers in the sequence, the arithmetic mean of the numbers (i.e., the sum of the numbers divided by the length of the sequence), the smallest number in the sequence, and the largest number in the sequence.
Notice that the length and sum methods can be called at any time, even if there are no numbers in the sequence. In this case of an "empty" sequence, both length and sum will be zero. The other methods should return Double.NaN if they are called for an empty sequence:
Notes: Do not try to store the entire sequence. Instead, just store the necessary information about the sequence: What is the sequence length, what is the sum of the numbers in the sequence, and what are the last, smallest and largest numbers? Each of these pieces of information can be stored in a private instance variable that is updated whenever nextNumber is activated.
Overload the + operator to allow you to add two statisticians from the project. If s1 and s2 are two statisticians, then the result of s1+s2 should be a new statistician that behaves as if it had all of the numbers of s1 followed by all of the numbers of s2.
I have this code so far:
statistician.cpp.
#include "Statistician.h"
Statistician::Statistician()
{
double sLength=0;
double sumNumb=0;
double smallest=9999;
double largest=-0.999;
}
void Statistician::nextNumber(double numb)
{
sLength++;
sumNumb+=numb;
lastNumb=numb;
if (smallest > numb)
smallest = numb;
if (largest < numb)
largest = numb;
}
double Statistician::sequenceLength()
{
return sLength;
}
double Statistician::sumNumbers()
{
return sumNumb;
}
double Statistician::smallestNumber()
{
if (sLength !=0 )
return smallest;
std::cout << "Error Sequence Is Empty";
}
double Statistician::largestNumber()
{
if (sLength !=0)
return largest;
std::cout << "Error Sequence Is Empty";
}
double Statistician::lastNumber()
{
return lastNumb;
}
void Statistician::getMean()
{
if (sLength==0)
std::cout << "Error Sequence Is Empty";
else
std::cout << "Arithmetic Mean Is: " <<(sumNumb/sLength);
}
void Statistician::deleteNumbers()
{
sLength=0;
sumNumb=0;
smallest=9999;
largest=-0.999;
}
Statistican.h
#ifndef STATISTICIAN_H
#define STATISTICIAN_H
#include
#include
#pragma once
class Statistician
{
private: //Data Members
double sLength;
double sumNumb;
double smallest;
double largest;
double lastNumb;
public:
Statistician(); // Constructor
double sequenceLength(); //Member Functions
double sumNumbers();
double smallestNumber();
double largestNumber();
double lastNumber();
void getMean();
void nextNumber(double);
void deleteNumbers();
};
#endif // STATISTICIAN_H
main.cpp
#include
#include "Statistician.h"
using namespace std;
int main()
{
Statistician s1;
s1.nextNumber(1.1);
s1.nextNumber(-2.4);
s1.nextNumber(0.8);
cout << "Largest Number: " << s1.largestNumber() << endl;
cout << "Smallest Number: " << s1.smallestNumber() << endl;
cout << "Sequence Length: " << s1.sequenceLength() << endl;
cout << "Last Number: " << s1.lastNumber() << endl;
cout << "Sum Of Sequence Numbers: " << s1.sumNumbers() << endl;
s1.getMean();
s1.deleteNumbers();
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
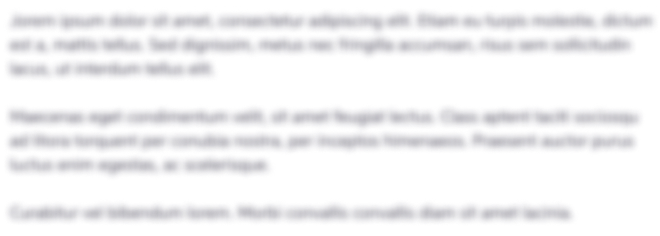
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started