Question
CODE IN C# (C SHARP) [2] Create a console application called CartDemo and add two classes in it: Product and ShoppingCart. You are provided with
CODE IN C# (C SHARP)
[2] Create a console application called CartDemo and add two classes in it: Product and ShoppingCart. You are provided with the content of the Program.cs file in the console application. Just click this link to download it. You must use it exactly as is:
using System; namespace CartDemo { class Program { static void Main(string[] args) { Product ball = new Product(1, 1000, 10) { Name = "Ball" }; Product tv = new Product(2, 1000, 1000) { Name = "TV" }; Product picture = new Product(3, 50, 110) { Name = "Picture" }; ShoppingCart cart1 = new ShoppingCart(1); ShoppingCart cart2 = new ShoppingCart(2); cart1.AddProduct(ball); cart1.AddProduct(tv); cart1.AddProduct(picture); Console.WriteLine(cart1); Console.WriteLine(cart2); tv.StockQuantity += 10; cart2.AddProduct(ball); cart2.AddProduct(tv); cart2.AddProduct(picture); Console.WriteLine(cart1); Console.WriteLine(cart2); } } }
The class diagrams are shown below. Make sure that your classes conform to them exactly.
[2] Create Product class.
Create the following class members:
[1] basePrice is a private read only field representing the base price of the product
[1] price is the private field representing current product. It is the dependent on the stock quantity of the product. If the quantity is less then 1000 the current price is 110% of base price, otherwise it is equal to base price.
[1] stockQuantity is private field representing the quantity of the product in stock
[3] StockQuantity is property that is accessor to stockQuantity field.
[1] Name is property representing the name of the product.
[1] SKU is read only property representing the stock keep number of the product.
[1] Price is read only property that is accessor for price field.
[2] A constructor with three parameters: SKU number, number of products in stock, and base price. It assigns them to appropriate members.
[1] Create ShoppingCart class
Create the following class members:
[1] cartValue is a private field representing the total price of all products in the cart and it is based on the current price of the product at the time when it was added to the shopping cart.
[1] CartValue is read only property providing the access to the cartValue field.
[1] Id is a read only property representing cart id.
[1] Products is read only property representing the list of products in the cart. You can create the list as you declare it or in the constructor.
[1] A constructor that takes 1 parameter: cart id and assigns the values to appropriates property.
[2] AddProduct method has one parameter: product object. The method will add the product to the list of products in the cart, and update the cart value. It also reduces added product stock quantity by 1.
[2] ToString() method that generates the string with information about the cart. Make sure that your output matches the one that is provided in the output image of the console result down below.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
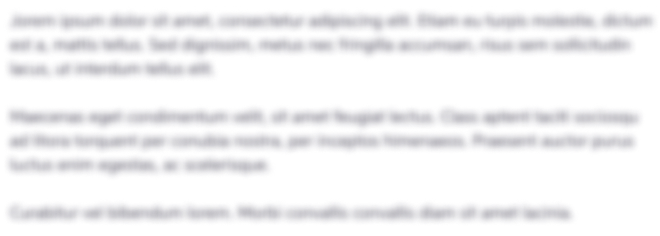
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started