Question
Code in c. Need the same result as defined output appearance like the one below. lab5.c #include lab5.h int main(void) { double l, w, h;/*
Code in c. Need the same result as defined output appearance like the one below.
lab5.c
#include "lab5.h"
int main(void)
{
double l, w, h;/* measures of the box */
double vol; /* volume of the box */
double s_area;/* surface area of the box */
FILE * data_in; /* input file pointer */
FILE * data_out; /* output file pointer */
data_out = open_out_file();
data_in = open_in_file();
print_headers(data_out);
find_box_values(data_out, data_in, l, h, w, &vol, &s_area);
fclose(data_in);
fclose(data_out);
return EXIT_SUCCESS;
}
/*-----------------------------------------------------------*/
lab5.h
// Lab 5 header file
// This file should not have any code added to it.
// This file must be included in your function find_box_values.c
#include
#include
#define IN_FILENAME "lab5.dat"
#define OUT_FILENAME "lab5.txt
"/* File Pointers */FILE *
open_out_file(void);
FILE * open_in_file(void);
/* Function Prototypes */
void print_headers(FILE *data_out);
void find_box_values(FILE *data_in, FILE *data_out, double l, double h, double w, double*vol, double*s_area);
open_out_file.c
#include "lab5.h"
FILE * open_out_file (void)
{
FILE * data_out; data_out = fopen(OUT_FILENAME, "w");
if (data_out == NULL)
{
printf("Error on fopen of %s ", OUT_FILENAME);
exit(EXIT_FAILURE);
}
return data_out;
}
open_in_file.c
#include "lab5.h"
FILE * open_in_file (void)
{
FILE * data_in; data_in = fopen(IN_FILENAME, "r");
if (data_in == NULL)
{
printf("Error on fopen of %s ", IN_FILENAME);
exit(EXIT_FAILURE);
}
return data_in;
}
print_headers.c
#include "lab5.h"
void print_headers(FILE *data_out)
{
fprintf(data_out, " Your Name, Lab5. ");
fprintf(data_out, " Length Width Height Volume SurfaceArea ");
fprintf(data_out, " ------ ----- ------ ------ ----------- ");
return;
}
lab5.dat
3.7 5.0 4.2
6.8 3.0 5.9
4.0 3.3 6.1
5.6 7.3 5.6
PROBLEM: 1. Write a function that finds both volume and surface area of a rectangular box. 2. Write a makefile to pull all these files together. Your function must use pointer notation (the address operator "&" and the indirection operator "*"). This function will be in a file separate and on its own. The function prototype is: /* Function to compute the volume and the surface area of a rectangular box */ void find_box_values (FILE * data_out, FILE *data_in, double I, double h, double w, double *vol, double *s_area); You will need the file lab5.c as your main/driver program for the function. This main program will call all the subfunctions: open_out_file.c open_in_file.c print_headers.c find_box_values.c THE FORMULAS (in algebraic notation)(must be translated to Cnotation): (1) the volume of a rectangular box. v=1*h*w (2) the surface area of a rectangular box. s_area = 2(1*w +h*w +h*1) INPUT/OUTPUT DESCRIPTION: The input is a list of varying length of type double values in the file lab5.dat. Each record (or line) will have three values for the three measures of the box. The data file will have four sets of values. The output is a chart showing the three measures of the box, the volume, and surface area. DEFINED OUTPUT APPEARANCE: Print statements included in the code, and require no changes, except for your name. Your Name, Lab5. Length Width Height Volume SurfaceArea ----- ------ --- 3.70 6.80 5.00 3.00 4.20 5.90 77.70 120.36 110.08 156.44 ONLY the two lines are shown here. You should validate the correctness of the other lines. ALGORITHM DEVELOPMENT - Pseudo code: /* ------ */ main /* main is given to you as lab5.c */ Call function open_out_file. Will open lab5.txt, mentioned in lab5.h. Call function open_in_file Will open lab5.dat, mentioned in lab5.h Call function print_headers Will print the column headers Call function find_box_values You write this one. Close the files. /* This code will reside in a file find_box_values.c /* In a separate file from the other functions */ // You need to do a #include of lab5.h void find_box_values(FILE *data_out, FILE *data_in, //fixed double I, double h, double w, double* vol, double*s_area) while( do an fscanf to read the I, w, h) ==3) 1 Calculate the *vol 1 Calculate the *s_area | Print the length, height, th, volume, surfaceArea print one more empty line. return /* -*/ REMINDERS: You should look at all the provided files and get an idea of what code each file contains. Remember to put your name and Lab 5 in the comment header of your function, in your makefile, and in the output. Remember the only operator for multiplication is the asterisk (*). You should examine the data file and confirm the correctness of the answer produced by your program. PROBLEM: 1. Write a function that finds both volume and surface area of a rectangular box. 2. Write a makefile to pull all these files together. Your function must use pointer notation (the address operator "&" and the indirection operator "*"). This function will be in a file separate and on its own. The function prototype is: /* Function to compute the volume and the surface area of a rectangular box */ void find_box_values (FILE * data_out, FILE *data_in, double I, double h, double w, double *vol, double *s_area); You will need the file lab5.c as your main/driver program for the function. This main program will call all the subfunctions: open_out_file.c open_in_file.c print_headers.c find_box_values.c THE FORMULAS (in algebraic notation)(must be translated to Cnotation): (1) the volume of a rectangular box. v=1*h*w (2) the surface area of a rectangular box. s_area = 2(1*w +h*w +h*1) INPUT/OUTPUT DESCRIPTION: The input is a list of varying length of type double values in the file lab5.dat. Each record (or line) will have three values for the three measures of the box. The data file will have four sets of values. The output is a chart showing the three measures of the box, the volume, and surface area. DEFINED OUTPUT APPEARANCE: Print statements included in the code, and require no changes, except for your name. Your Name, Lab5. Length Width Height Volume SurfaceArea ----- ------ --- 3.70 6.80 5.00 3.00 4.20 5.90 77.70 120.36 110.08 156.44 ONLY the two lines are shown here. You should validate the correctness of the other lines. ALGORITHM DEVELOPMENT - Pseudo code: /* ------ */ main /* main is given to you as lab5.c */ Call function open_out_file. Will open lab5.txt, mentioned in lab5.h. Call function open_in_file Will open lab5.dat, mentioned in lab5.h Call function print_headers Will print the column headers Call function find_box_values You write this one. Close the files. /* This code will reside in a file find_box_values.c /* In a separate file from the other functions */ // You need to do a #include of lab5.h void find_box_values(FILE *data_out, FILE *data_in, //fixed double I, double h, double w, double* vol, double*s_area) while( do an fscanf to read the I, w, h) ==3) 1 Calculate the *vol 1 Calculate the *s_area | Print the length, height, th, volume, surfaceArea print one more empty line. return /* -*/ REMINDERS: You should look at all the provided files and get an idea of what code each file contains. Remember to put your name and Lab 5 in the comment header of your function, in your makefile, and in the output. Remember the only operator for multiplication is the asterisk (*). You should examine the data file and confirm the correctness of the answer produced by your program
Step by Step Solution
There are 3 Steps involved in it
Step: 1
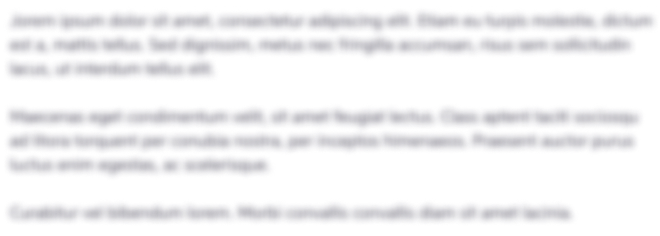
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started