Question
CODE IN C++ PLEASE AND WELL COMMENT THE PROGRAM. INCLUDE THE OUTPUT FOR THUMPS-UP! Lab: Use the following code to: Use OPERATOR OVERLOADING to modify
CODE IN C++ PLEASE AND WELL COMMENT THE PROGRAM. INCLUDE THE OUTPUT FOR THUMPS-UP!
Lab: Use the following code to:
Use OPERATOR OVERLOADING to modify CLOCKTYPE.
OVERLOAD the "= =" and "+=" (YOU may choose this, but clearly comment and document 1) What it does (2) what the Input is (3) What the Output is...
BE SURE TO ADD THE PROTOTYPE to the CLASS DEFINITION and to WRITE FULL-FUNCTION DEFINITIONS for both
#include
using namespace std;
class clockType
{
public:
void setTime(int, int, int);
void getTime(int&, int&, int&) const;
void printTime() const;
void incrementSeconds();
void incrementMinutes();
void incrementHours();
bool equalTime(const clockType&) const;
clockType();
clockType(int);
clockType(int, int);
clockType(int, int, int);
private:
int hr;
int min;
int sec;
};
int main()
{
//this program is to design and implement a class
//complete program that uses all the function types shown below
//using constructor to stop the program from printing garbage numbers
clockType myClock;
clockType yourClock;
int hours, hours2;
int minutes, minutes2;
int seconds, seconds2;
cout << "Default constructor for myClock:";
myClock.printTime();
cout << " Default constructor for yourClock:";
yourClock.printTime();
//set the time by calling the function
myClock.setTime(6, 5, 20);
cout << " Time of myClock: ";
myClock.printTime(); //print the time of myClock Line 3
cout << endl;
cout << "Time of yourClock: ";
yourClock.setTime(3, 2, 10);
yourClock.printTime(); //print the time of yourClock
cout << endl;
//Compare myClock and yourClock
if (myClock.equalTime(yourClock))
cout << "Both times are equal." << endl;
else
cout << "The two times are not equal." << endl;
cout << " Enter the hours, minutes, and "
<< "seconds of myClock: ";
cin >> hours >> minutes >> seconds;
//Set the time of myClock using the value of the
//variables hours, minutes, and seconds
myClock.setTime(hours, minutes, seconds);
cout << " New myClock: ";
myClock.printTime(); //print the time of myClock
cout << endl;
//Increment the time of myClock by one hour
myClock.incrementHours();
//Increment the time of myClock by one minute
myClock.incrementMinutes();
//Increment the time of myClock by one second
myClock.incrementSeconds();
cout << " After incrementing myClock by one hour, one minute and one second "
<< " myClock: ";
cout << " ";
myClock.printTime(); //print the time of myClock
cout << endl;
//Retrieve the hours, minutes, and seconds of the
//object myClock
myClock.getTime(hours, minutes, seconds);
//Output the value of hours, minutes, and seconds
cout << "hours = " << hours
<< ", minutes = " << minutes
<< ", seconds = " << seconds << endl;
cout << " Enter the hours, minutes, and "
<< "seconds of yourClock: " << endl;
cin >> hours2 >> minutes2 >> seconds2;
yourClock.setTime(hours2, minutes2, seconds2);
cout << " New yourClock: ";
yourClock.printTime(); //print the time of yourClock
cout << endl;
//increment the time of yourClock by one hour
yourClock.incrementHours();
//Increment the time of yourClock by one minute
yourClock.incrementMinutes();
//Increment the time of yourClock by one second
yourClock.incrementSeconds();
cout << " After incrementing yourClock by one hour, one minute and one second "
<< " yourClock: ";
yourClock.printTime(); //print the time of yourClock
yourClock.getTime(hours2, minutes2, seconds2);
//output the value of hours, minutes, and seconds of yourClock
cout << " hours = " << hours2
<< ", minutes = " << minutes2
<< ", seconds = " << seconds2 << endl;
cout << endl;
return 0;
}//end main
void clockType::setTime(int hours, int minutes, int seconds)
{
if (0 <= hours && hours < 24)
hr = hours;
else
hr = 0;
if (0 <= minutes && minutes < 60)
min = minutes;
else
min = 0;
if (0 <= seconds && seconds < 60)
sec = seconds;
else
sec = 0;
}
//Place the definitions of the remaining functions, getTime,
//incrementHours, incrementMinutes, incrementSeconds, printTime,
//and equalTime, of the class clockType, as described
//previously here.
void clockType::getTime(int& hours, int& minutes,
int& seconds) const
{
hours = hr;
minutes = min;
seconds = sec;
}
void clockType::printTime() const
{
if (hr < 10)
cout << "0";
cout << hr << ":";
if (min < 10)
cout << "0";
cout << min << ":";
if (sec < 10)
cout << "0";
cout << sec;
}
void clockType::incrementHours()
{
hr++;
if (hr > 23)
hr = 0;
}
void clockType::incrementMinutes()
{
min++;
if (min > 59)
{
min = 0;
incrementHours(); //increment hours
}
}
void clockType::incrementSeconds()
{
sec++;
if (sec > 59)
{
sec = 0;
incrementMinutes(); //increment minutes
}
}
bool clockType::equalTime(const clockType& otherClock) const
{
return (hr == otherClock.hr
&& min == otherClock.min
&& sec == otherClock.sec);
}
clockType::clockType() //default constructor
{
hr = -1;
min = -1;
sec = -1;
}
clockType::clockType(int hours)
{
if (0 <= hours && hours < 24)
hr = hours;
else
hr = 0;
}
clockType::clockType(int hours, int minutes)
{
if (0 <= minutes && minutes < 60)
min = minutes;
else
min = 0;
}
clockType::clockType(int hours, int minutes, int seconds)
{
if (0 <= seconds && seconds < 60)
sec = seconds;
else
sec = 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
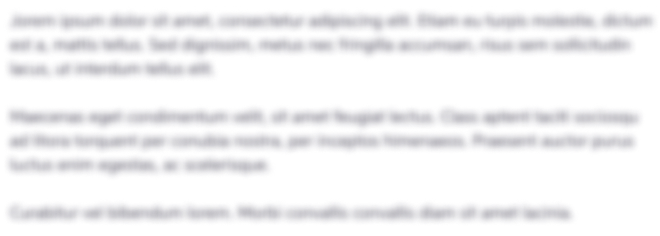
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started