Question
Code in c#. please keep in mind that I am a complete beginner. Review the code Open the InventoryMaintenance project You may need to set
Code in c#. please keep in mind that I am a complete beginner.
Review the code
Open the InventoryMaintenance project
You may need to set the property of the .txt file to "Content" and "Copy Always" or "Copy if newer" in the file's properties window
Display the code for the InvItemList class and note that it contains a readonly property named DisplayText.
Display the code for the Inventory Maintenance form and review the code that loads the items in the combo box and the code that executes when the selected item in the combo box is changed.
Run the application and view the items in the combo box. Notice that nothing happens when you change the selected item. Exit the application.
Add code that sorts and filters the inventory items
Find the FillItemListBox() method in the Inventory Maintenance form. It starts by storing the selected value of the combo box in a variable named filter and declaring a local collection of InvItem objects named filteredItems.
Code an if/else statement that uses LINQ to query the class-level collection named invItems based on the value of the filter variable. The LINQ queries should also order the inventory items by Description. Assign the result of each LINQ query to the filteredItems collection. Note: You can use method-based queries or query expressions here.
Update the foreach statement so it loops through the local filteredItems collection rather than the class-level invItems collection.
Test the application to be sure it displays the items in alphabetical order and filters the items by price when the selected item in the combo box changes.
Add the following new item to the inventory: ItemNo: 4372639 Description: Creeping Phlox Price: 24.99 Notice that the new item is sorted so it appears alphabetically in the display. Use the combo box to show items whose price is between $10 and $50 and see that the new item is displayed. When youre done, exit the application.
Open the InventoryItems.txt file and see that the items arent in alphabetical order, and that the item you just added is the last item in the file
Add code that uses LINQ to find the inventory item to delete
Run the application, select the item you added and click Delete. Note that the confirmation message identifies the wrong item for deletion. Click Cancel and exit the application.
Display the btnDelete_Click() event handler in the Inventory Maintenance form and comment out the line of code that uses the selected index value of the ListView control to get the selected InvItem object from the invItems collection.
Add code that uses that selected index value to retrieve the display text of the selected item from the Items collection of the ListView control.
Use a LINQ query to get the selected InvItem object from the InvItems collection. The LINQ query should select the item whose DisplayText property is equal to the display text value you just retrieved. Note: To do this, you need to use the First() or FirstOrDefault() method. Because of that, you should code this as a method-based query.
Run the application and try to delete the item you added in step 4. This time, the confirm message should present the correct inventory item. Click OK to delete the item and exit the application
=================
Here is what I have ==================
frmInvMaint.cs
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms;
namespace InventoryMaintenance { public partial class frmInvMaint : Form { public frmInvMaint() { InitializeComponent(); }
private List
private void frmInvMaint_Load(object sender, EventArgs e) { invItems = InvItemDB.GetItems(); LoadComboBox(); FillItemListBox(); }
private void LoadComboBox() { cboFilterBy.DataSource = new string[] { "All", "Under $10", "$10 to $50", "Over $50" }; }
private void FillItemListBox() { lstItems.Items.Clear(); string filter = cboFilterBy.SelectedValue.ToString(); IEnumerable
// add items to the filteredItems collection based on FilterBy value // change code to loop the filteredItems collection foreach (InvItem item in invItems) { lstItems.Items.Add(item.DisplayText); } }
private void btnAdd_Click(object sender, EventArgs e) { frmNewItem newItemForm = new frmNewItem(); InvItem invItem = newItemForm.GetNewItem(); if (invItem != null) { invItems.Add(invItem); InvItemDB.SaveItems(invItems); FillItemListBox(); } }
private void btnDelete_Click(object sender, EventArgs e) { int i = lstItems.SelectedIndex; if (i != -1) { InvItem invItem = (InvItem)invItems[i]; string message = $"Are you sure you want to delete {invItem.Description}?"; DialogResult button = MessageBox.Show(message, "Confirm Delete", MessageBoxButtons.YesNo); if (button == DialogResult.Yes) { invItems.Remove(invItem); InvItemDB.SaveItems(invItems); FillItemListBox(); } } }
private void btnExit_Click(object sender, EventArgs e) { this.Close(); }
private void cboFilterBy_SelectedIndexChanged(object sender, EventArgs e) { FillItemListBox(); } } }
frmInvMaint.resx
frmInvMaint.Designer.cs
namespace InventoryMaintenance { partial class frmInvMaint { ///
///
#region Windows Form Designer generated code
///
}
#endregion
private System.Windows.Forms.ListBox lstItems; private System.Windows.Forms.Button btnAdd; private System.Windows.Forms.Button btnDelete; private System.Windows.Forms.Button btnExit; private System.Windows.Forms.ComboBox cboFilterBy; private System.Windows.Forms.Label label2; } }
frmNewItem.cs
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Text; using System.Windows.Forms;
namespace InventoryMaintenance { public partial class frmNewItem : Form { public frmNewItem() { InitializeComponent(); }
private InvItem invItem = null;
public InvItem GetNewItem() { this.ShowDialog(); return invItem; }
private void btnSave_Click(object sender, EventArgs e) { if (IsValidData()) { invItem = new InvItem( Convert.ToInt32(txtItemNo.Text), txtDescription.Text, Convert.ToDecimal(txtPrice.Text) ); this.Close(); } }
private bool IsValidData() { return Validator.IsPresent(txtItemNo) && Validator.IsInt32(txtItemNo) && Validator.IsPresent(txtDescription) && Validator.IsPresent(txtPrice) && Validator.IsDecimal(txtPrice); }
private void btnCancel_Click(object sender, EventArgs e) { this.Close(); } } }
frmNewItem.resx
frmNewItem.Designer.cs
namespace InventoryMaintenance { partial class frmNewItem { ///
///
#region Windows Form Designer generated code
///
}
#endregion
private System.Windows.Forms.Label label1; private System.Windows.Forms.TextBox txtItemNo; private System.Windows.Forms.Label label2; private System.Windows.Forms.TextBox txtDescription; private System.Windows.Forms.Label label3; private System.Windows.Forms.TextBox txtPrice; private System.Windows.Forms.Button btnSave; private System.Windows.Forms.Button btnCancel; } }
InventoryItems.txt
3245649|Agapanthus|7.95 3762592|Limonium|6.95 9210584|Snail pellets|12.95 4738459|Japanese Red Maple|89.95
InvItem.cs
using System; using System.Collections.Generic; using System.Text;
namespace InventoryMaintenance { public class InvItem { public InvItem() { }
public InvItem(int itemNo, string description, decimal price) { ItemNo = itemNo; Description = description; Price = price; }
public int ItemNo { get; set; } public string Description { get; set; } public decimal Price { get; set; }
public string DisplayText => $"{ItemNo} {Description} ({Price:c})"; } }
InvItemDB.cs
using System; using System.Collections.Generic; using System.IO; using System.Text; using System.Xml;
namespace InventoryMaintenance { public static class InvItemDB { private const string Path = @"..\..\..\InventoryItems.txt"; private const string Separator = "|";
public static List
// create the object for the input stream for a text file StreamReader textIn = new StreamReader( new FileStream(Path, FileMode.OpenOrCreate, FileAccess.Read));
// read the data from the file and store it in the list while (textIn.Peek() != -1) { string row = textIn.ReadLine(); string[] columns = row.Split(Separator); InvItem item = new InvItem(); item.ItemNo = Convert.ToInt32(columns[0]); item.Description = columns[1]; item.Price = Convert.ToDecimal(columns[2]); items.Add(item); }
// close the input stream for the text file textIn.Close();
return items; }
public static void SaveItems(List
// write each item foreach (InvItem item in items) { textOut.Write(item.ItemNo + Separator); textOut.Write(item.Description + Separator); textOut.WriteLine(item.Price); }
// close the output stream for the text file textOut.Close(); } } }
Program.cs
using System; using System.Collections.Generic; using System.Linq; using System.Threading.Tasks; using System.Windows.Forms;
namespace InventoryMaintenance { static class Program { ///
Validator.cs
using System; using System.Collections.Generic; using System.Text; using System.Windows.Forms;
namespace InventoryMaintenance { public static class Validator { private static string title = "Entry Error"; public static string Title { get => title; set => title = value; }
public static bool IsPresent(TextBox textBox) { if (textBox.Text == "") { MessageBox.Show(textBox.Tag + " is a required field.", Title); textBox.Focus(); return false; } return true; }
public static bool IsDecimal(TextBox textBox) { decimal number = 0m; if (Decimal.TryParse(textBox.Text, out number)) { return true; } else { MessageBox.Show(textBox.Tag + " must be a decimal value.", Title); textBox.Focus(); return false; } }
public static bool IsInt32(TextBox textBox) { int number = 0; if (Int32.TryParse(textBox.Text, out number)) { return true; } else { MessageBox.Show(textBox.Tag + " must be an integer.", Title); textBox.Focus(); return false; } }
public static bool IsWithinRange(TextBox textBox, decimal min, decimal max) { decimal number = Convert.ToDecimal(textBox.Text); if (number < min || number > max) { MessageBox.Show(textBox.Tag + " must be between " + min + " and " + max + ".", Title); textBox.Focus(); return false; } return true; } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
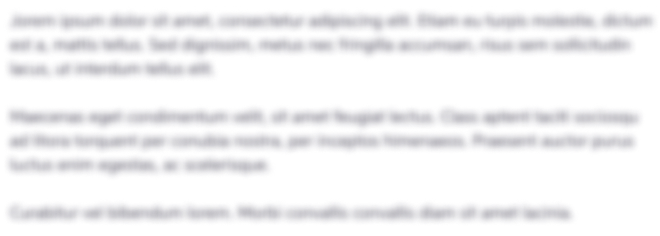
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started