Question
CODE IN C++. You are to implement and test a Queue template class. The class should use a linked list as its underlying representation. This
CODE IN C++.
You are to implement and test a Queue template class. The class should use a linked list as its underlying representation. This assignment is worth 6% of your grade. Please read the requirements carefully, paying particular attention to the names and input and output requirements of the class and its methods. We will be testing your class in our program, so if you do not follow the requirements the test program will not compile and will not be marked. Queue Class Class Description Your class should be named QueueT and should support these operations:
Creating an empty queue
Inserting a value Removing a value
Finding the size of the queue
Printing the contents of the queue
Adding the contents of one queue to the end of another Merging the contents of two queues into a third, new, queue Class Attributes Your class should be implemented using a linked list and should have the following private member variables
A pointer to a NodeT that represents the front of the queue A pointer to a NodeT that represents the back of the queue
An int that records the current size of the queue (i.e. the number of values in the queue) Queue Public Methods You must implement a QueueT template class to store data of any type. The queue must be implemented using a singly linked list of Nodes, where NodeT is a class that you also implement. Note that the enqeue and deqeue methods should be implemented in constant time. The QueueT class should implement the following public methods:
constructor creates an empty queue
copy constructor a constructor that creates a deep copy of its constant QueueT reference parameter
destructor deallocates dynamic memory allocated by the queue
operator= overloads the assignment operator for QueueT (deep) copies its constant QueueT reference parameter into the calling object and returns a reference to the calling object after de-allocating any dynamic memory associated with the original contents of the calling object; if the calling object is equal to the parameter the operator should not copy it
enqueue inserts its template type parameter at the back of the queue dequeue removes and returns the value at the front of the queue; if the queue is empty throws a runtime_error (this error class is defined in the stdexcept library file)
print prints the contents of the queue, one value per line, from front to back
empty returns true if the queue is empty, false otherwise size returns the number of items stored in the queue
concatenate has two parameters, a QueueT reference and an integer (referred to as n in this description) adds the first n values stored in its QueueT parameter to the end of the calling object, the resulting queue therefore contains its original contents order unchanged followed by the first n values of the parameter queue in their original order; the values added to the calling object should be removed from the parameter; both queue's sizes should be adjusted as appropriate; if n is greater than the size of the parameter a runtime_error should be thrown and no changes made to either queue
merge returns a QueueT object that contains the contents of the calling object and its constant QueueT reference parameter, the resulting queue should start with the first value in the calling object, followed by the first value in the parameter, subsequent values should be copied in order from the two queues alternating between them; no changes should be made to either the calling object or the parameter; example: calling object = {a,b,c,d,e}, parameter = {r,s,t}, result = {a,r,b,s,c,t,d,e}
getFront returns a pointer to the node at the front of the queue. This method is provided to you here: NodeT* getFront() { return front; }; Its purpose is to allow us to directly access your queue's linked list for testing. It is not something a working class should include since allowing access to the internal structure of a class is not good design. Note that if you called your pointer to the node at the front of the queue something other than "front" you will need to change the method given above to return the correct attribute. Additional Notes The calling object should be made constant for any method where the calling object's attribute values should not change
You may implement helper methods if you wish (for example, you might want to implement a deep copy method that can be used by the copy constructor and the overloaded assignment operator)
Your class may include other private attributes that you deem necessary Method parameters and return values are noted (and highlighted) in the method descriptions you must not add additional parameters to the methods; if the method description does not mention a parameter it does not have one, similarly if no return value is mentioned the method is void (or a constructor or destructor)
The parameter type for enqeue and the return type of deqeue should be your template variable see the implementation notes near the end of this document.
CMPT 225 Assignment 2 Queue You are to implement and test a Queue template class. The class should use a linked list as its underlying representation. This assignment is worth 6% of your grade. Please read the requirements carefully, paying particular attention to the names and input and output requirements of the class and its methods. We will be testing your class in our program, so if you do not follow the requirements the test program will not compile and will not be marked. Queue Class Class Description Your class should be named QueueT and should support these operations: . Creating an empty queue Inserting a value . Removing a value . Finding the size of the queue Printing the contents of the queue Adding the contents of one queue to the end of another Merging the contents of two queues into a third, new, queue Class Attributes Your class should be implemented using a linked list and should have the following private member variables . A pointer to a NodeT that represents the front of the queue . A pointer to a NodeT that represents the back of the queue . An int that records the current size of the queue (i.e. the number of values in the queue) Queue Public Methods You must implement a Queuer template class to store data of any type. The queue must be implemented using a singly linked list of Nodes, where NodeT is a class that you also implement. Note that the enqeue and deqeue methods should be implemented in constant time. The Queue T class should implement the following public methods: . constructor - creates an empty queue . copy constructor - a constructor that creates a deep copy of its constant QueueT reference parameter . destructor-deallocates dynamic memory allocated by the queue operator= - overloads the assignment operator for QueueT - (deep) copies its constant QueueT reference parameter into the calling object and returns a reference to the callingStep by Step Solution
There are 3 Steps involved in it
Step: 1
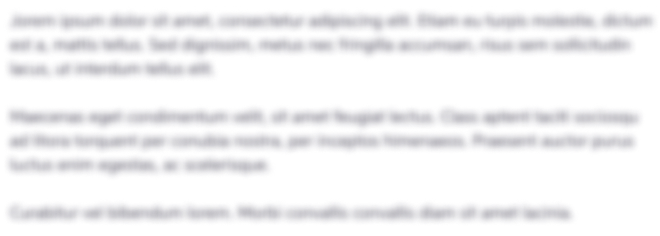
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started