Question
Code in JAVA You are asked to implement Connect 4 which is a two player game. The game will be demonstrated in class. The game
Code in JAVA
You are asked to implement Connect 4 which is a two player game. The game will be demonstrated in class. The game is played on a 6x7 grid, and is similar to tic-tac-toe, except that instead of getting three in a row, you must get four in a row. To take your turn, you choose a column, and slide a checker of your color into the column that you choose. The checker drops into the slot, falling either all the way to the bottom, or resting on top of the highest checker already in that column. You may not place a checker into a column that is full. You win when you create a row, column or diagonal of four in a row of your color.
Your project should have at least three classes: Cell, GameBoard, Connect4Game and GameDriver.
The Cell class will represent a single location in the game board. It should contain at least the following:
Instance variable:
- A private variable that indicates whether the location on the game board is empty, holds a red checker, or holds a blue checker. The variable should be of an enumerated type called State, that you define.
Methods:
- A constructor that takes one argument of type State. This constructor should use its parameter to set the instance variable.
- An public accessor (getter) method for the instance variable.
- A public mutator (setter) method for the instance variable.
The GameBoard class holds the information about the entire game board:
Instance variable:
- A private two-dimensional array of cells.
Methods:
- A no-argument constructor, that does the necessary initialization of StdDraw. The constructor should initialize the game board to be a 6 by 7 array of Cells, all of which are set to be empty. The constructor should also use StdDraw.setCanvasSize(), StdDraw.setXscale(), and StdDraw.setYscale(). You may choose the default size on the screen of the game board.
- A constructor with one argument, which is the number of pixels in the height of the game board. This constructor should also do the same necessary initialization as the no-argument constructor.
- A public boolean method called columnFull that takes a column number as an argument, and returns true if the column is full and false if the column still has space.
- A public boolean method called addChecker that takes a column and a State, and adds that color checker to the game board. The method returns false if the column was already full, and true otherwise.
- A public void method called drawGameBoard that displays the game board in its current state. The board should basically look like a Connect 4 game board, but you're free to be artistic.
- A public boolean method called GameOver that returns true of the game is finished. It takes as parameters the row and column position of the last checker that has been played, and checks to see if there are four in a row of that same color going vertically, horizontally or diagonally through that position.
The class Connect4Game should have
- an instance variable of type GameBoard
- A no-argument constructor that does all necessary initialization
- A public void method called playGame that starts interaction with users, manages turn-taking, gets input from users via the keyboard and console, and controls display of the game board. When taking input from the user, check for validity of the input, and continue asking until valid input is given. Create private methods within this class to divide up the work in an organized way and makes your code easier to read.
The class GameDriver should have a main method that creates a Connect4Game object and calls its playGame method.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
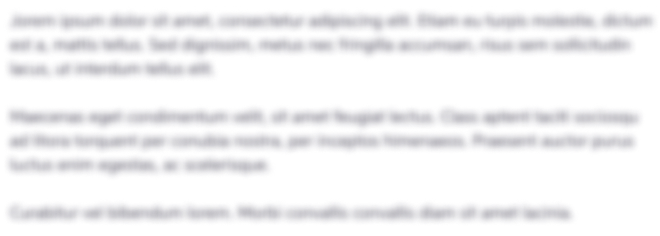
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started