Question
Code must be written in Python 3 please. Also, I'd love it if the bonus questions were completed too. All functions must include a docstring
Code must be written in Python 3 please. Also, I'd love it if the bonus questions were completed too.
All functions must include a docstring comment indicating what the function does.
For this program, you will use the file called cities_by_continent.csv (under Resources). You will note that each line in this file contains a continent, followed by a few major cities from that continent. In this assignment you will do the following:
1.Read in all the continents into a dictionary. The key for each item in the dictionary should be the continent name. The value for that key should be a list containing the cities of that continent So for example, once you have read in the dictionary, if you were to print it (and you should!!), it would look like this:
{'Europe': ['Paris', 'London', 'Berlin'], 'Asia': ['Tokyo', 'Beijing', 'Seoul'], 'South America': ['Caracas', 'Lima', 'Willemstad'], 'North America': ['Ottawa', 'Mexico City', 'San Jose']}
Small bonus challenge (5 points): Have the cities appear in a tuple instead of a list.
Do this step in a function called . This function should return the dictionary that you created.
2.Now create a function called . This function should accept one argument, namely, the dictionary that was created in step 1. The function should examine that dictionary, and should print out all of the continents. The function should then ask the user to choose a continent.
Because it is the end of the quarter, we will keep it simple and assume assume that the user types in a valid continent name, and with the proper case.
Bonus (5 points): Loop until the user provides a valid continent name. Also, allow the user to enter any case and it will still work.
The function should return the name of the continent the user entered.
3.Have a function called that accepts 2 arguments: The dictionary we have been working with, and the continent choice the user selected in step 2. Using a format string, output the following type of statement. (Lets pretend the user typed Asia for their continent):
Some important cities in Asia include: ['Tokyo', 'Beijing', 'Seoul']
Bonus (5 points): Remove the cities from the list, so that it outputs:
Some important cities in Asia include Tokyo, Beijing, Seoul.
i.e. Note that the square brackets and quotes are removed. If you do this bonus, you may struggle with removing the last comma. Dont spend too much time worrying about that. You will still get the bonus credit.
SO
Your code should consist of 3 functions, and then a series of function calls. The outline of your module will be something like this:
def generateCities():
Your code here..
def getContinentChoice(the_dictionary):
Your code here..
def printCities(the_dictionary,the_users_choice):
Your code here..
dctCities = generateCities()
userContinentchoice = getContinentChoice(dctCities)
printCities(dctCities,userContinentChoice)
The 'cities_by_continent.csv' file is formatted exactly like this:
Europe,Paris,London,Berlin Africa,Cairo,Tripoli,Rabat Asia,Tokyo,Beijing,Seoul South America,Caracas,Lima,Willemstad North America,Ottawa,Mexico City,San Jose
Step by Step Solution
There are 3 Steps involved in it
Step: 1
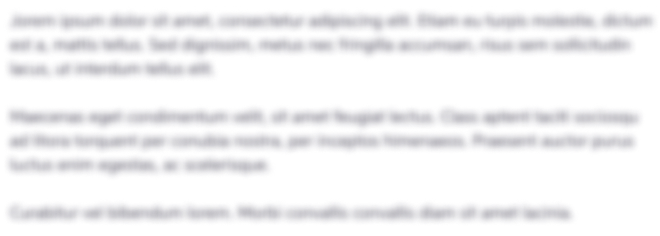
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started