Question
Code to open the file (colors.txt) is already provided. It also reads the number of colors from the file (4) and uses that to initialize
Code to open the file (colors.txt) is already provided. It also reads the number of colors from the file (4) and uses that to initialize colorfulPalette to be of the correct size. Add the following:
Fill in function readColor() to read in one color from the file stream passed, and add it to the palette. Each color is on its own line, e.g.: RGB 255 0 0 HSV 60 91 84 Use the following algorithm: Read the type of color (RGB or HSV). If its RGB, read in the red, green, and blue components, create an anonymous object of type RgbColor and pass it to addColor() of the palette. If its instead HSV, read in the hue, saturation, and value, create an anonymous object of type HsvColor and pass that to addColor() of palette. Return whether the read was successful (true/false) so that the reading loop in main() works.
Relevant Code:
// Read one color (RGB or HSV) from the file stream and add it to the palette. // Report back whether a color could be read. bool readColor(istream& colorStream, Palette& palette);
int main(int argc, char* args[]) { // Open file containing colors (RGB or HSV). const char COLOR_FILENAME[] = "colors.txt"; ifstream colorStream(COLOR_FILENAME);
// Read number of colors in file and make palette of appropriate size. int maxColors; colorStream >> maxColors; Palette colorfulPalette(maxColors);
// Read each color from file stream. while (readColor(colorStream, colorfulPalette)) ;
// Make copy of color palette, then convert all its colors to grayscale. Palette grayPalette(colorfulPalette); grayPalette.convertToGrayscale();
// Current palette used to display image (initially the colorful version). // Realistically, should just point to the palette to use, but we need to // test copying/assignment. Palette palette(colorfulPalette);
// Allow user to enter commands to choose color palette used to display image. char option;
do { // Display image translating indexed color to colors from palette. drawImage(screenSurface, image, IMAGE_HEIGHT, IMAGE_WIDTH, palette);
// Update the surface to display color. SDL_UpdateWindowSurface(window);
// Allow user to enter commmands to test color palettes. cout << endl; cout << "Preview image using which palette?" << endl; cout << "c)olor" << endl; cout << "g)rayscale" << endl; cout << "ex)it" << endl; cout << "Palette: "; cin >> option;
switch (option) { case 'c': // color palette = colorfulPalette; break;
case 'g': // grayscale palette = grayPalette; break;
case 'x': // exit break;
default: cout << "Unknown palette: " << option << endl; break; } } while (option != 'x'); // exit?
return 0; }
// Read one color (RGB or HSV) from the file stream and add it to the palette. // Report back whether a color could be read. bool readColor(istream& colorStream, Palette& palette) { string type; // type of color ("RGB" or "HSV")
// FILL IN FUNCTION.
return false; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
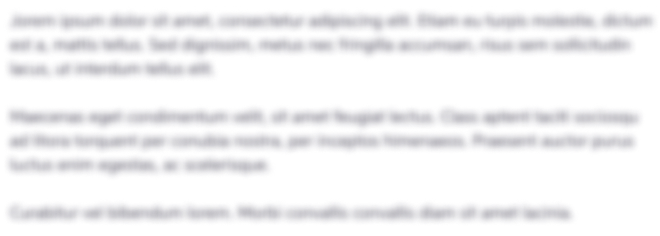
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started