Question
CODE USING C++ PROGRAMMING LANGUAGE --------------------------------------------------------------------- DO NOT USE any of the STL (including the stack and queue type) in your code. ------------------------------------------------------------------------------------------------------------ SongHeap Implement
CODE USING C++ PROGRAMMING LANGUAGE ---------------------------------------------------------------------
DO NOT USE any of the STL (including the stack and queue type) in your code.
------------------------------------------------------------------------------------------------------------
SongHeap
Implement a min-heap using a dynamic array implementation. The class should be named SongHeap, and it uses the csv dataset provided below (Billboard Top 100). Write all basic class functions (default and copy constructors, destructor, copy assignment).
SongHeap will hold information about each song including the dates it appeared on the Billboard chart. These dates will be stored in a static array dates, and frequency indicates how many times the song appeared on the chart in 2020.
SongHeap has a data member songList, which is an array of Songs. Set the initial size of songList to 5, and double the size as needed. The song title acts as a key, and smaller keys have higher priority (ex. A before B).
struct Song{ string title; //used as key string artist; string dates[52]; //filled starting from index 0 int frequency; //should match # of elements in dates };
Reading in Songs
The list of songs to add to the tree will be in top.csv . Discard the first line of the file in the driver, then read in each song. Pass all the information to the insert function to add each song to the tree.
------------------------------------------------------------------------------------------------------------
Heap Functions
Implement the following functions: 1. void insert(string title, string artist, string date). You should first check all the songs in songList to see if the song is already in the heap. If so, update the frequency and dates of the Song. Otherwise, create and add a Song to the heap (i.e., songList). The heap should grow as needed.
2. string deleteMin(): deletes the Song with the min key and returns its title. 3. print(): prints all the songs in the heap using the following format arr[i]: title (See the execution example). 4.printSong(string title): finds and prints all the information about the song.
------------------------------------------------------------------------------------------------------------
The SongHeap class should be in two files named songheap.h and songheap.cpp. The driver should be in a file named main.cpp.
------------------------------------------------------------------------------------------------------------
Execution example ------------------------------------------------------------------------------------------------------------ Hello! Processing the first 30 lines of top1.csv file. The array is full. Resizing array to 10 elements. The array is full. Resizing array to 20 elements.
Printing tree: arr[1] = All I Want For Christmas Is You arr[2] = Blinding Lights arr[3] = Say So arr[4] = Circles arr[5] = Rain On Me arr[6] = The Scotts arr[7] = The Box arr[8] = Stuck With U arr[9] = Savage arr[10] = Toosie Slide arr[11] = Rockstar arr[12] = Trollz
Which song do you want to print? Blinding Lights
Title: Blinding Lights Artist: The Weeknd Appeared on the Billboard chart 4 times on: 2020-04-11 2020-04-18 2020-05-02 2020-05-09
Which song do you want to print? Testing Testing is not in the heap.
Removing the min value. "All I Want For Christmas Is You" has been removed. Printing the updated heap:
arr[1] = Blinding Lights arr[2] = Circles arr[3] = Say So arr[4] = Savage arr[5] = Rain On Me arr[6] = The Scotts arr[7] = The Box arr[8] = Stuck With U arr[9] = Trollz arr[10] = Toosie Slide arr[11] = Rockstar
Removing the min value. "Blinding Lights" has been removed. Printing the updated heap:
arr[1] = Circles arr[2] = Rain On Me arr[3] = Say So arr[4] = Savage arr[5] = Rockstar arr[6] = The Scotts arr[7] = The Box arr[8] = Stuck With U arr[9] = Trollz arr[10] = Toosie Slide
This is the end of the execution example. Goodbye!
CSV FILE: top.csv
title,artist,date All I Want For Christmas Is You,Mariah Carey,2020-01-04 All I Want For Christmas Is You,Mariah Carey,2020-01-11 Circles,Post Malone,2020-01-18 The Box,Roddy Ricch,2020-01-25 The Box,Roddy Ricch,2020-02-01 The Box,Roddy Ricch,2020-02-08 The Box,Roddy Ricch,2020-02-15 The Box,Roddy Ricch,2020-02-22 The Box,Roddy Ricch,2020-02-29 The Box,Roddy Ricch,2020-03-07 The Box,Roddy Ricch,2020-03-14 The Box,Roddy Ricch,2020-03-21 The Box,Roddy Ricch,2020-03-28 The Box,Roddy Ricch,2020-04-04 Blinding Lights,The Weeknd,2020-04-11 Blinding Lights,The Weeknd,2020-04-18 Toosie Slide,Drake,2020-04-25 Blinding Lights,The Weeknd,2020-05-02 Blinding Lights,The Weeknd,2020-05-09 The Scotts,"THE SCOTTS - Travis Scott & Kid Cudi",2020-05-16 Say So,Doja Cat Featuring Nicki Minaj,2020-05-23 Stuck With U,Ariana Grande & Justin Bieber,2020-05-30 Savage,Megan Thee Stallion,2020-06-06 Rain On Me,Lady Gaga & Ariana Grande,2020-06-13 Rockstar,DaBaby Featuring Roddy Ricch,2020-06-20 Rockstar,DaBaby Featuring Roddy Ricch,2020-06-27 Trollz,6ix9ine & Nicki Minaj,2020-07-04 Rockstar,DaBaby Featuring Roddy Ricch,2020-07-11 Rockstar,DaBaby Featuring Roddy Ricch,2020-07-18 Rockstar,DaBaby Featuring Roddy Ricch,2020-07-25 Rockstar,DaBaby Featuring Roddy Ricch,2020-08-01 Rockstar,DaBaby Featuring Roddy Ricch,2020-08-08 Cardigan,Taylor Swift,2020-08-15 Watermelon Sugar,Harry Styles,2020-08-22 WAP,Cardi B Featuring Megan Thee Stallion,2020-08-29 WAP,Cardi B Featuring Megan Thee Stallion,2020-09-05 Dynamite,BTS,2020-09-12 Dynamite,BTS,2020-09-19 WAP,Cardi B Featuring Megan Thee Stallion,2020-09-26 WAP,Cardi B Featuring Megan Thee Stallion,2020-10-03 Dynamite,BTS,2020-10-10 Franchise,Travis Scott Featuring Young Thug & M.I.A.,2020-10-17 Savage Love (Laxed - Siren Beat),Jawsh 685 x Jason Derulo,2020-10-24 Mood,24kGoldn Featuring iann dior,2020-10-31 Mood,24kGoldn Featuring iann dior,2020-11-07 Positions,Ariana Grande,2020-11-14 Mood,24kGoldn Featuring iann dior,2020-11-21 Mood,24kGoldn Featuring iann dior,2020-11-28 Mood,24kGoldn Featuring iann dior,2020-12-05 Life Goes On,BTS,2020-12-12 Mood,24kGoldn Featuring iann dior,2020-12-19 All I Want For Christmas Is You,Mariah Carey,2020-12-26
Step by Step Solution
There are 3 Steps involved in it
Step: 1
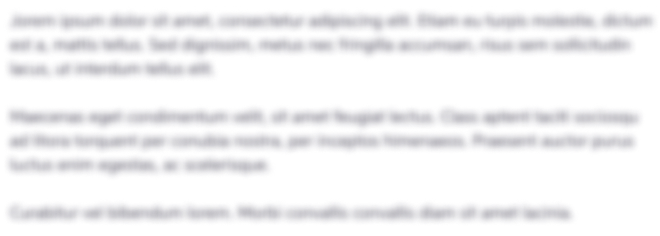
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started