Question
Coding Assignment in Java (Prior INFO) Focus of the Assignment on Programming Techniques This assignment will focus on creating a immutable class, where once an
Coding Assignment in Java
(Prior INFO)
Focus of the Assignment on Programming Techniques
This assignment will focus on
creating a immutable class, where once an object has been created the data in the object cannot be altered, only accessed.
input from a large text data file where each line of will be a single record of data. The data from each record will be used to make a new object of the immutable class and will then be stored in an ArrayList.
use of a try-with-resources for reading the data in the large data file.
using ArrayLists of objects.
A good solution to this assignment will take advantage of the idea that ArrayLists only contain references to objects by making use of multiple ArrayLists that reference smaller and smaller subsets of the same set of objects.
I. Programming Assignment Overview
Institutions of higher education in the United States are required by law to track campus safety issues, including rates of various crimes. This data is gathered and made available in various forms via such sites as https://www.data.gov/. This programming project will use real world data about the frequency of serious crimes against persons occurring on campuses of higher education in the United States in the years 2014, 2015 and 2016. Your project will read the data from a provided text file, storing records (each record unique by school and year) in objects of a class of your own creation in an ArrayList, then use that data to analyze the data to provide reports based on user input.
Description of the Data in the File oncampuscrime.txt
Each line of data is the file is one unique record containing information about serious crimes against persons occurring in one year (2014, 2015 or 2016) on a campus of an institution of higher education in the United States. There are over 30,000 records in the file. Each record will consist of the following data values, each item of data separated by a space:
the year the state institution or campus type number of male students number of female students number of murders umber of negligent homicides - integer; -1 indicates data not available, otherwise the data is >= zero number of rapes - integer; -1 indicates data not available, otherwise the data is >= zero number of aggravated assaults- integer; -1 indicates data not available, otherwise the data is >= zero
Example of Data from the File oncampuscrime.txt
2016 MA 2 15290 14618 0 0 1 0 2014 FL 3 418 538 0 0 0 0 2015 GA 4 432 1131 1 1 1 1 2014 MD 1 1803 2515 0 0 0 0 2015 MN 9 0 103 0 0 0 0
- integer consisting of 2014, 2015 or 2016 - string consisting of the USPS abbreviation for a state - integer from 1 to 9 inclusive (equivalence list given below) - positive integer - positive integer - integer; -1 indicates data not available, otherwise the data is >= zero
2016 CT 2 695 1170 0 0 7 2
List of USPS State Abbreviations
AL, AK, AZ, AR, CA, CO, CT, LA, ME, MD, MA, MI, MN, MS, OH, OK, OR, PA, RI, SC, SD,
DE, FL, GA, HI, ID, IL, IN, IA, KS, KY, MO, MT, NE, NV, NH, NJ, NM, NY, NC, ND, TN, TX, UT, VT, VA, WA, WV, WI, WY
CMPS 260 Spring 2018 Programming Assignment #7 (2018-04-10) 3
List of Institution or Campus Types
1 public, 4 year or above
2 private nonprofit, 4 year or above
3 private for profit, 4 year or above
4 public, 2 year
5 private nonprofit, 2 year
6 private for profit, 2 year
7 public, less than 2 year
8 private nonprofit, less than 2 year
9 private for profit, less than 2 year
PROBLEM!!!!!
How would I make CampusClassAnalysis.java an immutable class and make the main.java correspond.
CampusClassAnalysis.java
public class CampusCrimeAnalysis {
private int year;
private String state;
private int campusType;
private int maleStudentCount;
private int femaleStudentCount;
private int murderCount;
private int negligentHomicidesCount;
private int rapeCount;
private int aggravatedAssaultsCount;
/**
*
* @return the year
*
*/
public int getYear() {
return year;
}
/**
*
* @param year
* the year to set
*
*/
public void setYear(int year) {
this.year = year;
}
/**
*
* @return the state
*
*/
public String getState() {
return state;
}
/**
*
* @param state
* the state to set
*
*/
public void setState(String state) {
this.state = state;
}
/**
*
* @return the campusType
*
*/
public int getCampusType() {
return campusType;
}
/**
*
* @param campusType
* the campusType to set
*
*/
public void setCampusType(int campusType) {
this.campusType = campusType;
}
/**
*
* @return the maleStudentCount
*
*/
public int getMaleStudentCount() {
return maleStudentCount;
}
/**
*
* @param maleStudentCount
* the maleStudentCount to set
*
*/
public void setMaleStudentCount(int maleStudentCount) {
this.maleStudentCount = maleStudentCount;
}
/**
*
* @return the femaleStudentCount
*
*/
public int getFemaleStudentCount() {
return femaleStudentCount;
}
/**
*
* @param femaleStudentCount
* the femaleStudentCount to set
*
*/
public void setFemaleStudentCount(int femaleStudentCount) {
this.femaleStudentCount = femaleStudentCount;
}
/**
*
* @return the murderCount
*
*/
public int getMurderCount() {
return murderCount;
}
/**
*
* @param murderCount
* the murderCount to set
*
*/
public void setMurderCount(int murderCount) {
this.murderCount = murderCount;
}
/**
*
* @return the negligentHomicidesCount
*
*/
public int getNegligentHomicidesCount() {
return negligentHomicidesCount;
}
/**
*
* @param negligentHomicidesCount
* the negligentHomicidesCount to set
*
*/
public void setNegligentHomicidesCount(int negligentHomicidesCount) {
this.negligentHomicidesCount = negligentHomicidesCount;
}
/**
*
* @return the rapeCount
*
*/
public int getRapeCount() {
return rapeCount;
}
/**
*
* @param rapeCount
* the rapeCount to set
*
*/
public void setRapeCount(int rapeCount) {
this.rapeCount = rapeCount;
}
/**
*
* @return the aggravatedAssaultsCount
*
*/
public int getAggravatedAssaultsCount() {
return aggravatedAssaultsCount;
}
/**
*
* @param aggravatedAssaultsCount
* the aggravatedAssaultsCount to set
*
*/
public void setAggravatedAssaultsCount(int aggravatedAssaultsCount) {
this.aggravatedAssaultsCount = aggravatedAssaultsCount;
}
}
Main.java
public class Main {
private static String inputFileName = "oncampuscrime.txt";
private static String crimeTypeList = "Murder,NegHom,Rape,AgAslt,All";
private static String yearList = "2014,2015,2016,All";
private static String campusTypeList = "0-All,1-Pub4yr,2-PriNoProf,3-PriProf4yr,4-Pub2yr,"
+ "5-PriNoProf2yr,6-PriProf2yr,7-Pub<2yr,8-PriNoProf<2yr,9-PriProf<2yr";
private static String stateAbbr = "AL,AK,AZ,AR,CA,CO,CT,DE,FL,GA,HI,ID,IL,IN,IA,KS,KY,LA,ME,MD,MA,MI,MN,MS,MO,MT,NE,NV,NH,NJ,NM,NY,NC,ND,OH,OK,OR,PA,RI,SC,SD,TN,TX,UT,VT,VA,WA,WV,WI,WY";
public static void main(String[] args) throws IOException {
Scanner scanner = new Scanner(new File(inputFileName));
ArrayList
// reading data from file and creating a list of object storing each
// record in the file
while (scanner.hasNext()) {
String[] inputLineList = scanner.nextLine().split(" ");
if (inputLineList.length != 9) {
continue;
}
CampusCrimeAnalysis campusCrimeAnalysis = new CampusCrimeAnalysis();
campusCrimeAnalysis.setYear(Integer.parseInt(inputLineList[0]));
campusCrimeAnalysis.setState(inputLineList[1]);
campusCrimeAnalysis.setCampusType(Integer.parseInt(inputLineList[2]));
campusCrimeAnalysis.setMaleStudentCount(Integer.parseInt(inputLineList[3]));
campusCrimeAnalysis.setFemaleStudentCount(Integer.parseInt(inputLineList[4]));
campusCrimeAnalysis.setMurderCount(Integer.parseInt(inputLineList[5]));
campusCrimeAnalysis.setNegligentHomicidesCount(Integer.parseInt(inputLineList[6]));
campusCrimeAnalysis.setRapeCount(Integer.parseInt(inputLineList[7]));
campusCrimeAnalysis.setAggravatedAssaultsCount(Integer.parseInt(inputLineList[8]));
campusCrimeAnalysisList.add(campusCrimeAnalysis);
}
scanner.close();
String[] inputList = getInputList();
int totalStudentCount = -1;// indicate total needed
if (inputList[0].trim().toLowerCase().equals("percent")) {
totalStudentCount = 0; // to indicate percent needed
}
int year = inputList[2].trim().toLowerCase().equals("all") ? 0 : Integer.parseInt(inputList[2].trim());// year=0
// represents
// all
int campusType = Integer.parseInt(inputList[3]);// 0-represents all
String state = inputList[4].trim().toLowerCase().equals("all") ? "" : inputList[4].trim();// ""
// represents
// all
// states
int totalCrime = 0;
String crimeType = inputList[1].trim().toLowerCase();
int maleStudentCount = 0;
int femaleStudentCount = 0;
// iterate over the file contents and calculate counts based on user
// inputs
for (int i = 0; i < campusCrimeAnalysisList.size(); i++) {
CampusCrimeAnalysis object = campusCrimeAnalysisList.get(i);
// condition for all or specific year & all or specific campus &&
// all or specific state ignoring -1 values
if ((year == 0 || year == object.getYear()) && (campusType == 0 || campusType == object.getCampusType())
&& (state.equals("") || state.equalsIgnoreCase(object.getState()))) {
if (totalStudentCount == 0) {
totalStudentCount += object.getMaleStudentCount() + object.getFemaleStudentCount();
}
maleStudentCount += object.getMaleStudentCount();
femaleStudentCount += object.getFemaleStudentCount();
switch (crimeType) {
case "murder": // murder count
totalCrime += (object.getMurderCount() != -1 ? object.getMurderCount() : 0);
break;
case "neghom":// negligent homicides count
totalCrime += (object.getNegligentHomicidesCount() != -1 ? object.getNegligentHomicidesCount() : 0);
break;
case "rape":// rape count
totalCrime += (object.getRapeCount() != -1 ? object.getRapeCount() : 0);
break;
case "agaslt":// aggrevated assualts count
totalCrime += (object.getAggravatedAssaultsCount() != -1 ? object.getAggravatedAssaultsCount() : 0);
break;
default: // all counts
totalCrime += (object.getMurderCount() != -1 ? object.getMurderCount() : 0);
totalCrime += (object.getNegligentHomicidesCount() != -1 ? object.getNegligentHomicidesCount() : 0);
totalCrime += (object.getRapeCount() != -1 ? object.getRapeCount() : 0);
totalCrime += (object.getAggravatedAssaultsCount() != -1 ? object.getAggravatedAssaultsCount() : 0);
break;
}
}
}
// display results
System.out.println("Male students count:" + maleStudentCount);
System.out.println("Female students count:" + femaleStudentCount);
if (totalStudentCount == -1) {// total count required scenario
System.out.println("Total crime = " + totalCrime + (totalCrime == 0 ? "/No data available" : ""));
} else {
System.out.println((totalCrime == 0 ? "Crime count = 0 or no data avalialbe"
: "1 crime per " + Math.round((double) totalStudentCount / totalCrime) + " students"));
}
}
private static String[] getInputList() {
String[] inputList = new String[5];
Scanner scanner = new Scanner(System.in);
System.out.println("Enter Total or Percent:");
inputList[0] = scanner.nextLine();
System.out.println("Enter crime type (" + crimeTypeList + ")");
inputList[1] = scanner.nextLine();
if (crimeTypeList.indexOf(inputList[1]) == -1) {
inputList[1] = "All";
}
System.out.println("Enter year (" + yearList + "):");
inputList[2] = scanner.nextLine();
if (yearList.indexOf(inputList[2]) == -1) {
inputList[2] = "All";
}
System.out.println(campusTypeList + " " + "Enter campus type Nummber:");
inputList[3] = scanner.nextLine();
try {
if (Integer.parseInt(inputList[3]) < 0 || Integer.parseInt(inputList[3]) > 9) {
inputList[3] = "0";
}
} catch (Exception e) {// occurs when non-numeric values entered.
inputList[3] = "0";
}
// printing state abbreviations 10/line
String[] stateAbbrList = stateAbbr.split(",");
for (int i = 0; i < stateAbbrList.length; i++) {
System.out.print(stateAbbrList[i]);
if ((i + 1) % 10 == 0 && i < stateAbbrList.length) {
System.out.println("");
} else {
System.out.print(" ");
}
}
inputList[4] = scanner.nextLine();
if (stateAbbr.indexOf(inputList[4]) == -1) {
inputList[4] = "All";
}
scanner.close();
return inputList;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
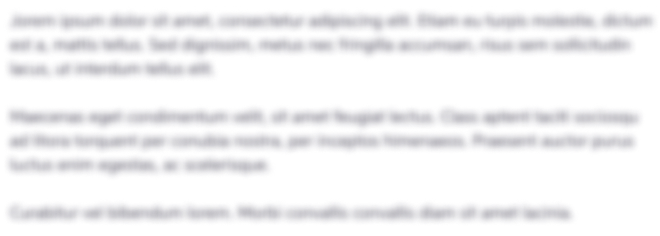
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started