Question
Coding in C++ Working on a project that deals with getting students informations and grades and putting them into a registrar, I need help with
Coding in C++
Working on a project that deals with getting students informations and grades and putting them into a registrar, I need help with the design on the project. Here are what I need to do with designing.
Come up with a design to solve the problem. Your design should include:
What are the member variables for the classes (Student and Registrar) and their types
A drawing of the relation between the objects of the two classes
Basically just name some variables that will go in each file and tell me how they relate between eachother classes. Dont have to write a full working code just an outline
Here are the files that I need to make members in
Student.h
class Student {
public:
Student(); // default constructor
Student(const string &cwid); // constructor with parameter
void addCourseGrade(const string &courseName, char grade); // add course name and grade to student's record
double getGPA(); // calculate and return GPA
void printTranscript(); // print transcript - see Student.cpp for the format
string getCWID(); // return the CWID of this student
private:
// any private member variables and methods go here
// TO BE COMPLETED
Student.cpp
Student::Student() {
// TO BE COMPLETED
}
Student::Student(const string &cwid) {
// TO BE COMPLETED
}
string Student::getCWID() {
// TO BE COMPLETED
}
void Student::addCourseGrade (const string &courseName, char grade) {
// TO BE COMPLETED
}
double Student::getGPA() {
// TO BE COMPLETED
}
// print transcript in this (sample) format:
// TRANSCRIPT FOR CWID=279750343
// CS 121 C
// CS 253 B
// CS 131 B
// GPA = 2.6667
void Student::printTranscript() {
// TO BE COMPLETED
}
Registrar.h
class Registrar {
public:
void readTextfile(string filename); // Load information from a text file with the given filename: THIS IS COMPLETE
void addLine(string courseName, string cwid, char grade); // process a line from the text file
Student& getStudent(string cwid) const; // return the Student object corresponding to a given CWID
// getStudent must throw an exception if cwid is invalid
// add constructors, destructors, assignment operators if needed
private:
// Add private member variables for your class along with any
// other variables required to implement the public member functions
// TO BE COMPLETED
Registrar.cpp
void Registrar::readTextfile(string filename) {
ifstream myfile(filename);
if (myfile.is_open()) {
cout << "Successfully opened file " << filename << endl;
string courseName;
string cwid;
char grade;
while (myfile >> courseName >> cwid >> grade) {
// cout << cwid << " " << grade << endl;
addLine(courseName, cwid, grade);
}
myfile.close();
}
else
throw invalid_argument("Could not open file " + filename);
}
// return Student object corresponding to a given CWID
// getStudent must throw an exception if cwid is invalid
Student& Registrar::getStudent(string cwid) const {
// TO BE COMPLETED
}
// process a line from the text file
void Registrar::addLine(string courseName, string cwid, char grade) {
// TO BE COMPLETED
Step by Step Solution
There are 3 Steps involved in it
Step: 1
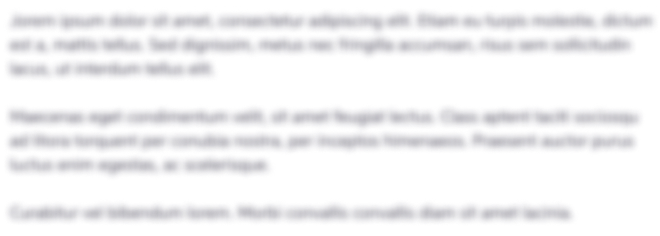
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started