Answered step by step
Verified Expert Solution
Question
1 Approved Answer
combine all these codes into a single one import java.util.Scanner; public class Lab7 { public static void main ( String[] args ) { Scanner scan
combine all these codes into a single one
import java.util.Scanner; public class Lab7 { public static void main(String[] args) { Scanner scan = new Scanner(System.in); String sFirstname, sLastname, sAsuID; double sGrade; String pName, pDesc, pFname; // Read some input data println("The student's first name?"); sFirstname = scan.nextLine(); println("The student's last name?"); sLastname = scan.nextLine(); println("The student's ASU ID?"); sAsuID = scan.nextLine(); println("Program name?"); pName = scan.nextLine(); println("Program desc?"); pDesc = scan.nextLine(); println("Program filename?"); pFname = scan.nextLine(); println("Program grade?"); sGrade = scan.nextDouble(); scan.nextLine(); println(""); scan.close(); // Create (define) a Student object "student1" Student student1 = new Student(sFirstname, sLastname, sAsuID); System.out.println("Making a student record ...[" + student1 + "]"); // Use the setGrade setter to set student1's grade student1.setGrade(sGrade); // Create (define) a Program object "program1" by "student1" Program program1 = new Program(pName, pDesc, pFname, student1); System.out.println("Making a program record ...[" + program1 + "]"); // Invoke makeReport to show the report of student1 makeReport(student1, program1); } private static void makeReport(Student student, Program program) { println(" ========== Program Submission Detail =========="); pprint("Student", student.getFullName()); pprint("ASU ID", student.getAsuid()); pprint("Grade", "" + student.getGrade()); println(""); pprint("Program", program.getProgramName()); pprint("Filename", program.getFileName()); pprint("Description", program.getDescription()); pprint("Datetime", program.getCreatedDate()); } private static void pprint(String key, String value) { println(String.format("%-12s: %-10s", key, value)); } private static void println(String s) { System.out.println(s); } }
public class Student { private String firstName, lastName, fullName, asuid; double grade; public Student(String firstName, String lastName, String asuid) { super(); this.firstName = firstName; this.lastName = lastName; this.fullName = firstName + " " + lastName; this.asuid = asuid; } public String toSTring() { return "Student: " + fullName + " ASU ID: " + asuid + " Grade: " + grade; } public String getFirstName() { return firstName; } public void setFirstName(String firstName) { this.firstName = firstName; } public String getLastName() { return lastName; } public void setLastName(String lastName) { this.lastName = lastName; } public String getFullName() { return fullName; } public void setFullName(String fullName) { this.fullName = fullName; } public String getAsuid() { return asuid; } public void setAsuid(String asuid) { this.asuid = asuid; } public double getGrade() { return grade; } public void setGrade(double grade) { this.grade = grade; } }
import java.time.LocalDateTime; public class Program { private String programName, description, createdDate, fileName; private Student author; public Program(String programName, String description, String fileName, Student author) { this.programName = programName; this.description = description; this.fileName = fileName; this.author = author; this.createdDate = LocalDateTime.now().toString(); } public String toString() { return String.format("Program: %s, Desc: %s, Filename: %s, Author: %s", programName, description, fileName, author.toString()); } public String getProgramName() { return programName; } public String getDescription() { return description; } public String getCreatedDate() { return createdDate; } public String getFileName() { return fileName; } public Student getAuthor() { return author; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
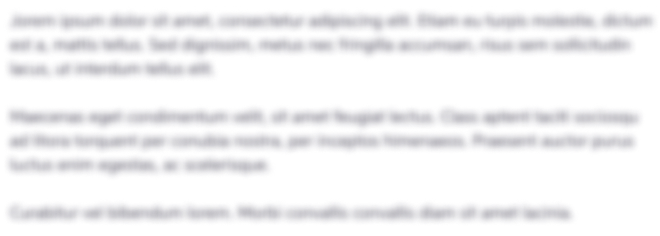
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started