Question
Combine the keypad and LCD codes in compliance to the following requirements: your last name to appear on LCD row 1, your last name to
Combine the keypad and LCD codes in compliance to the following requirements: your last name to appear on LCD row 1, your last name to appear on row 2, and on row 3 Key pressed: with ,0,1,2,A,3,4,5,6,B,7,8,9,C,*,0,#,D in row 3 column 14.
// Displaying messages on the 3 x 16 LCD // PORT C7 - PORT C4 output (LCD data bus); PORT C2 PORT C0 output (LCD control signals) // PORTB7 output (LCD Chip Select Bit active Low)
// Includes LCD initialization routine
#include
#include "derivative.h" /* derivative-specific definitions */
//globals - 4-bit initialization sequence for LCD - data: PC7,6,5,4 E: PC2 R/W~: PC1 RS: PC0
const unsigned char cInit_commands[20] = {0x30,0x30,0x30,0x20,0x20,0x90,0x10,0x50,0x70,0x80,0x50,0xE0,
0x60,0xA0,0x00,0xE0,0x00,0x10,0x00,0x60};
const unsigned char cMessage[3][17] =
{ {'D','a','v','e''},
{' ',' ','s','m','i','t','h' '},
{' ',' ',' ','T'';}};//data in Program Flash
const unsigned char cE = 0x04;
const unsigned char cRS = 0x01;
unsigned char cValues;
int p;
//function prototypes
void initLCD(void);
void initPorts(void);
void LCDputs(char*);
void LCDputch(char);
void cmdwrt(unsigned char);
void datawrt(char);
void position(int,int);
void delay1u(void);
void delay100u(void);
void delay2m(void);
void delays(int);
/************main************/
void main()
{
initPorts( ); // port initializations
initLCD( ); // LCD inititialization
cValues = 'C';
// datawrt('6'); // demonstrates single character write
// position(1,0);
// LCDputs(" "); // blank row 1
// position(2,0);
// LCDputs(" "); // blank row 2
// position(3,0);
// LCDputs(" "); // blank row 3
for(;;)
{
for (p=0;p<3;p++)
{
position(p+1,0);
LCDputs(cMessage[p]); //passes message address
}
delays(20000); //wait a long time
} /* forever loop */
}
/*********functions*********/
void initPorts( )
{
unsigned char cValue;
DDRB = 0x80; //LCD CSB active low
DDRC = 0xFF; // PC7-PC4 - 4-bit LCD data bus, PC2 - E, PC1 - R/W~, PC0 - RS: all outputs
cValue = PORTB;
PORTB = cValue | 0x00; // LCD CSB (PORT B7) enabled with a logic low
}
// sends initialization commands one-by-one
void initLCD( )
{
unsigned char i;
for (i=0;i<=19;i++)
{
cmdwrt(cInit_commands[i]);
}
}
// sends a control word to LCD bus
void cmdwrt(unsigned char cCtrlword)
{
PORTC = cCtrlword; // output command onto LCD data pins
PORTC = cCtrlword + cE; // generate enable pulse to latch it (xxxx x100)
delay1u( ); // hold it for 1us
PORTC = cCtrlword; // end enable pulse (xxxx x000)
delay2m(); // allow 2ms to latch command inside LCD
PORTC = 0x00;
delay2m(); // allow 2ms to latch command inside LCD
}
void position(int iRow_value, int iCol_value)
{
int iPos_h, iPos_l, iValue;
if(iRow_value == 1) iValue = (0x80+0x00);
if(iRow_value == 2) iValue = (0x80+0x10);
if(iRow_value == 3) iValue = (0x80+0x20);
iPos_h=((iValue + iCol_value) & 0xF0);
iPos_l=((iValue + iCol_value) & 0x0F) << 4;
cmdwrt(iPos_h);
cmdwrt(iPos_l);
}
//Sends a string of characters to the LCD;...
void LCDputs(char *sptr)
{
while(*sptr)
{ //...the string must end in a 0x00 (null character)
datawrt(*sptr); // sptr is a pointer to the characters in the string
++sptr;
}
}
//Sends a single character to the LCD;...
void LCDputch(char cValues)
{
datawrt(cValues); // single character value
}
// sends the character passed in by caller to LCD
void datawrt(char cAscii)
{
char cAscii_high, cAscii_low;
cAscii_high = (cAscii & 0xF0);
cAscii_low = (cAscii & 0x0F) << 4; // Shift left by 4 bits
PORTC = cAscii_high; // output ASCII character upper nibble onto LCD data pins
PORTC = cAscii_high + cRS + cE; // generate enable pulse to latch it (0xxx x101)
delay1u( ); // hold it for 1us
PORTC = cAscii_high + cRS; // end enable pulse (0xxx x001)
delay1u( ); // hold it for 1us
PORTC = cAscii_low; // output ASCII character lower nibble onto LCD data pins
PORTC = cAscii_low + cRS + cE; // generate enable pulse to latch it (0xxx x101)
delay1u( ); // hold it for 1us
PORTC = cAscii_low + cRS; // end enable pulse (0xxx x001)
delay100u( ); // allow 100us to latch data inside LCD
}
void delay1u( )
{
unsigned int i;
for(i=0;i<=0x0f;i++)
{ /* adjust condition field for delay time */
asm("nop");
}
}
void delay100u( )
{
unsigned int i,j;
for(i=0;i<=0x02;i++)
{ /* adjust condition field for delay time */
for(j=0;j<=0xff;j++)
{
asm("nop");
}
}
}
void delay2m( )
{
unsigned int i,j;
for (i=0;i<=0x20;i++)
{ /* adjust condition field for delay time */
for (j=0;j<=0xff;j++)
{
asm("nop");
}
}
}
void delays(int k )
{
unsigned int i,j;
for (i=0;i<=k;i++)
{ /* adjust condition field for delay time */
for (j=0;j<=0xff;j++)
{
asm("nop");
}
}
} /********* end of file ***********************/
THEN add this set of code to it.
// 4x4 keypad driver (uses software polling) // PORT A7 - PORT A4 input from keypad rows; PORT A3 PORT A0 output to keypad columns // Activates one column at a time and read rows
// Identifies closed key and displays the results on the PORT T7 PORT T4 LEDs #include
#include "derivative.h" /* derivative-specific definitions */
#include
{
0x00, // All Columns logic High
0x01, // Only Column 1 logic High
0x02, // Only Column 2 logic High
0x04, // Only Column 3 logic High
0x08 // Only Column 4 logic High
}; // End of the row array
const unsigned char cResult[17] = // One dimension array for results of 0 thru F- Logic low turns LED on
{
0xF0, // no key pressed or more than one key pressed all LEDs off
0xE0, // Row 1, Col 1 key pressed LED4 off LED3 off LED2 off LED1 on
0xD0, // Row 1, Col 2 key pressed LED4 off LED3 off LED2 on LED1 off
0xC0, // Row 1, Col 3 key pressed LED4 off LED3 off LED2 on LED1 on
0xB0, // Row 1, Col 4 key pressed LED4 off LED3 on LED2 off LED1 off
0xA0, // Row 2, Col 1 key pressed LED4 off LED3 on LED2 off LED1 on
0x90, // Row 2, Col 2 key pressed LED4 off LED3 on LED2 on LED1 off
0x80, // Row 2, Col 3 key pressed LED4 off LED3 on LED2 on LED1 on
0x70, // Row 2, Col 4 key pressed LED4 on LED3 off LED2 off LED1 off
0x60, // Row 3, Col 1 key pressed LED4 on LED3 off LED2 off LED1 on
0x50, // Row 3, Col 2 key pressed LED4 on LED3 off LED2 on LED1 off
0x40, // Row 3, Col 3 key pressed LED4 on LED3 off LED2 on LED1 on
0x30, // Row 3, Col 4 key pressed LED4 on LED3 on LED2 off LED1 off
0x20, // Row 4, Col 1 key pressed LED4 on LED3 on LED2 off LED1 on
0x10, // Row 4, Col 2 key pressed LED4 on LED3 on LED2 on LED1 off
0x00, // Row 4, Col 3 key pressed LED4 off LED3 on LED2 off LED1 on
0xF0, // Row 2, Col 1 key pressed LED4 off LED3 off LED2 off LED1 off
}; // End of the row array
unsigned char cRow[5]; // One dimension array pointing to the active Row
unsigned char cValue; // Variable used in reading PORT A
unsigned int i, j, k, m, n; // Counter variables
/************main************/ void main(void)
{ DDRA = 0x0F; // Upper nibble Rows: input, Lower nibble Columns: output
//PUCR = 0x01; // This command would eEnable Port A pull up resistors -
DDRT = 0xF0; // Upper nibble LEDs: output logic low turns LED on
while(1) // Infinite while loop
{
for(i = 0; i < 5; i++)
{
cRow[i] = 0xF0; // Initialize all row results to open condition
}
// Key press detector loop
i = 0;
cValue = PORTA & 0xF0; // Read current value on upper nibble
PORTA = cValue | cCol[i]; // Output logic High to all columns
while ( cRow[i] == 0xF0 ) // Stay in loop until key pressed
{
cRow[i] = PORTA & 0xF0; // Use mask to focus on upper nibble
}
// Key press identification loop
for(i = 1; i < 5; i++)
{
cValue = PORTA & 0xF0; // Read current value on upper nibble
PORTA = cValue | cCol[i]; // Output logic High to Column i where | is or operator
for (j = 0; j <= 200; j++) // Delay loop allows time for the Column output to reach keypad {
asm("nop"); // No operation assembly instruction } cRow[i] = PORTA & 0xF0; // Read all row results for Column i
}
// Key press assignment loop
n = 0; // Set n value to 0 used as the identifier for the Row and Column
for(i = 1; i < 5; i++)
{
switch (cRow[i])
{
case 0x00: // No key pressed for Column i
n += 0;
break;
case 0x10: // Column i Row 1 key pressed
n += 0 + i;
break;
case 0x20: // Column i Row 2 key pressed
n += 4 + i;
break;
case 0x40: // Column i Row 3 key pressed
n += 8 + i;
break;
case 0x80: // Column i + 1 Row 4 key pressed
n += 12 + i;
break;
default: // more than one key pressed
n += 17;
break;
} // end of switch case
} // end for loop
if (n >= 17) n = 0; // More than one key pressed
// Display result on LEDs
PTT = cResult[n];
m = 0;
// This routine is provided only because there are only 4 LEDs to display the results
for (i = 0; i <= 10; i++) // LED delay routine
{
for (j = 0; j <= 300; j++)
{
for (k = 0; k <= 255; k++)
{
asm (nop);
} // end of k count loop
} // end of j count loop
if (n == 16) // Row 4 Col 4 will be indicated by flashing all 4 LEDs
{
if (m == 0)
{
m++;
PTT = 0x00; // Row 4 Col 4 all 4 LEDs turned on
}
else
{
m = 0;
PTT = cResult[n]; // Row 4 Col 4 all 4 LEDs turned off
}
} // End of if (n == 16)
} // end of i count loop
} // end of Infinite while loop
} // end of main function
Step by Step Solution
There are 3 Steps involved in it
Step: 1
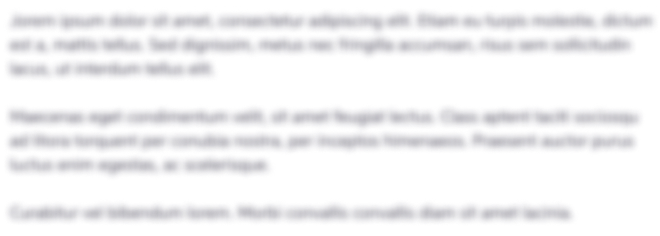
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started