Answered step by step
Verified Expert Solution
Question
1 Approved Answer
COMP 1020 Winter 2021 I need help with the first part of this homework, please as soon as possible; This is the testing code; public
COMP 1020 Winter 2021
I need help with the first part of this homework, please as soon as possible;
This is the testing code;
public class TestA2Phase1 { public static void main( String[] args ) { int[][] b0, b1, b2, b3; GameOfLife g; String[] state = { "dead", "live" }; // Test with a hard-coded starting board (b0) b0 = new int[][]{ { 0, 0, 1 }, { 1, 1, 1 }, { 0, 1, 0 } }; b1 = new int[][]{ { 0, 0, 1 }, { 1, 0, 1 }, { 1, 1, 1 } }; b2 = new int[][]{ { 0, 1, 0 }, { 1, 0, 1 }, { 1, 0, 1 } }; b3 = new int[][]{ { 0, 1, 0 }, { 1, 0, 1 }, { 0, 0, 0 } }; System.out.println( "Phase 1 Testing:" ); g = new GameOfLife( b0, 10 ); System.out.println( " Testing the newState method:" ); for ( int i = 1; i Phase 1: We are going to implement the Game of Life using two-dimensional arrays of ints (called "boards"). Each int in a board is a cell if the cell is alive, then the corresponding int is 1, and if the cell is dead, then the corresponding int is 0. At each time tick, all the cells evolve simultaneously -- we get a new board. We are going to store all the different boards, starting at time tick 0, in an array of boards we're really going to be working with a three dimensional array of ints. The extra dimension in the array represents time. In this phase, implement a simple class CameOfLife, which should have: . Two instance variables: the three dimensional array of ints representing the boards over time, and another variable (an int) that contains the current time tick - that is, the variable tells you how many times the starting board at time tick 0) has been evolved so far. A class (static) method cloneOneBoard that accepts a board (a 2D array of ints) as a parameter and returns a clone (deep copy) of it. Use the following header: public static int[] clone OneBoard( int [] [] board) A constructor that accepts a starting board (a 2D array of ints) and a number, n, of time ticks (an int). The constructor should initialize the 3D array instance variable so that it can hold boards for na time ticks (including the starting board at time tick 0). (You should initialize the instance variable but only allocate the time dimension (the first dimension). We will add the boards for various time ticks later.) The current time tick instance variable should be set to 0. Finally, the constructor should also create a clone of the starting board and make the clone be the board at time tick 0 in the 3D array instance variable. Two accessor methods: - getCurrentTick, which returns the current time tick - that is, it returns the time tick of the most recently created board stored in the 3D array instance variable. - getMaxTick, which returns the time tick that would be associated with the last position in the 3D array instance variable that is, if we filled up the 3D array instance variable completely, what would be the time tick associated with the last board created? (Remember: Time tick 0 is the time tick of the starting board.) . An instance method newState that accepts a cell's current state and the number of live neighbours it currently has. The method should return the new state of the cell after the next time tick, using the rules above. Use the following header: public int newState( int cellCurrentState, int numLiveNeighbours ) An instance method evolveOnce that first checks if the current time tick is less that the length of the time dimension (the first dimension) of the array of boards this check ensures that we have room for one more board in the array of boards. If there is room for one more board in the array, then it creates a new board (the same size as the starting board), puts it into the 3D array as the board at the next time tick, transitions all cells (puts their new states into the new board), and increments the current time tick to the new time tick. (If there is no room for one more board in the array of boards, then this method should do nothing.) Use the following header: public void evolveOnce() 3 The code to put the new states of the cells into the new boards is too long to all be in one method. So evolveOnce should call smaller helper methods to do parts of the work. Here are some helper methods that you must write for evolveOnce to call. Each helper method is passed references to the current board and the new board, and puts the new states of only some of the cells into the new board: evolve Corners puts the new states of just the corner cells into the new board, based on states in the current board - that is, this method evolves cells that have exactly 3 neighbours. Use the following header: public void evolve Corners ( int ( current Board, int[newBoard) - evolveEdges puts the new states of just cells on the edges of the board except for the corner cells) into the new board, based on states in the current board that is, this method evolves cells that have exactly 5 neighbours. Use the following header: public void evolveEdges( int [] [] currentBoard, int[] [] newBoard ) - evolveMiddle puts the new states of just cells in the middle of the board into the new board, based on states in the current board that is, this method evolves cells that have exactly 8 neighbours. Use the following header: public void evolveMiddle( int [] [] current Board, int [] newBoard ) Warning: For each of the helper methods, make sure that you look at states in current Board (not in newBoard) when computing the number of live neighbours a cell has. A toString method that returns a String representation of the most recent board (the current board). Use the current time tick to figure out which board in the array is the most recent board. (Every row should start with 3 blanks and there should be a newline character " " between each pair of rows.) . An equals method that accepts a board as a parameter. The method should return true if the current board in the array of boards and the parameter board are the same size and the cells are the same; otherwise, the method should return false. Note: You should add more helper methods as needed to make the required methods short. You can test your class using the supplied test program TestA2Phase1.java. You should get the output below. Phase 1 Testing: Testing the newState method: A live cell with 1 live neighbours will be dead A dead cell with 1 live neighbours will be dead A live cell with 2 live neighbours will be live A dead cell with 2 live neighbours will be dead A live cell with 3 live neighbours will be live A dead cell with 3 live neighbours will be live A live cell with 4 live neighbours will be dead A dead cell with 4 live neighbours will be dead Now trying multiple evolutions of the whole board: Maximum time tick allowed: 9 At time tick 0: Current time tick = 0 4 001 111 010 At time tick 1: Current time tick = 1 001 101 111 At time tick 2: Current time tick = 2 010 101 101 At time tick 3: Current time tick = 3 010 101 000 Current time tick at very end: 3 Phase 1: We are going to implement the Game of Life using two-dimensional arrays of ints (called "boards"). Each int in a board is a cell if the cell is alive, then the corresponding int is 1, and if the cell is dead, then the corresponding int is 0. At each time tick, all the cells evolve simultaneously -- we get a new board. We are going to store all the different boards, starting at time tick 0, in an array of boards we're really going to be working with a three dimensional array of ints. The extra dimension in the array represents time. In this phase, implement a simple class CameOfLife, which should have: . Two instance variables: the three dimensional array of ints representing the boards over time, and another variable (an int) that contains the current time tick - that is, the variable tells you how many times the starting board at time tick 0) has been evolved so far. A class (static) method cloneOneBoard that accepts a board (a 2D array of ints) as a parameter and returns a clone (deep copy) of it. Use the following header: public static int[] clone OneBoard( int [] [] board) A constructor that accepts a starting board (a 2D array of ints) and a number, n, of time ticks (an int). The constructor should initialize the 3D array instance variable so that it can hold boards for na time ticks (including the starting board at time tick 0). (You should initialize the instance variable but only allocate the time dimension (the first dimension). We will add the boards for various time ticks later.) The current time tick instance variable should be set to 0. Finally, the constructor should also create a clone of the starting board and make the clone be the board at time tick 0 in the 3D array instance variable. Two accessor methods: - getCurrentTick, which returns the current time tick - that is, it returns the time tick of the most recently created board stored in the 3D array instance variable. - getMaxTick, which returns the time tick that would be associated with the last position in the 3D array instance variable that is, if we filled up the 3D array instance variable completely, what would be the time tick associated with the last board created? (Remember: Time tick 0 is the time tick of the starting board.) . An instance method newState that accepts a cell's current state and the number of live neighbours it currently has. The method should return the new state of the cell after the next time tick, using the rules above. Use the following header: public int newState( int cellCurrentState, int numLiveNeighbours ) An instance method evolveOnce that first checks if the current time tick is less that the length of the time dimension (the first dimension) of the array of boards this check ensures that we have room for one more board in the array of boards. If there is room for one more board in the array, then it creates a new board (the same size as the starting board), puts it into the 3D array as the board at the next time tick, transitions all cells (puts their new states into the new board), and increments the current time tick to the new time tick. (If there is no room for one more board in the array of boards, then this method should do nothing.) Use the following header: public void evolveOnce() 3 The code to put the new states of the cells into the new boards is too long to all be in one method. So evolveOnce should call smaller helper methods to do parts of the work. Here are some helper methods that you must write for evolveOnce to call. Each helper method is passed references to the current board and the new board, and puts the new states of only some of the cells into the new board: evolve Corners puts the new states of just the corner cells into the new board, based on states in the current board - that is, this method evolves cells that have exactly 3 neighbours. Use the following header: public void evolve Corners ( int ( current Board, int[newBoard) - evolveEdges puts the new states of just cells on the edges of the board except for the corner cells) into the new board, based on states in the current board that is, this method evolves cells that have exactly 5 neighbours. Use the following header: public void evolveEdges( int [] [] currentBoard, int[] [] newBoard ) - evolveMiddle puts the new states of just cells in the middle of the board into the new board, based on states in the current board that is, this method evolves cells that have exactly 8 neighbours. Use the following header: public void evolveMiddle( int [] [] current Board, int [] newBoard ) Warning: For each of the helper methods, make sure that you look at states in current Board (not in newBoard) when computing the number of live neighbours a cell has. A toString method that returns a String representation of the most recent board (the current board). Use the current time tick to figure out which board in the array is the most recent board. (Every row should start with 3 blanks and there should be a newline character " " between each pair of rows.) . An equals method that accepts a board as a parameter. The method should return true if the current board in the array of boards and the parameter board are the same size and the cells are the same; otherwise, the method should return false. Note: You should add more helper methods as needed to make the required methods short. You can test your class using the supplied test program TestA2Phase1.java. You should get the output below. Phase 1 Testing: Testing the newState method: A live cell with 1 live neighbours will be dead A dead cell with 1 live neighbours will be dead A live cell with 2 live neighbours will be live A dead cell with 2 live neighbours will be dead A live cell with 3 live neighbours will be live A dead cell with 3 live neighbours will be live A live cell with 4 live neighbours will be dead A dead cell with 4 live neighbours will be dead Now trying multiple evolutions of the whole board: Maximum time tick allowed: 9 At time tick 0: Current time tick = 0 4 001 111 010 At time tick 1: Current time tick = 1 001 101 111 At time tick 2: Current time tick = 2 010 101 101 At time tick 3: Current time tick = 3 010 101 000 Current time tick at very end: 3
Step by Step Solution
There are 3 Steps involved in it
Step: 1
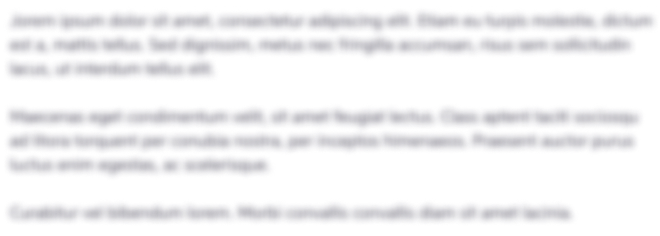
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started