Question
complete in C++ code Lists Many programming languages have some kind list data structure, which allows for insertion and deletion of elements, essentially an array
complete in C++ code
Lists
Many programming languages have some kind list data structure, which allows for insertion and deletion of elements, essentially an array that changes size. C++ has vectors, Java has the ArrayList, Python has lists (which is what they're called generically anyway). But how do they really work? All of these actually use arrays, which naturally have immutable lengths.
Your Task
Your task is to write a function, insert, which inserts a new item into an existing array, then returns the modified array. Since you can not really do this, you will have create a new array which has all the elements of the original array, plus the new item in its proper place, and return that new array instead. This is how lists actually work. I won't make you write the code for deleting items; after you do this, you'll understand how it works anyway.
You may notice that the return type of insert is a pointer to an integer. This is fine, since an array is just a pointer to the first item in the array.
Grading
2 points for each:
Adds the new item to the array
Inserts the new item in the correct place
Does not remove any items from the array
Does not cause any memory leaks, segmentation faults, etc
-
Comments explains how it works
The starter code is listed bellow
#include
/* * insert takes an array of ints and inserts an item * at a given index, shifting all elements to the right * to make room for it as needed. There must be a * length number of integers in the array. It then * returns the new array with item inserted correctly. * * If index >= length, item is appended to the array * If index < 0, insertion point is calculated counting * from the end of the array. -1 = length-1, etc. */ int * insert(int arr[], int length, int item, int index); /* * print takes an array of ints and prints it * with spaces between each item. There must be * a length number of integers. */ void print(int arr[], int length); int main() { //These next two lines make an array and put numbers in it int * numbers = new (std::nothrow) int [4]; for(int i = 0; i < 4; i++) { numbers[i] = (i * 2) + 2; } //Prints 2 4 6 8 print(numbers, 4); numbers = insert(numbers, 4, 100, 2); //Prints 2 4 100 6 8 print(numbers, 5); //Feel free to add your own test cases here, // to make sure your function works //Memory cleanup delete [] numbers; return 0; } int * insert(int arr[], int length, int item, int index) { //YOUR CODE GOES HERE return arr; //Delete this line } void print(int arr[], int length) { for(int i = 0; i < length; i++) { std::cout << arr[i] << " "; } std::cout << std::endl; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
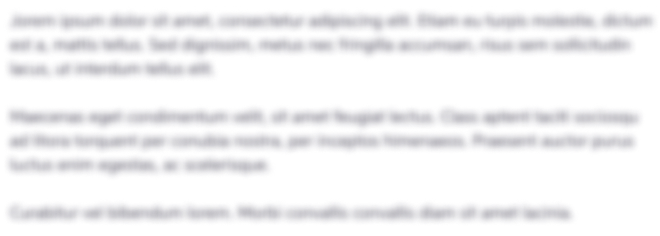
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started