Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Complete in C + + . Instructions: MobileElement implementation with your balance ( ) code. Your task is to complete a program that reads a
Complete in C Instructions: MobileElement implementation with your balance code.
Your task is to complete a program that reads a description of a mobile and computes, for each bar, the position along that bar where the string should be tied so that, when the bar is hanging from that string, it will be balanced. This position will be expressed as a fraction between and of the length of the bar from the left end to the place where the string is tied.
The main program will be named runMobiles and will take its input from a file named in a commandline parameter or if no parameter is provided, from standard in Eg it can be run on an input in test.in like this:
runMobiles test.in
or like this
runMobiles test.in
Your task is to write the function balance which computes the connection points for the bar it is given as a parameter and for all bars hanging from it directly or indirectly. Given a mobile with N bars, it should accomplish this task in ON time.
Attached is mobile.cpp:
#include "mobile.h
#include
#include
#include
using namespace std;
Create a weight element
MobileElement::MobileElement double w
: isABarfalse weightw id leftnullptr rightnullptr balancePoint
Create a bar element
MobileElement::MobileElement string idstr, Mobile lft Mobile rght
: isABartrue weight
ididstr leftlft rightrght balancePoint
MobileElement::~MobileElement
delete left;
delete right;
Read and write mobiles
Mobile MobileElement::readTree istream& in
char c;
in c;
if c
This is the start of a new bar description
string id;
in id;
Mobile left readTreein;
Mobile right readTreein;
Mobile newBar new MobileElement id left, right;
in c;
assert c ; Check for syntax error
return newBar;
else
A decorative weight
double w;
inputbackc;
in w;
return new MobileElementw;
std::istream& operatorstd::istream& in Mobile& m
m MobileElement::readTree in;
return in;
void MobileElement::printTree ostream& out, int level const
out stringlevel;
if isABar
out id
;
leftprintTreeout level;
out
;
rightprintTreeout level;
out ;
else
out fixed setprecision weight;
void MobileElement::listBars ostream& out const
if isABar
out id : fixed setprecision balancePoint endl;;
leftlistBarsout;
rightlistBarsout;
else
Do nothing for decorative elements
std::ostream& operatorstd::ostream& out, Mobile m
if m
mprintTree out;
out
;
mlistBarsout;
return out;
double MobileElement::balance
Insert your code here, replacing the return statement below
return ;
Attached is mobile.h:
#ifndef MOBILEH
#define MOBILEH
#include
#include
#include
class MobileElement;
typedef MobileElement Mobile;
class MobileElement
bool isABar;
For decorative objects only, ignored for bars:
double weight;
For bars only, ignored for decorative objects
std::string id;
Mobile left;
Mobile right;
double balancePoint;
public:
Create a weight element
MobileElement double weight;
Create a bar element
MobileElement std::string idstr, Mobile left, Mobile right;
~MobileElement;
When invoked on a bar, compute and set the balancePoint
for that bar and for all bars that hang from it directly
or indirectly.
@return the total weight of all elements hanging from this bar
directly or indirectly
double balance ;
double getBalancePoint const return balancePoint;
private:
static Mobile readTree std::istream& in;
void printTree std::ostream& out, int level const;
void listBars std::ostream& out const;
friend std::istream& operatorstd::istream& in Mobile& m;
friend std::ostream& operatorstd::ostream& out, Mobile m;
;
Read and write mobiles
std::istream& operatorstd::istream& in Mobile& m;
std::ostream& operatorstd::ostream& out, Mobile m;
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
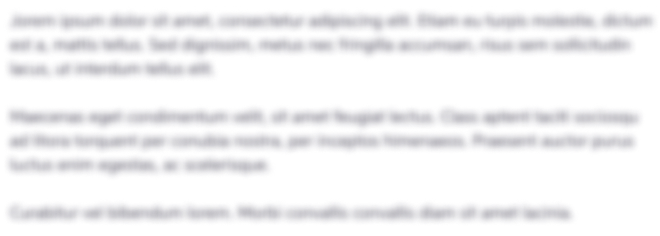
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started