Question
//Complete the C++ function to find the length of a linked list. #include using namespace std; class Node { public: Node *next; int data; Node(int
//Complete the C++ function to find the length of a linked list.
#include
using namespace std;
class Node { public: Node *next; int data;
Node(int d) { data = d; next = nullptr; } };
class LinkedList { public: Node *head; Node *tail; LinkedList() { head = nullptr; tail = nullptr; }
void push(int data) { Node *newNode = new Node(data); if (!head) { head = newNode; tail = newNode; } else { tail->next = newNode; tail = newNode; } }
int getCount() { // Complete this Function }
void deleteList() { Node *curr = head; Node *next = nullptr; while (curr) { next = curr->next; delete curr; curr = next; } head = nullptr; }
};
int main() { LinkedList l1; l1.push(8); l1.push(9); l1.push(3); l1.push(14); l1.push(5);
LinkedList l2; l2.push(8); l2.push(18);
LinkedList l3;
LinkedList l4; l4.push(42);
LinkedList l5; l5.push(8); l5.push(1); l5.push(12);
cout << l1.getCount() << endl; cout << "Expected: 5" << endl;
cout << l2.getCount() << endl; cout << "Expected: 2" << endl;
cout << l3.getCount() << endl; cout << "Expected: 0" << endl;
cout << l4.getCount() << endl; cout << "Expected: 1" << endl;
cout << l5.getCount() << endl; cout << "Expected: 3" << endl;
l1.deleteList(); l2.deleteList(); l3.deleteList(); l4.deleteList(); l5.deleteList();
return 1; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
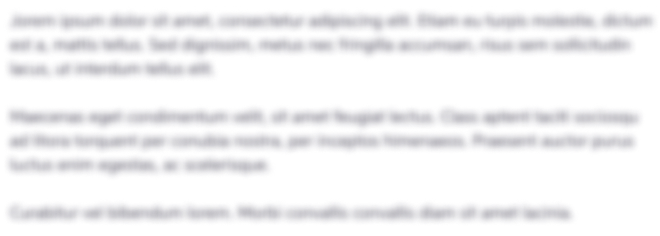
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started