Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Complete the code for a GUI application that allows the user to purchase tickets for a movie. There are 4 movies to choose from. A
Complete the code for a GUI application that allows the user to purchase tickets for a movie. There are 4 movies to choose from. A ticket to a 2D movie costs $12, while a 3D ticket costs $17.
import javafx.application.*; import javafx.stage.*; import javafx.scene.*; import javafx.scene.layout.*; import javafx.scene.control.*; import javafx.geometry.*; public class MessageBox { public static void show(String message, String title) { Stage stage = new Stage(); stage.initModality(Modality.APPLICATION_MODAL); stage.setTitle(title); stage.setMinWidth(250); Label lbl = new Label(); lbl.setText(message); Button btnOk = new Button(); btnOk.setText("OK"); btnOk.setOnAction(e -> stage.close()); VBox pane = new VBox(20); pane.getChildren().addAll(lbl, btnOk); pane.setAlignment(Pos.CENTER); Scene scene = new Scene(pane, 300, 200); stage.setScene(scene); stage.showAndWait(); } }
import javafx.application.Application; import javafx.event.ActionEvent; import javafx.scene.layout.HBox; import javafx.scene.layout.VBox; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.control.Label; import javafx.scene.control.ChoiceBox; import javafx.scene.control.TextField; import javafx.stage.Stage; import javafx.geometry.*; public class Lab11 extends Application { // Declare the class properties private Stage stage; private VBox sceneBox; private HBox movieListingBox; private HBox numTicketsBox; private HBox buttonBox; private Label movieListingLabel; private Label numTicketsLabel; private ChoiceBoxThis is what the application looks like when it loads PURCHASE MOVIE TICKETS Select a movie: Number of tickets: PURCHASE CANCEL The user can select a movie from the dropdown list and enter a value for the number of tickets in the text box PURCHASE MOVIE TICKETS Select a movie: Batman V Superman (3D) Number of tickets:4 PURCHASE CANCELmovieChoiceBox; private TextField numTicketsTextField; private Button purchaseButton; private Button cancelButton; // Use these constants when calculating total cost private static double TICKET_PRICE_3D = 17.0; private static double TICKET_PRICE_2D = 12.0; @Override public void start(Stage primaryStage) { stage = primaryStage; stage.setTitle("PURCHASE MOVIE TICKETS"); // TODO: Initialize movieListingLabel and set its minimum width to 75 // TODO: Initialize movieChoiceBox and add the following movie titles to it: // Batman V Superman (3D) // Kung Fu Panda 3 (2D) // My Big Fat Greek Wedding 2 (3D) // Rush Hour (2D) // Place movieListingLabel and movieChoiceBox into the movieListingBox container movieListingBox = new HBox(20, movieListingLabel, movieChoiceBox); // TODO: Initialize numTicketsLabel and set its minimum width to 75 // TODO: Initialize numTicketsTextField and set its preferred column width to 4 // Place numTicketsLabel and numTicketsTextField into the numTicketsBox container numTicketsBox = new HBox(20, numTicketsLabel, numTicketsTextField); // TODO: Initialize purchaseButton and cancelButton // Set the actions for the buttons cancelButton.setOnAction(e -> close()); purchaseButton.setOnAction(e -> displayResults()); // TODO: Place the two buttons into buttonBox with 60 pixels between them // Center the buttons in buttonBox buttonBox.setAlignment(Pos.CENTER); // Add the sub-containers to the sceneBox and put some padding around each one sceneBox = new VBox(60, movieListingBox, numTicketsBox, buttonBox); sceneBox.setPadding(new Insets(30,20,30,20)); // Create a new Scene object with the sceneBox in it Scene scene = new Scene(sceneBox, 440, 280); // TODO: Set scene to be the scene for stage and show the stage } // This method will close and application public void close() { stage.close(); } // TODO: Code the displayResults method. This method is called when the user clicks on the // purchase button. // // Call MessageBox.show to generate dialog boxes. // // Here is some pseudo code for this method: // // Initialize numTickets (int) to 0 and totalCost (double) to 0.0 // Set movieSelection to the value that is currently selected in movieChoiceBox // // If movieSelection is null: // Show an error dialog box that says, "Please select a movie!" // Else: // Try: // Set numTickets to be the parsed value of numTicketsTextField // If movieSelection ends with "(2D)": // Set totalCost = numTickets * TICKET_PRICE_2D // Else: // Set totalCost = numTickets * TICKET_PRICE_3D // Display a dialog box that shows the total cost // Catch (NumberFormatException e): // Display an error dialog box that says, "Please enter a number for the number of tickets!" // public void displayResults() { } // Start the application in the main method public static void main(String[] args) { Application.launch(args); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
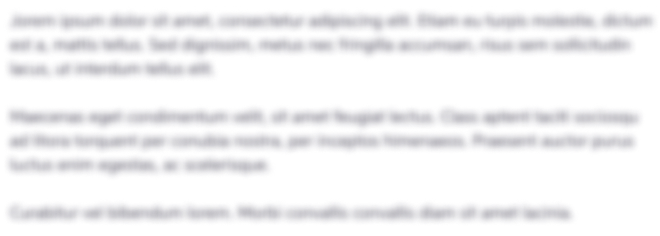
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started